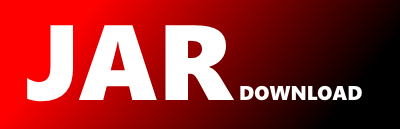
shz.PropHelp Maven / Gradle / Ivy
package shz;
import shz.cache.MapCache;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.Field;
import java.text.Collator;
import java.util.*;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.stream.Collectors;
@SuppressWarnings("unchecked")
public final class PropHelp {
private PropHelp() {
throw new IllegalStateException();
}
public static Properties getProp(InputStream is) {
Objects.requireNonNull(is);
Properties prop = new Properties();
try {
prop.load(is);
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(is);
}
Properties result = new Properties();
Enumeration> en = prop.propertyNames();
String key;
while (en.hasMoreElements()) {
key = (String) en.nextElement();
String val = prop.getProperty(key);
if (Validator.nonBlank(val)) result.setProperty(key, val);
}
return result;
}
static final class PropSetFieldStrategy extends ToObject.SetFieldStrategy {
@Override
public Function, Class>, Collection>>> supCollection() {
return t -> (u, r) -> {
String m = u.apply(t);
if (Validator.isBlank(m)) return Collections.emptySet();
return ToSet.collect(keys(t).stream().filter(Objects::nonNull).filter(k -> k.startsWith(m)).map(k -> executor(m, r)).filter(Objects::nonNull), true);
};
}
@Override
public Function, Class>[], Map, ?>>> supMap() {
return t -> (u, r) -> {
String m = u.apply(t);
if (Validator.isBlank(m)) return Collections.emptyMap();
return ToMap.collect(keys(t).stream().filter(Objects::nonNull).filter(k -> k.startsWith(m)), k -> executor(m, r[0]), k -> executor(m, r[1]), true);
};
}
@Override
public Function, Class>, Object>> supObject() {
return t -> (u, r) -> {
String m = u.apply(t);
if (Validator.isBlank(m)) return null;
return keys(t).stream().filter(Objects::nonNull).anyMatch(k -> k.startsWith(m)) ? executor(m, r) : null;
};
}
Map map;
@Override
protected Collection keys(Field field) {
return map.keySet();
}
@Override
protected Object executor(String key, Class> cls) {
return ToObject.fromMap(cls, map, f -> key + "." + f.getName(), Function.identity(), this);
}
T fromMap(Class extends T> cls, Map map) {
this.map = map;
return ToObject.fromMap(cls, map, Field::getName, Function.identity(), this);
}
T fromMap(T t, Map map) {
this.map = map;
return ToObject.fromMap(t, map, Field::getName, Function.identity(), this);
}
}
private static final PropSetFieldStrategy STRATEGY = new PropSetFieldStrategy();
public static T toObject(Class extends T> cls, Map map) {
return ((PropSetFieldStrategy) STRATEGY.clone()).fromMap(cls, map);
}
public static T toObject(T t, Map map) {
return ((PropSetFieldStrategy) STRATEGY.clone()).fromMap(t, map);
}
public static T toObject(Class extends T> cls, Properties prop) {
return toObject(cls, propToMap(prop));
}
private static Map propToMap(Properties prop) {
if (prop == null) return Collections.emptyMap();
Enumeration> en = prop.propertyNames();
String key;
Map map = new HashMap<>();
while (en.hasMoreElements()) {
key = (String) en.nextElement();
map.put(key, prop.getProperty(key));
}
return map;
}
public static T toObject(T t, Properties prop) {
return toObject(t, propToMap(prop));
}
public static List toObjects(Class extends T> cls, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy