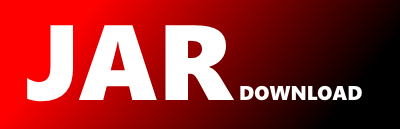
shz.core.ZipHelp Maven / Gradle / Ivy
package shz.core;
import shz.core.msg.ServerFailureMsg;
import java.io.*;
import java.nio.charset.Charset;
import java.util.*;
import java.util.jar.*;
import java.util.zip.*;
public final class ZipHelp {
private ZipHelp() {
throw new IllegalStateException();
}
public static void compress(Set srcs, File des, int dataSize) {
ZipOutputStream zos = IOHelp.getZos(des);
srcs.stream().filter(Objects::nonNull).filter(f -> f.exists() && f.canRead()).forEach(f -> compress0(zos, dataSize, "", f));
}
public static void compress0(ZipOutputStream zos, int dataSize, String entryName, File... files) {
Arrays.stream(files).forEach(f -> {
if (f.isFile()) {
BufferedInputStream bis = IOHelp.getBis(f);
try {
zos.putNextEntry(new ZipEntry(entryName + f.getName()));
} catch (IOException e) {
throw PRException.of(e);
}
IOHelp.read(bis, zos, dataSize, null, (i, o) -> {
try {
o.closeEntry();
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(i);
}
});
} else {
File[] fs = f.listFiles();
if (NullHelp.isEmpty(fs)) try {
zos.putNextEntry(new ZipEntry(entryName + f.getName() + "/"));
} catch (IOException e) {
throw PRException.of(e);
}
else compress0(zos, dataSize, entryName + "/" + f.getName(), fs);
}
});
}
public static void compress(Set srcs, File des) {
compress(srcs, des, IOHelp.DEFAULT_DATA_SIZE);
}
public static void decompress(File src, File des, int dataSize) {
if (src == null) return;
FileHelp.checkCopyFolder(des);
ZipFile zip;
try {
zip = new ZipFile(src);
} catch (IOException e) {
throw PRException.of(e);
}
Enumeration extends ZipEntry> entries = zip.entries();
InputStream is = null;
try {
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
File f = new File(des, entry.getName());
if (entry.isDirectory()) {
if (!f.mkdirs() && !f.exists()) throw new IllegalArgumentException();
continue;
}
is = zip.getInputStream(entry);
IOHelp.read(is, IOHelp.getBos(f), dataSize, null, (i, o) -> IOHelp.close(o));
}
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(is);
}
}
public static void decompress(File src, File des) {
decompress(src, des, IOHelp.DEFAULT_DATA_SIZE);
}
public static void updateZipFile(File file, Map entryNameFiles, int dataSize) {
File tempFile;
try {
tempFile = File.createTempFile(file.getName(), null);
} catch (IOException e) {
throw PRException.of(e);
}
FileHelp.deleteFile(tempFile);
ServerFailureMsg.requireNon(!file.renameTo(tempFile), "文件不可重命名");
ZipInputStream zis = null;
ZipOutputStream zos = null;
ZipEntry entry;
try {
zis = IOHelp.getZis(tempFile);
zos = IOHelp.getZos(file);
while ((entry = zis.getNextEntry()) != null) if (!entryNameFiles.containsKey(entry.getName())) {
//拷贝原文件,去除需要更新的文件
zos.putNextEntry(new ZipEntry(entry.getName()));
IOHelp.read(zis, zos, dataSize, null, (i, o) -> {
try {
o.closeEntry();
} catch (IOException e) {
throw PRException.of(e);
}
});
}
} catch (IOException e) {
IOHelp.close(zos);
throw PRException.of(e);
} finally {
IOHelp.close(zis);
}
try {
for (Map.Entry kv : entryNameFiles.entrySet()) {
//添加更新的文件
BufferedInputStream bis = IOHelp.getBis(kv.getValue());
zos.putNextEntry(new ZipEntry(kv.getKey()));
IOHelp.read(bis, zos, dataSize, null, (i, o) -> {
try {
o.closeEntry();
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(i);
}
});
}
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(zos);
FileHelp.deleteFile(tempFile);
}
}
public static InputStream getIsFromZis(ZipInputStream zis, String entryName, int dataSize) {
if (zis == null || NullHelp.isBlank(entryName)) return null;
ZipEntry entry;
try {
while ((entry = zis.getNextEntry()) != null)
if (entry.getName().equals(entryName))
return new ByteArrayInputStream(IOHelp.read(zis, dataSize, null));
} catch (IOException e) {
throw PRException.of(e);
}
return null;
}
public static InputStream getIsFromZipFile(ZipFile zip, String entryName, int dataSize) {
if (zip == null || NullHelp.isBlank(entryName)) return null;
Enumeration extends ZipEntry> entries = zip.entries();
InputStream is;
try {
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
if (entry.getName().equals(entryName)) {
is = zip.getInputStream(entry);
return new ByteArrayInputStream(IOHelp.read(is, dataSize, null));
}
}
} catch (IOException e) {
throw PRException.of(e);
}
return null;
}
public static void updateJarFile(File file, Map entryNameFiles, int dataSize) {
File tempFile;
try {
tempFile = File.createTempFile(file.getName(), null);
} catch (IOException e) {
throw PRException.of(e);
}
FileHelp.deleteFile(tempFile);
ServerFailureMsg.requireNon(!file.renameTo(tempFile), "文件不可重命名");
JarInputStream jis = null;
JarOutputStream jos = null;
JarEntry entry;
try {
jis = IOHelp.getJis(tempFile);
jos = IOHelp.getJos(file);
while ((entry = (JarEntry) jis.getNextEntry()) != null) {
if (!entryNameFiles.containsKey(entry.getName())) {
jos.putNextEntry(new JarEntry(entry.getName()));
IOHelp.read(jis, jos, dataSize, null, (i, o) -> {
try {
o.closeEntry();
} catch (IOException e) {
throw PRException.of(e);
}
});
}
}
} catch (IOException e) {
IOHelp.close(jos);
throw PRException.of(e);
} finally {
IOHelp.close(jis);
}
try {
for (Map.Entry kv : entryNameFiles.entrySet()) {
BufferedInputStream bis = IOHelp.getBis(kv.getValue());
jos.putNextEntry(new JarEntry(kv.getKey()));
IOHelp.read(bis, jos, dataSize, null, (i, o) -> {
try {
o.closeEntry();
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(i);
}
});
}
} catch (IOException e) {
IOHelp.close(jos);
throw PRException.of(e);
} finally {
IOHelp.close(jos);
FileHelp.deleteFile(tempFile);
}
}
public static InputStream getIsFromJis(JarInputStream jis, String entryName, int dataSize) {
if (jis == null || NullHelp.isBlank(entryName)) return null;
JarEntry entry;
try {
while ((entry = (JarEntry) jis.getNextEntry()) != null) {
if (entry.getName().equals(entryName))
return new ByteArrayInputStream(IOHelp.read(jis, dataSize, null));
}
} catch (IOException e) {
throw PRException.of(e);
}
return null;
}
public static InputStream getIsFromJarFile(JarFile jar, String entryName, int dataSize) {
if (jar == null || NullHelp.isBlank(entryName)) return null;
Enumeration entries = jar.entries();
InputStream is;
try {
while (entries.hasMoreElements()) {
JarEntry entry = entries.nextElement();
if (entry.getName().equals(entryName)) {
is = jar.getInputStream(entry);
return new ByteArrayInputStream(IOHelp.read(is, dataSize, null));
}
}
} catch (IOException e) {
throw PRException.of(e);
}
return null;
}
public static void makeJar(File file, JarOutputStream jos) {
File[] files = file.listFiles();
if (NullHelp.nonEmpty(files)) try {
int idx = file.getAbsolutePath().replace("\\\\", "/").lastIndexOf("/") + 1;
for (File f : files) makeJar0(f, jos, idx);
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(jos);
}
}
private static void makeJar0(File file, JarOutputStream jos, int idx) throws IOException {
if (file.isDirectory()) {
File[] files = file.listFiles();
if (NullHelp.nonEmpty(files)) for (File f : files) makeJar0(f, jos, idx);
} else {
BufferedInputStream bis = IOHelp.getBis(file);
jos.putNextEntry(new JarEntry(file.getAbsolutePath().replace("\\\\", "/").substring(idx)));
IOHelp.read(bis, jos, IOHelp.DEFAULT_DATA_SIZE, null, (i, o) -> {
try {
o.closeEntry();
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(i);
}
});
}
}
public static void makeRunnableJar(JarInputStream jis, JarOutputStream jos) {
JarEntry entry;
try {
while ((entry = jis.getNextJarEntry()) != null) {
if (JarFile.MANIFEST_NAME.equals(entry.getName())) continue;
jos.putNextEntry(entry);
IOHelp.read(jis, jos, IOHelp.DEFAULT_DATA_SIZE, null, (i, o) -> {
try {
o.closeEntry();
} catch (IOException e) {
throw PRException.of(e);
}
});
}
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(jos, jis);
}
}
public static void makeRunnableJar(JarInputStream jis, File newJar, Manifest manifest) {
makeRunnableJar(jis, IOHelp.getJos(newJar, false, manifest));
}
public static void makeRunnableJar(JarInputStream jis, File newJar, String mainClass, String version) {
Manifest manifest = jis.getManifest();
if (manifest == null) manifest = new Manifest();
Attributes a = manifest.getMainAttributes();
String oldMainClass = a.putValue("Main-Class", mainClass);
if (oldMainClass != null) {
IOHelp.close(jis);
return;
}
if (version == null) {
version = (String) a.get("Manifest-Version");
if (version == null) version = (String) a.get("Signature-Version");
}
a.putValue("Manifest-Version", version);
makeRunnableJar(jis, IOHelp.getJos(newJar, false, manifest));
}
public static String read(InputStream is, Charset charset) {
BufferedReader br;
try {
br = new BufferedReader(new InputStreamReader(new GZIPInputStream(new ByteArrayInputStream(IOHelp.read(is))), charset));
} catch (IOException e) {
throw PRException.of(e);
}
StringWriter sw = new StringWriter();
IOHelp.read(br, sw);
return sw.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy