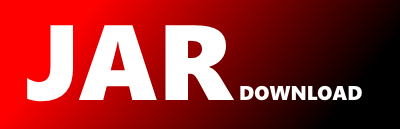
shz.core.lock.NonReentrantLock Maven / Gradle / Ivy
package shz.core.lock;
import java.io.Serializable;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.locks.AbstractQueuedSynchronizer;
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.Lock;
/**
* 一个锁对应一个AQS阻塞队列,对应多个条件变量,每个条件变量有自己的一个条件队列
* 不可重入锁
*/
public class NonReentrantLock implements Lock, Serializable {
private static final long serialVersionUID = 2471059261620346798L;
private static class Sync extends AbstractQueuedSynchronizer {
private static final long serialVersionUID = 7790452590145398391L;
protected boolean isHeldExclusively() {
// 规定state=1为锁已被持有
return getState() == 1;
}
protected boolean tryAcquire(int acquires) {
assert acquires == 1;
if (compareAndSetState(0, 1)) {
// 如果state=0则尝试获取锁
setExclusiveOwnerThread(Thread.currentThread());
return true;
}
return false;
}
protected boolean tryRelease(int releases) {
assert releases == 1;
if (getState() == 0)
throw new IllegalMonitorStateException();
// 释放锁
setExclusiveOwnerThread(null);
setState(0);
return true;
}
Condition newCondition() {
// 条件变量
return new ConditionObject();
}
}
private final Sync sync = new Sync();
@Override
public final void lock() {
sync.acquire(1);
}
@Override
public final void lockInterruptibly() throws InterruptedException {
sync.acquireInterruptibly(1);
}
@Override
public final boolean tryLock() {
return sync.tryAcquire(1);
}
@Override
public final boolean tryLock(long time, TimeUnit unit) throws InterruptedException {
return sync.tryAcquireNanos(1, unit.toNanos(time));
}
@Override
public final void unlock() {
sync.release(1);
}
@Override
public final Condition newCondition() {
return sync.newCondition();
}
public final boolean isLocked() {
return sync.isHeldExclusively();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy