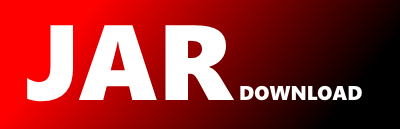
shz.api.server.SysApi Maven / Gradle / Ivy
package shz.api.server;
import shz.orm.annotation.Id;
import shz.orm.annotation.Table;
@Table(name = "sys_api")
public class SysApi {
/**
* 主键
*/
@Id
private Long id;
/**
* 应用程序名
*/
private String appName;
/**
* 分组名
*/
private String groupName;
/**
* 注解路径
*/
private String notePath;
/**
* 路径
*/
private String path;
/**
* 请求方法
*/
private String method;
/**
* 名称
*/
private String name;
/**
* 请求参数
*/
private String req;
/**
* 响应结果
*/
private String res;
/**
* 权限值
*/
private Integer permission;
/**
* 是否要登录
*/
private Boolean login;
/**
* 排序
*/
private Integer sort;
/**
* 是否可用
*/
private Boolean enabled;
/**
* 每秒限制次数
*/
private Integer qpsLimit;
/**
* 可重复读流
*/
private Boolean reusable;
/**
* 重复请求阈值毫秒数
*/
private Long repeatedLimit;
/**
* 是否记录访问
*/
private Boolean record;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getAppName() {
return appName;
}
public void setAppName(String appName) {
this.appName = appName;
}
public String getGroupName() {
return groupName;
}
public void setGroupName(String groupName) {
this.groupName = groupName;
}
public String getNotePath() {
return notePath;
}
public void setNotePath(String notePath) {
this.notePath = notePath;
}
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
public String getMethod() {
return method;
}
public void setMethod(String method) {
this.method = method;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getReq() {
return req;
}
public void setReq(String req) {
this.req = req;
}
public String getRes() {
return res;
}
public void setRes(String res) {
this.res = res;
}
public Integer getPermission() {
return permission;
}
public void setPermission(Integer permission) {
this.permission = permission;
}
public Boolean getLogin() {
return login;
}
public void setLogin(Boolean login) {
this.login = login;
}
public Integer getSort() {
return sort;
}
public void setSort(Integer sort) {
this.sort = sort;
}
public Boolean getEnabled() {
return enabled;
}
public void setEnabled(Boolean enabled) {
this.enabled = enabled;
}
public Integer getQpsLimit() {
return qpsLimit;
}
public void setQpsLimit(Integer qpsLimit) {
this.qpsLimit = qpsLimit;
}
public Boolean getReusable() {
return reusable;
}
public void setReusable(Boolean reusable) {
this.reusable = reusable;
}
public Long getRepeatedLimit() {
return repeatedLimit;
}
public void setRepeatedLimit(Long repeatedLimit) {
this.repeatedLimit = repeatedLimit;
}
public Boolean getRecord() {
return record;
}
public void setRecord(Boolean record) {
this.record = record;
}
@Override
public String toString() {
return "SysApi{" +
"id=" + id +
", appName='" + appName + '\'' +
", groupName='" + groupName + '\'' +
", notePath='" + notePath + '\'' +
", path='" + path + '\'' +
", method='" + method + '\'' +
", name='" + name + '\'' +
", req='" + req + '\'' +
", res='" + res + '\'' +
", permission=" + permission +
", login=" + login +
", sort=" + sort +
", enabled=" + enabled +
", qpsLimit=" + qpsLimit +
", reusable=" + reusable +
", repeatedLimit=" + repeatedLimit +
", record=" + record +
'}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy