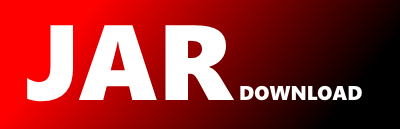
shz.core.NullHelp Maven / Gradle / Ivy
package shz.core;
import shz.core.constant.ArrayConstant;
import java.lang.reflect.Array;
import java.util.*;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Supplier;
@SuppressWarnings("unchecked")
public final class NullHelp {
private NullHelp() {
throw new IllegalStateException();
}
//////////////////////////////CharSequence
public static boolean isEmpty(CharSequence cs) {
return cs == null || cs.length() == 0;
}
public static boolean nonEmpty(CharSequence cs) {
return !isEmpty(cs);
}
public static boolean isBlank(CharSequence cs) {
int len;
if (cs == null || (len = cs.length()) == 0) return true;
for (int i = 0; i < len; ++i) if (!Character.isWhitespace(cs.charAt(i))) return false;
return true;
}
public static boolean nonBlank(CharSequence cs) {
return !isBlank(cs);
}
//////////////////////////////CharSequence
//////////////////////////////array
public static boolean isEmpty(boolean[] array) {
return array == null || array.length == 0;
}
public static boolean nonEmpty(boolean[] array) {
return !isEmpty(array);
}
public static boolean isEmpty(byte[] array) {
return array == null || array.length == 0;
}
public static boolean nonEmpty(byte[] array) {
return !isEmpty(array);
}
public static boolean isEmpty(char[] array) {
return array == null || array.length == 0;
}
public static boolean nonEmpty(char[] array) {
return !isEmpty(array);
}
public static boolean isBlank(char[] array) {
if (isEmpty(array)) return true;
for (char e : array) if (!Character.isWhitespace(e)) return false;
return true;
}
public static boolean nonBlank(char[] array) {
return !isBlank(array);
}
public static boolean isEmpty(short[] array) {
return array == null || array.length == 0;
}
public static boolean nonEmpty(short[] array) {
return !isEmpty(array);
}
public static boolean isEmpty(int[] array) {
return array == null || array.length == 0;
}
public static boolean nonEmpty(int[] array) {
return !isEmpty(array);
}
public static boolean isEmpty(long[] array) {
return array == null || array.length == 0;
}
public static boolean nonEmpty(long[] array) {
return !isEmpty(array);
}
public static boolean isEmpty(float[] array) {
return array == null || array.length == 0;
}
public static boolean nonEmpty(float[] array) {
return !isEmpty(array);
}
public static boolean isEmpty(double[] array) {
return array == null || array.length == 0;
}
public static boolean nonEmpty(double[] array) {
return !isEmpty(array);
}
public static boolean isEmpty(Object[] array) {
return array == null || array.length == 0;
}
public static boolean nonEmpty(Object[] array) {
return !isEmpty(array);
}
public static boolean isBlank(Object[] array) {
if (isEmpty(array)) return true;
for (Object obj : array) if (!isBlank(obj)) return false;
return true;
}
public static boolean nonBlank(Object[] array) {
return !isBlank(array);
}
//////////////////////////////array
public static boolean isEmpty(Collection> collection) {
return collection == null || collection.size() == 0;
}
public static boolean nonEmpty(Collection> collection) {
return !isEmpty(collection);
}
public static boolean isBlank(Collection> collection) {
if (isEmpty(collection)) return true;
for (Object obj : collection) if (!isBlank(obj)) return false;
return true;
}
public static boolean nonBlank(Collection> collection) {
return !isBlank(collection);
}
public static boolean isEmpty(Map, ?> map) {
return map == null || map.size() == 0;
}
public static boolean nonEmpty(Map, ?> map) {
return !isEmpty(map);
}
public static boolean isBlank(Map, ?> map) {
if (isEmpty(map)) return true;
for (Object obj : map.values()) if (!isBlank(obj)) return false;
return true;
}
public static boolean nonBlank(Map, ?> map) {
return !isBlank(map);
}
public static boolean isEmpty(Object obj) {
if (obj == null) return true;
if (obj instanceof CharSequence) return isEmpty((CharSequence) obj);
if (obj instanceof Collection) return isEmpty((Collection>) obj);
if (obj instanceof Map) return isEmpty((Map, ?>) obj);
if (obj instanceof Object[]) return isEmpty((Object[]) obj);
if (obj instanceof boolean[]) return isEmpty((boolean[]) obj);
if (obj instanceof byte[]) return isEmpty((byte[]) obj);
if (obj instanceof char[]) return isEmpty((char[]) obj);
if (obj instanceof short[]) return isEmpty((short[]) obj);
if (obj instanceof int[]) return isEmpty((int[]) obj);
if (obj instanceof long[]) return isEmpty((long[]) obj);
if (obj instanceof float[]) return isEmpty((float[]) obj);
if (obj instanceof double[]) return isEmpty((double[]) obj);
return false;
}
public static boolean nonEmpty(Object obj) {
return !isEmpty(obj);
}
public static boolean isBlank(Object obj) {
if (obj == null) return true;
if (obj instanceof CharSequence) return isBlank((CharSequence) obj);
if (obj instanceof Collection) return isBlank((Collection>) obj);
if (obj instanceof Map) return isBlank((Map, ?>) obj);
if (obj instanceof Object[]) return isBlank((Object[]) obj);
if (obj instanceof char[]) return isBlank((char[]) obj);
return false;
}
public static boolean nonBlank(Object obj) {
return !isBlank(obj);
}
public static boolean isZero(Number number) {
return number == null || number.doubleValue() == 0D;
}
public static boolean isZero(Object obj) {
if (obj == null) return true;
if (obj instanceof Number) return ((Number) obj).doubleValue() == 0D;
return false;
}
public static T off(T t) {
if (t == null) return null;
if (t instanceof List) return ((List>) t).isEmpty() ? (T) Collections.EMPTY_LIST : t;
if (t instanceof Set) return ((Set>) t).isEmpty() ? (T) Collections.EMPTY_SET : t;
if (t instanceof Map) return ((Map, ?>) t).isEmpty() ? (T) Collections.EMPTY_MAP : t;
if (t instanceof Object[]) return ((Object[]) t).length == 0 ? (T) emptyArray(t.getClass()) : t;
if (t instanceof boolean[]
|| t instanceof byte[]
|| t instanceof char[]
|| t instanceof short[]
|| t instanceof int[]
|| t instanceof long[]
|| t instanceof float[]
|| t instanceof double[])
return isEmpty(t) ? (T) emptyPrimitiveArray(t.getClass()) : t;
return t;
}
private static final Map, Object[]> EMPTY_ARRAY_CACHE = new ConcurrentHashMap<>(128);
private static T[] emptyArray(Class> cls) {
if (cls == Object[].class) return (T[]) ArrayConstant.EMPTY_OBJECT_ARRAY;
if (cls == String[].class) return (T[]) ArrayConstant.EMPTY_STRING_ARRAY;
return (T[]) EMPTY_ARRAY_CACHE.computeIfAbsent(cls, k -> (T[]) Array.newInstance(cls.getComponentType(), 0));
}
private static Object emptyPrimitiveArray(Class> cls) {
if (cls == boolean[].class) return ArrayConstant.EMPTY_BOOLEAN_ARRAY;
if (cls == byte[].class) return ArrayConstant.EMPTY_BYTE_ARRAY;
if (cls == char[].class) return ArrayConstant.EMPTY_CHAR_ARRAY;
if (cls == short[].class) return ArrayConstant.EMPTY_SHORT_ARRAY;
if (cls == int[].class) return ArrayConstant.EMPTY_INT_ARRAY;
if (cls == long[].class) return ArrayConstant.EMPTY_LONG_ARRAY;
if (cls == float[].class) return ArrayConstant.EMPTY_FLOAT_ARRAY;
if (cls == double[].class) return ArrayConstant.EMPTY_DOUBLE_ARRAY;
return null;
}
public static T empty(Class> cls) {
if (cls == null) throw new IllegalStateException();
if (List.class.isAssignableFrom(cls)) return (T) Collections.EMPTY_LIST;
if (Set.class.isAssignableFrom(cls)) return (T) Collections.EMPTY_SET;
if (Map.class.isAssignableFrom(cls)) return (T) Collections.EMPTY_MAP;
if (Object[].class.isAssignableFrom(cls)) return (T) emptyArray(cls);
return (T) emptyPrimitiveArray(cls);
}
public static T empty(Class> cls, T t) {
return t == null ? empty(cls) : off(t);
}
public static T nonNull(T t) {
if ((t = off(t)) == null) throw new NullPointerException();
return t;
}
public static T nonNull(Class extends T> cls, T t) {
if ((t = empty(cls, t)) == null) throw new NullPointerException();
return t;
}
public static T blankOrElse(Object obj, T defaultValue) {
return isBlank(obj) ? defaultValue : (T) obj;
}
public static T blankOrElse(Object obj, Supplier supplier) {
return isBlank(obj) ? supplier.get() : (T) obj;
}
public static T emptyOrElse(Object obj, T defaultValue) {
return isEmpty(obj) ? defaultValue : (T) obj;
}
public static T emptyOrElse(Object obj, Supplier supplier) {
return isEmpty(obj) ? supplier.get() : (T) obj;
}
public static T nullOrElse(Object obj, T defaultValue) {
return obj == null ? defaultValue : (T) obj;
}
public static T nullOrElse(Object obj, Supplier supplier) {
return obj == null ? supplier.get() : (T) obj;
}
public static boolean isAnyEmpty(Object obj1, Object obj2) {
return isEmpty(obj1) || isEmpty(obj2);
}
public static boolean nonAnyEmpty(Object obj1, Object obj2) {
return !isAnyEmpty(obj1, obj2);
}
public static boolean isAnyEmpty(Object obj1, Object obj2, Object obj3) {
return isEmpty(obj1) || isEmpty(obj2) || isEmpty(obj3);
}
public static boolean nonAnyEmpty(Object obj1, Object obj2, Object obj3) {
return !isAnyEmpty(obj1, obj2, obj3);
}
public static boolean isAnyEmpty(Object... objects) {
for (Object obj : objects) if (isEmpty(obj)) return true;
return false;
}
public static boolean nonAnyEmpty(Object... objects) {
return !isAnyEmpty(objects);
}
public static boolean isAnyBlank(Object obj1, Object obj2) {
return isBlank(obj1) || isBlank(obj2);
}
public static boolean nonAnyBlank(Object obj1, Object obj2) {
return !isAnyBlank(obj1, obj2);
}
public static boolean isAnyBlank(Object obj1, Object obj2, Object obj3) {
return isBlank(obj1) || isBlank(obj2) || isBlank(obj3);
}
public static boolean nonAnyBlank(Object obj1, Object obj2, Object obj3) {
return !isAnyBlank(obj1, obj2, obj3);
}
public static boolean isAnyBlank(Object... objects) {
for (Object obj : objects) if (isBlank(obj)) return true;
return false;
}
public static boolean nonAnyBlank(Object... objects) {
return !isAnyBlank(objects);
}
public static boolean isAnyNull(Object obj1, Object obj2) {
return obj1 == null || obj2 == null;
}
public static boolean nonAnyNull(Object obj1, Object obj2) {
return !isAnyNull(obj1, obj2);
}
public static boolean isAnyNull(Object obj1, Object obj2, Object obj3) {
return obj1 == null || obj2 == null || obj3 == null;
}
public static boolean nonAnyNull(Object obj1, Object obj2, Object obj3) {
return !isAnyNull(obj1, obj2, obj3);
}
public static boolean isAnyNull(Object... objects) {
for (Object obj : objects) if (obj == null) return true;
return false;
}
public static boolean nonAnyNull(Object... objects) {
return !isAnyNull(objects);
}
public static void requireNon(boolean condition) {
if (condition) throw new IllegalArgumentException();
}
public static void requireNonEmpty(Object obj) {
if (isEmpty(obj)) throw new IllegalArgumentException();
}
public static void requireNonAnyEmpty(Object obj1, Object obj2) {
if (isAnyEmpty(obj1, obj2)) throw new IllegalArgumentException();
}
public static void requireNonAnyEmpty(Object obj1, Object obj2, Object obj3) {
if (isAnyEmpty(obj1, obj2, obj3)) throw new IllegalArgumentException();
}
public static void requireNonAnyEmpty(Object... objects) {
if (isAnyEmpty(objects)) throw new IllegalArgumentException();
}
public static void requireNonBlank(Object obj) {
if (isBlank(obj)) throw new IllegalArgumentException();
}
public static void requireNonAnyBlank(Object obj1, Object obj2) {
if (isAnyBlank(obj1, obj2)) throw new IllegalArgumentException();
}
public static void requireNonAnyBlank(Object obj1, Object obj2, Object obj3) {
if (isAnyBlank(obj1, obj2, obj3)) throw new IllegalArgumentException();
}
public static void requireNonAnyBlank(Object... objects) {
if (isAnyBlank(objects)) throw new IllegalArgumentException();
}
public static void requireNonNull(Object obj) {
if (obj == null) throw new NullPointerException();
}
public static void requireNonAnyNull(Object obj1, Object obj2) {
if (isAnyNull(obj1, obj2)) throw new NullPointerException();
}
public static void requireNonAnyNull(Object obj1, Object obj2, Object obj3) {
if (isAnyNull(obj1, obj2, obj3)) throw new NullPointerException();
}
public static void requireNonAnyNull(Object... objects) {
if (isAnyNull(objects)) throw new NullPointerException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy