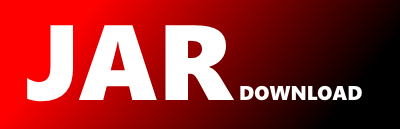
shz.core.model.RpcPayload Maven / Gradle / Ivy
package shz.core.model;
import java.io.Serializable;
import java.util.Arrays;
public final class RpcPayload implements Serializable {
private static final long serialVersionUID = 6658228362092147731L;
private final String className;
private final String methodName;
private String[] ptypes;
private String rtype;
private Object[] args;
private T data;
private RpcPayload(String className, String methodName, String[] ptypes, String rtype, Object[] args, T data) {
this.className = className;
this.methodName = methodName;
this.ptypes = ptypes;
this.rtype = rtype;
this.args = args;
this.data = data;
}
public static RpcPayload of(String className, String methodName, String[] ptypes, String rtype, Object[] args, T data) {
return new RpcPayload<>(className, methodName, ptypes, rtype, args, data);
}
public static RpcPayload of(String className, String methodName) {
return of(className, methodName, null, null, null, null);
}
public RpcPayload ptypes(String[] ptypes) {
this.ptypes = ptypes;
return this;
}
public RpcPayload rtype(String rtype) {
this.rtype = rtype;
return this;
}
public RpcPayload args(Object[] args) {
this.args = args;
return this;
}
public RpcPayload data(T data) {
this.data = data;
return this;
}
public String className() {
return className;
}
public String methodName() {
return methodName;
}
public String[] ptypes() {
return ptypes;
}
public String rtype() {
return rtype;
}
public Object[] args() {
return args;
}
public T data() {
return data;
}
@Override
public String toString() {
return "RpcPayload{" +
"className='" + className + '\'' +
", methodName='" + methodName + '\'' +
", ptypes=" + Arrays.toString(ptypes) +
", rtype='" + rtype + '\'' +
", args=" + Arrays.toString(args) +
", data=" + data +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy