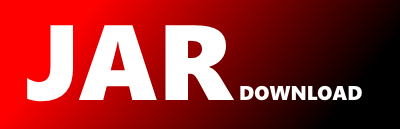
shz.core.NetHelp Maven / Gradle / Ivy
package shz.core;
import java.io.IOException;
import java.net.*;
import java.util.*;
import java.util.function.Predicate;
import java.util.regex.Pattern;
public final class NetHelp {
private NetHelp() {
throw new IllegalStateException();
}
public static final Pattern IPV4 = Pattern.compile("\\b((?!\\d\\d\\d)\\d+|1\\d\\d|2[0-4]\\d|25[0-5])\\.((?!\\d\\d\\d)\\d+|1\\d\\d|2[0-4]\\d|25[0-5])\\.((?!\\d\\d\\d)\\d+|1\\d\\d|2[0-4]\\d|25[0-5])\\.((?!\\d\\d\\d)\\d+|1\\d\\d|2[0-4]\\d|25[0-5])\\b");
public static final Pattern IPV6 = Pattern.compile("(([\\da-fA-F]{1,4}:){7}[\\da-fA-F]{1,4}|([\\da-fA-F]{1,4}:){1,7}:|([\\da-fA-F]{1,4}:){1,6}:[\\da-fA-F]{1,4}|([\\da-fA-F]{1,4}:){1,5}(:[\\da-fA-F]{1,4}){1,2}|([\\da-fA-F]{1,4}:){1,4}(:[\\da-fA-F]{1,4}){1,3}|([\\da-fA-F]{1,4}:){1,3}(:[\\da-fA-F]{1,4}){1,4}|([\\da-fA-F]{1,4}:){1,2}(:[\\da-fA-F]{1,4}){1,5}|[\\da-fA-F]{1,4}:((:[\\da-fA-F]{1,4}){1,6})|:((:[\\da-fA-F]{1,4}){1,7}|:)|fe80:(:[\\da-fA-F]{0,4}){0,4}%[\\da-zA-Z]+|::(ffff(:0{1,4})?:)?((25[0-5]|(2[0-4]|1?\\d)?\\d)\\.){3}(25[0-5]|(2[0-4]|1?\\d)?\\d)|([\\da-fA-F]{1,4}:){1,4}:((25[0-5]|(2[0-4]|1?\\d)?\\d)\\.){3}(25[0-5]|(2[0-4]|1?\\d)?\\d))");
public static boolean isIpv4(String ip) {
if (NullHelp.isBlank(ip)) return false;
return IPV4.matcher(ip).matches();
}
public static boolean isIpv6(String ip) {
if (NullHelp.isBlank(ip)) return false;
return IPV6.matcher(ip).matches();
}
public static long ipv4ToLong(String ip) {
if (!isIpv4(ip)) return -1L;
String[] a = ip.split("\\.");
return (Long.parseLong(a[0]) << 24
| Long.parseLong(a[1]) << 16
| Long.parseLong(a[2]) << 8
| Long.parseLong(a[3]));
}
public static String longToIpv4(long ip) {
return (ip >> 24 & 0xff) +
"." + (ip >> 16 & 0xff) +
"." + (ip >> 8 & 0xff) +
"." + (ip & 0xff);
}
public static boolean isInnerIp(String ip) {
if ("127.0.0.1".equals(ip)) return true;
long longIp = ipv4ToLong(ip);
if (longIp < 0L) return false;
//c类: 192.168.0.0 - 192.168.255.255
if (longIp >= ipv4ToLong("192.168.0.0")) return longIp <= ipv4ToLong("192.168.255.255");
//b类: 172.16.0.0 - 172.31.255.255
if (longIp >= ipv4ToLong("172.16.0.0")) return longIp <= ipv4ToLong("172.31.255.255");
//a类: 10.0.0.0 - 10.255.255.255
if (longIp >= ipv4ToLong("10.0.0.0")) return longIp <= ipv4ToLong("10.255.255.255");
return false;
}
public static String getIpByHost(String hostName) {
try {
return InetAddress.getByName(hostName).getHostAddress();
} catch (UnknownHostException e) {
return hostName;
}
}
public static Set getLocalAddresses(Predicate predicate) {
Enumeration networkInterfaces;
try {
networkInterfaces = NetworkInterface.getNetworkInterfaces();
} catch (SocketException e) {
throw PRException.of(e);
}
if (networkInterfaces == null) return Collections.emptySet();
Set result = new LinkedHashSet<>();
while (networkInterfaces.hasMoreElements()) {
NetworkInterface networkInterface = networkInterfaces.nextElement();
Enumeration inetAddresses = networkInterface.getInetAddresses();
while (inetAddresses.hasMoreElements()) {
InetAddress inetAddress = inetAddresses.nextElement();
if (inetAddress == null || (predicate != null && !predicate.test(inetAddress))) continue;
result.add(inetAddress);
}
}
return result.isEmpty() ? Collections.emptySet() : result;
}
public static InetAddress getLocalhost() {
Set localAddresses = getLocalAddresses(inetAddress -> !inetAddress.isLoopbackAddress() && inetAddress instanceof Inet4Address);
if (!localAddresses.isEmpty()) {
InetAddress result = null;
for (InetAddress inetAddress : localAddresses) {
if (!inetAddress.isSiteLocalAddress()) return inetAddress;
if (result == null) result = inetAddress;
}
return result;
}
try {
return InetAddress.getLocalHost();
} catch (UnknownHostException e) {
return null;
}
}
public static byte[] getHardwareAddress(InetAddress inetAddress) {
if (inetAddress == null) return null;
try {
NetworkInterface networkInterface = NetworkInterface.getByInetAddress(inetAddress);
return networkInterface == null ? null : networkInterface.getHardwareAddress();
} catch (SocketException e) {
throw PRException.of(e);
}
}
public static String getMacAddress(InetAddress inetAddress, String separator) {
if (inetAddress == null) return null;
byte[] mac = getHardwareAddress(inetAddress);
if (mac == null) return null;
StringBuilder sb = new StringBuilder();
String s = Integer.toHexString(mac[0] & 0xff);
if (s.length() == 1) sb.append(0);
sb.append(s);
for (int i = 1; i < mac.length; ++i) {
sb.append(separator);
s = Integer.toHexString(mac[i] & 0xff);
if (s.length() == 1) sb.append(0);
sb.append(s);
}
return sb.toString();
}
public static String getMacAddress(InetAddress inetAddress) {
return getMacAddress(inetAddress, "-");
}
public static boolean ping(String ip, int timeoutMills) {
try {
return InetAddress.getByName(ip).isReachable(timeoutMills);
} catch (IOException e) {
return false;
}
}
public static boolean isOpen(InetSocketAddress address, int timeoutMills) {
try (Socket sc = new Socket()) {
sc.connect(address, timeoutMills);
} catch (IOException e) {
return false;
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy