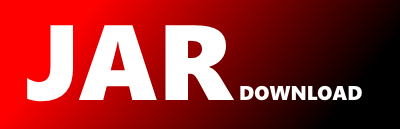
shz.net.udp.UdpServer Maven / Gradle / Ivy
package shz.net.udp;
import shz.core.ThreadHelp;
import java.util.Map;
import java.util.Objects;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ExecutorService;
public final class UdpServer {
private ExecutorService executor;
private volatile boolean init;
private final Map> handlers;
private static class UDPServerHolder {
private static final UdpServer instance = new UdpServer();
}
private UdpServer() {
handlers = new ConcurrentHashMap<>();
}
public static UdpServer getInstance() {
return UDPServerHolder.instance;
}
public void init(ExecutorService executor) {
if (init) return;
this.executor = executor == null
? ThreadHelp.getExecutor(ThreadHelp.TPConfig.of("UdpServerExecutor").tpType(ThreadHelp.TPType.FIXED_THREAD_POOL))
: executor;
init = true;
}
public void init() {
init(null);
}
public void register(UdpServerHandler, ?> handler) {
Objects.requireNonNull(handler);
handlers.put(handler.port, handler);
}
public void start(int port) {
UdpServerHandler, ?> handler = handlers.get(port);
Objects.requireNonNull(handler);
if (handler.stop) handler.stop = false;
executor.execute(handler);
}
public void start() {
handlers.forEach((k, v) -> {
if (v == null) return;
if (v.stop) v.stop = false;
executor.execute(v);
});
}
public void stop(int port) {
UdpServerHandler, ?> handler = handlers.get(port);
Objects.requireNonNull(handler);
handler.stop = true;
}
public void stop() {
handlers.forEach((k, v) -> {
if (v == null) return;
v.stop = true;
});
}
public void remove(int port) {
stop(port);
handlers.remove(port);
if (executor != null && handlers.size() == 0) executor.shutdown();
}
public void remove() {
stop();
handlers.clear();
if (executor != null) executor.shutdown();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy