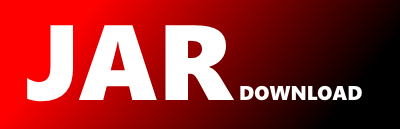
com.alphasystem.docx4j.builder.wml.TblPrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of open-xml-builder Show documentation
Show all versions of open-xml-builder Show documentation
Alpha system commons library
package com.alphasystem.docx4j.builder.wml;
import java.math.BigInteger;
import org.docx4j.wml.BooleanDefaultTrue;
import org.docx4j.wml.CTShd;
import org.docx4j.wml.CTString;
import org.docx4j.wml.CTTblCellMar;
import org.docx4j.wml.CTTblLayoutType;
import org.docx4j.wml.CTTblLook;
import org.docx4j.wml.CTTblOverlap;
import org.docx4j.wml.CTTblPPr;
import org.docx4j.wml.CTTblPrChange;
import org.docx4j.wml.Jc;
import org.docx4j.wml.JcEnumeration;
import org.docx4j.wml.STTblLayoutType;
import org.docx4j.wml.STTblOverlap;
import org.docx4j.wml.TblBorders;
import org.docx4j.wml.TblPr;
import org.docx4j.wml.TblWidth;
/**
* Fluent API builder for org.docx4j.wml.TblPr
.
*
*/
public class TblPrBuilder
extends com.alphasystem.docx4j.builder.OpenXmlBuilder
{
private TblPrBuilder.TblStyleBuilder tblStyleBuilder;
private TblPrBuilder.TblStyleRowBandSizeBuilder tblStyleRowBandSizeBuilder;
private TblPrBuilder.TblStyleColBandSizeBuilder tblStyleColBandSizeBuilder;
/**
* Initialize the underlying object.
*
*/
public TblPrBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public TblPrBuilder(TblPr object) {
super(object);
tblStyleBuilder = new TblPrBuilder.TblStyleBuilder(this.object.getTblStyle());
tblStyleRowBandSizeBuilder = new TblPrBuilder.TblStyleRowBandSizeBuilder(this.object.getTblStyleRowBandSize());
tblStyleColBandSizeBuilder = new TblPrBuilder.TblStyleColBandSizeBuilder(this.object.getTblStyleColBandSize());
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public TblPrBuilder(TblPr src, TblPr target) {
this(target);
if (src!= null) {
CTTblPrChange tblPrChange = src.getTblPrChange();
if (tblPrChange!= null) {
tblPrChange = new CTTblPrChangeBuilder(tblPrChange, object.getTblPrChange()).getObject();
}
org.docx4j.wml.CTTblPrBase.TblStyle tblStyle = src.getTblStyle();
if (tblStyle!= null) {
tblStyle = new TblPrBuilder.TblStyleBuilder(tblStyle, object.getTblStyle()).getObject();
}
CTTblPPr tblpPr = src.getTblpPr();
if (tblpPr!= null) {
tblpPr = new CTTblPPrBuilder(tblpPr, object.getTblpPr()).getObject();
}
CTTblOverlap tblOverlap = src.getTblOverlap();
if (tblOverlap!= null) {
tblOverlap = new com.alphasystem.docx4j.builder.wml.CTTblOverlapBuilder(tblOverlap, object.getTblOverlap()).getObject();
}
org.docx4j.wml.CTTblPrBase.TblStyleRowBandSize tblStyleRowBandSize = src.getTblStyleRowBandSize();
if (tblStyleRowBandSize!= null) {
tblStyleRowBandSize = new TblPrBuilder.TblStyleRowBandSizeBuilder(tblStyleRowBandSize, object.getTblStyleRowBandSize()).getObject();
}
org.docx4j.wml.CTTblPrBase.TblStyleColBandSize tblStyleColBandSize = src.getTblStyleColBandSize();
if (tblStyleColBandSize!= null) {
tblStyleColBandSize = new TblPrBuilder.TblStyleColBandSizeBuilder(tblStyleColBandSize, object.getTblStyleColBandSize()).getObject();
}
TblWidth tblW = src.getTblW();
if (tblW!= null) {
tblW = new com.alphasystem.docx4j.builder.wml.TblWidthBuilder(tblW, object.getTblW()).getObject();
}
Jc jc = src.getJc();
if (jc!= null) {
jc = new com.alphasystem.docx4j.builder.wml.JcBuilder(jc, object.getJc()).getObject();
}
TblWidth tblCellSpacing = src.getTblCellSpacing();
if (tblCellSpacing!= null) {
tblCellSpacing = new com.alphasystem.docx4j.builder.wml.TblWidthBuilder(tblCellSpacing, object.getTblCellSpacing()).getObject();
}
TblWidth tblInd = src.getTblInd();
if (tblInd!= null) {
tblInd = new com.alphasystem.docx4j.builder.wml.TblWidthBuilder(tblInd, object.getTblInd()).getObject();
}
TblBorders tblBorders = src.getTblBorders();
if (tblBorders!= null) {
tblBorders = new TblBordersBuilder(tblBorders, object.getTblBorders()).getObject();
}
CTShd shd = src.getShd();
if (shd!= null) {
shd = new CTShdBuilder(shd, object.getShd()).getObject();
}
CTTblLayoutType tblLayout = src.getTblLayout();
if (tblLayout!= null) {
tblLayout = new com.alphasystem.docx4j.builder.wml.CTTblLayoutTypeBuilder(tblLayout, object.getTblLayout()).getObject();
}
CTTblCellMar tblCellMar = src.getTblCellMar();
if (tblCellMar!= null) {
tblCellMar = new CTTblCellMarBuilder(tblCellMar, object.getTblCellMar()).getObject();
}
CTTblLook tblLook = src.getTblLook();
if (tblLook!= null) {
tblLook = new CTTblLookBuilder(tblLook, object.getTblLook()).getObject();
}
CTString tblCaption = src.getTblCaption();
if (tblCaption!= null) {
tblCaption = new com.alphasystem.docx4j.builder.wml.CTStringBuilder(tblCaption, object.getTblCaption()).getObject();
}
withTblPrChange(tblPrChange).withTblStyle(tblStyle).withTblpPr(tblpPr).withTblOverlap(tblOverlap).withBidiVisual(WmlBuilderFactory.cloneBooleanDefaultTrue(src.getBidiVisual())).withTblStyleRowBandSize(tblStyleRowBandSize).withTblStyleColBandSize(tblStyleColBandSize).withTblW(tblW).withJc(jc).withTblCellSpacing(tblCellSpacing).withTblInd(tblInd).withTblBorders(tblBorders).withShd(shd).withTblLayout(tblLayout).withTblCellMar(tblCellMar).withTblLook(tblLook).withTblCaption(tblCaption);
}
}
protected TblPr createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createTblPr();
}
public TblPrBuilder withTblPrChange(CTTblPrChange value) {
if (value!= null) {
object.setTblPrChange(value);
}
return this;
}
public TblPrBuilder withTblStyle(org.docx4j.wml.CTTblPrBase.TblStyle value) {
if (value!= null) {
object.setTblStyle(value);
}
return this;
}
public TblPrBuilder withTblStyle(String val) {
boolean initialized = (val!= null);
if (initialized) {
withTblStyle(tblStyleBuilder.withVal(val).getObject());
}
return this;
}
public TblPrBuilder.TblStyleBuilder getTblStyleBuilder() {
return tblStyleBuilder;
}
public TblPrBuilder withTblpPr(CTTblPPr value) {
if (value!= null) {
object.setTblpPr(value);
}
return this;
}
public TblPrBuilder withTblOverlap(CTTblOverlap value) {
if (value!= null) {
object.setTblOverlap(value);
}
return this;
}
public TblPrBuilder withTblOverlap(STTblOverlap val) {
if (val!= null) {
object.setTblOverlap(new com.alphasystem.docx4j.builder.wml.CTTblOverlapBuilder().withVal(val).getObject());
}
return this;
}
public TblPrBuilder withBidiVisual(BooleanDefaultTrue value) {
if (value!= null) {
object.setBidiVisual(value);
}
return this;
}
public TblPrBuilder withBidiVisual(Boolean val) {
if (val!= null) {
object.setBidiVisual(new BooleanDefaultTrueBuilder().withVal(val).getObject());
}
return this;
}
public TblPrBuilder withTblStyleRowBandSize(org.docx4j.wml.CTTblPrBase.TblStyleRowBandSize value) {
if (value!= null) {
object.setTblStyleRowBandSize(value);
}
return this;
}
public TblPrBuilder withTblStyleRowBandSize(Long val) {
boolean initialized = (val!= null);
if (initialized) {
withTblStyleRowBandSize(tblStyleRowBandSizeBuilder.withVal(val).getObject());
}
return this;
}
public TblPrBuilder.TblStyleRowBandSizeBuilder getTblStyleRowBandSizeBuilder() {
return tblStyleRowBandSizeBuilder;
}
public TblPrBuilder withTblStyleColBandSize(org.docx4j.wml.CTTblPrBase.TblStyleColBandSize value) {
if (value!= null) {
object.setTblStyleColBandSize(value);
}
return this;
}
public TblPrBuilder withTblStyleColBandSize(Long val) {
boolean initialized = (val!= null);
if (initialized) {
withTblStyleColBandSize(tblStyleColBandSizeBuilder.withVal(val).getObject());
}
return this;
}
public TblPrBuilder.TblStyleColBandSizeBuilder getTblStyleColBandSizeBuilder() {
return tblStyleColBandSizeBuilder;
}
public TblPrBuilder withTblW(TblWidth value) {
if (value!= null) {
object.setTblW(value);
}
return this;
}
public TblPrBuilder withJc(Jc value) {
if (value!= null) {
object.setJc(value);
}
return this;
}
public TblPrBuilder withJc(JcEnumeration val) {
if (val!= null) {
object.setJc(new com.alphasystem.docx4j.builder.wml.JcBuilder().withVal(val).getObject());
}
return this;
}
public TblPrBuilder withTblCellSpacing(TblWidth value) {
if (value!= null) {
object.setTblCellSpacing(value);
}
return this;
}
public TblPrBuilder withTblInd(TblWidth value) {
if (value!= null) {
object.setTblInd(value);
}
return this;
}
public TblPrBuilder withTblBorders(TblBorders value) {
if (value!= null) {
object.setTblBorders(value);
}
return this;
}
public TblPrBuilder withShd(CTShd value) {
if (value!= null) {
object.setShd(value);
}
return this;
}
public TblPrBuilder withTblLayout(CTTblLayoutType value) {
if (value!= null) {
object.setTblLayout(value);
}
return this;
}
public TblPrBuilder withTblLayout(STTblLayoutType type) {
if (type!= null) {
object.setTblLayout(new com.alphasystem.docx4j.builder.wml.CTTblLayoutTypeBuilder().withType(type).getObject());
}
return this;
}
public TblPrBuilder withTblCellMar(CTTblCellMar value) {
if (value!= null) {
object.setTblCellMar(value);
}
return this;
}
public TblPrBuilder withTblLook(CTTblLook value) {
if (value!= null) {
object.setTblLook(value);
}
return this;
}
public TblPrBuilder withTblCaption(CTString value) {
if (value!= null) {
object.setTblCaption(value);
}
return this;
}
public TblPrBuilder withTblCaption(String val) {
if (val!= null) {
object.setTblCaption(new com.alphasystem.docx4j.builder.wml.CTStringBuilder().withVal(val).getObject());
}
return this;
}
/**
* Fluent API builder for org.docx4j.wml.CTTblPrBase$TblStyle
.
*
*/
public static class TblStyleBuilder
extends com.alphasystem.docx4j.builder.OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public TblStyleBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public TblStyleBuilder(org.docx4j.wml.CTTblPrBase.TblStyle object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public TblStyleBuilder(org.docx4j.wml.CTTblPrBase.TblStyle src, org.docx4j.wml.CTTblPrBase.TblStyle target) {
this(target);
if (src!= null) {
withVal(src.getVal());
}
}
protected org.docx4j.wml.CTTblPrBase.TblStyle createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createCTTblPrBaseTblStyle();
}
public TblPrBuilder.TblStyleBuilder withVal(String value) {
if (value!= null) {
object.setVal(value);
}
return this;
}
}
/**
* Fluent API builder for org.docx4j.wml.CTTblPrBase$TblStyleColBandSize
.
*
*/
public static class TblStyleColBandSizeBuilder
extends com.alphasystem.docx4j.builder.OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public TblStyleColBandSizeBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public TblStyleColBandSizeBuilder(org.docx4j.wml.CTTblPrBase.TblStyleColBandSize object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public TblStyleColBandSizeBuilder(org.docx4j.wml.CTTblPrBase.TblStyleColBandSize src, org.docx4j.wml.CTTblPrBase.TblStyleColBandSize target) {
this(target);
if (src!= null) {
withVal(WmlBuilderFactory.cloneBigInteger(src.getVal()));
}
}
protected org.docx4j.wml.CTTblPrBase.TblStyleColBandSize createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createCTTblPrBaseTblStyleColBandSize();
}
public TblPrBuilder.TblStyleColBandSizeBuilder withVal(BigInteger value) {
if (value!= null) {
object.setVal(value);
}
return this;
}
/**
* Calls setVal
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public TblPrBuilder.TblStyleColBandSizeBuilder withVal(String value) {
if (value!= null) {
object.setVal(new BigInteger(value));
}
return this;
}
/**
* Calls setVal
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public TblPrBuilder.TblStyleColBandSizeBuilder withVal(Long value) {
if (value!= null) {
object.setVal(BigInteger.valueOf(value));
}
return this;
}
}
/**
* Fluent API builder for org.docx4j.wml.CTTblPrBase$TblStyleRowBandSize
.
*
*/
public static class TblStyleRowBandSizeBuilder
extends com.alphasystem.docx4j.builder.OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public TblStyleRowBandSizeBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public TblStyleRowBandSizeBuilder(org.docx4j.wml.CTTblPrBase.TblStyleRowBandSize object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public TblStyleRowBandSizeBuilder(org.docx4j.wml.CTTblPrBase.TblStyleRowBandSize src, org.docx4j.wml.CTTblPrBase.TblStyleRowBandSize target) {
this(target);
if (src!= null) {
withVal(WmlBuilderFactory.cloneBigInteger(src.getVal()));
}
}
protected org.docx4j.wml.CTTblPrBase.TblStyleRowBandSize createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createCTTblPrBaseTblStyleRowBandSize();
}
public TblPrBuilder.TblStyleRowBandSizeBuilder withVal(BigInteger value) {
if (value!= null) {
object.setVal(value);
}
return this;
}
/**
* Calls setVal
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public TblPrBuilder.TblStyleRowBandSizeBuilder withVal(String value) {
if (value!= null) {
object.setVal(new BigInteger(value));
}
return this;
}
/**
* Calls setVal
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public TblPrBuilder.TblStyleRowBandSizeBuilder withVal(Long value) {
if (value!= null) {
object.setVal(BigInteger.valueOf(value));
}
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy