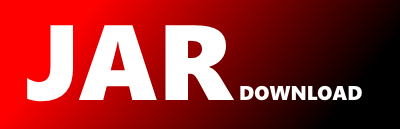
io.github.shanqiang.table.TableBuilder Maven / Gradle / Ivy
package io.github.shanqiang.table;
import io.github.shanqiang.exception.UnknownTypeException;
import io.github.shanqiang.offheap.ByteArray;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import static java.lang.Double.parseDouble;
import static java.lang.Integer.parseInt;
import static java.lang.Long.parseLong;
public class TableBuilder {
private final LinkedHashMap columnName2Index = new LinkedHashMap<>();
private final List columns;
public TableBuilder(Map columnTypeMap) {
if (columnTypeMap.size() <= 0) {
throw new IllegalArgumentException("at least one column");
}
int i = 0;
columns = new ArrayList<>(columnTypeMap.size());
for (String columnName : columnTypeMap.keySet()) {
columns.add(new Column(columnName, columnTypeMap.get(columnName)));
columnName2Index.put(columnName, i++);
}
}
public TableBuilder(List columns) {
if (columns.size() <= 0) {
throw new IllegalArgumentException("at least one column");
}
this.columns = columns;
}
public Column getColumn(String columnName) {
return columns.get(columnName2Index.get(columnName));
}
public int size() {
return columns.get(0).size();
}
public int columnSize() {
return columns.size();
}
public Type getType(int index) {
return columns.get(index).getType();
}
public TableBuilder append(int index, ByteArray value) {
if (null == value) {
columns.get(index).add(null);
return this;
}
Type type = columns.get(index).getType();
Comparable comparable;
if (type != Type.VARBYTE) {
String string = new String(value.getBytes(), value.getOffset(), value.getLength());
string = string.trim();
switch (type) {
case DOUBLE:
comparable = parseDouble(string);
break;
case BIGINT:
comparable = parseLong(string);
break;
case INT:
comparable = parseInt(string);
break;
case BIGDECIMAL:
comparable = new BigDecimal(string);
break;
default:
throw new UnknownTypeException(type.name());
}
} else {
comparable = value;
}
columns.get(index).add(comparable);
return this;
}
public TableBuilder append(int index, Integer value) {
appendValue(index, value);
return this;
}
public TableBuilder append(int index, Long value) {
appendValue(index, value);
return this;
}
public TableBuilder append(int index, Double value) {
appendValue(index, value);
return this;
}
public TableBuilder append(int index, String value) {
appendValue(index, value);
return this;
}
public TableBuilder append(int index, BigDecimal value) {
appendValue(index, value);
return this;
}
public TableBuilder appendValue(int index, Object value) {
if (null == value) {
columns.get(index).add(null);
return this;
}
if (value.getClass() == byte[].class) {
columns.get(index).add(new ByteArray((byte[]) value));
} else {
columns.get(index).add((Comparable) value);
}
return this;
}
public Table build() {
return new Table(columns);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy