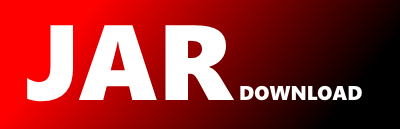
shz.net.netty.NettyDecoderHandler Maven / Gradle / Ivy
package shz.net.netty;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.handler.codec.ByteToMessageDecoder;
import shz.core.AccessibleCacheHelp;
import shz.core.Serializer;
import java.util.List;
public class NettyDecoderHandler extends ByteToMessageDecoder {
private final Class cls;
public NettyDecoderHandler() {
cls = AccessibleCacheHelp.getParameterizedType(getClass(), NettyDecoderHandler.class, "I");
}
@Override
protected void decode(ChannelHandlerContext ctx, ByteBuf in, List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy