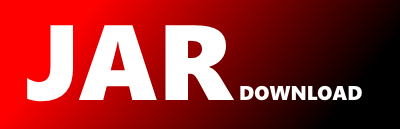
shz.TimeHelp Maven / Gradle / Ivy
package shz;
import java.sql.Timestamp;
import java.time.*;
import java.time.format.DateTimeFormatter;
import java.time.temporal.ChronoUnit;
import java.time.temporal.Temporal;
import java.util.Date;
import java.util.TimeZone;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public final class TimeHelp {
private TimeHelp() {
throw new IllegalStateException();
}
public static final ZoneId ZONE_ID = ZoneId.of("Asia/Shanghai");
public static LocalDateTime toLdt(Instant instant) {
if (instant == null) return null;
return LocalDateTime.ofInstant(instant, ZONE_ID);
}
public static LocalDateTime toLdt(ZonedDateTime zdt) {
if (zdt == null) return null;
return zdt.toLocalDateTime();
}
public static LocalDateTime toLdt(Date date) {
if (date == null) return null;
return LocalDateTime.ofInstant(Instant.ofEpochMilli(date.getTime()), ZONE_ID);
}
public static LocalDateTime toLdt(Long timestamp) {
if (timestamp == null) return null;
return LocalDateTime.ofInstant(Instant.ofEpochMilli(timestamp), ZONE_ID);
}
public static final DateTimeFormatter DATE_TIME_PATTERN = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
public static LocalDateTime toLdt(String s) {
if ((s = formatTimeString(s)) == null) return null;
return LocalDateTime.parse(s, DATE_TIME_PATTERN);
}
private static String formatTimeString(String s) {
String yyyy = find(s);
if (yyyy == null || yyyy.length() < 4) return null;
boolean flag = yyyy.length() == s.length();
if (flag) s = s.substring(4);
StringBuilder sb = new StringBuilder(19).append(flag ? yyyy.substring(0, 4) : yyyy);
for (int i = 0; i < 5; ++i) {
boolean appendTime;
if (flag) {
int length = s.length();
String value = null;
if (length > 2) {
value = s.substring(0, 2);
s = s.substring(2);
} else if (length == 2 || length == 1) {
value = s;
s = "";
}
appendTime = appendTime(sb, value, i);
} else {
s = s.replaceFirst("\\d+", "");
appendTime = appendTime(sb, find(s), i);
}
if (!appendTime) break;
}
return sb.toString();
}
private static String find(String s) {
if (s == null) return null;
Matcher matcher = Pattern.compile("\\d+", 0).matcher(s);
return matcher.find() ? matcher.group(0) : null;
}
private static boolean appendTime(StringBuilder sb, String value, int nest) {
if (value == null) {
switch (nest) {
case 0:
sb.append("-01-01 00:00:00");
break;
case 1:
sb.append("-01 00:00:00");
break;
case 2:
sb.append(" 00:00:00");
break;
case 3:
sb.append(":00:00");
break;
case 4:
sb.append(":00");
break;
}
return false;
}
switch (nest) {
case 0:
case 1:
sb.append("-");
break;
case 2:
sb.append(" ");
break;
case 3:
case 4:
sb.append(":");
break;
}
if (value.length() == 1) sb.append("0");
sb.append(value);
return true;
}
public static LocalDateTime toLdt(Object obj) {
if (obj == null) return null;
if (obj instanceof LocalDateTime) return (LocalDateTime) obj;
if (obj instanceof Instant) return toLdt((Instant) obj);
if (obj instanceof ZonedDateTime) return toLdt((ZonedDateTime) obj);
if (obj instanceof Date) return toLdt((Date) obj);
if (obj instanceof Number) return toLdt(((Number) obj).longValue());
if (obj instanceof CharSequence) return toLdt(((CharSequence) obj).toString());
return null;
}
public static final ZoneOffset ZONE_OFFSET = ZoneOffset.of("+8");
public static Instant toInstant(LocalDateTime ldt) {
if (ldt == null) return null;
return ldt.toInstant(ZONE_OFFSET);
}
public static Instant toInstant(ZonedDateTime zdt) {
if (zdt == null) return null;
return zdt.toInstant();
}
public static Instant toInstant(Date date) {
if (date == null) return null;
return Instant.ofEpochMilli(date.getTime());
}
public static Instant toInstant(String s) {
return toInstant(toLdt(s));
}
public static Instant toInstant(Long timestamp) {
if (timestamp == null) return null;
return Instant.ofEpochMilli(timestamp);
}
public static Instant toInstant(Object obj) {
return toInstant(toLdt(obj));
}
public static ZonedDateTime toZdt(LocalDateTime ldt) {
if (ldt == null) return null;
return ZonedDateTime.ofInstant(ldt.toInstant(ZONE_OFFSET), ZONE_ID);
}
public static ZonedDateTime toZdt(Instant instant) {
if (instant == null) return null;
return ZonedDateTime.ofInstant(instant, ZONE_ID);
}
public static ZonedDateTime toZdt(Date date) {
if (date == null) return null;
return ZonedDateTime.ofInstant(Instant.ofEpochMilli(date.getTime()), ZONE_ID);
}
public static ZonedDateTime toZdt(String s) {
return toZdt(toLdt(s));
}
public static ZonedDateTime toZdt(Long timestamp) {
return ZonedDateTime.ofInstant(Instant.ofEpochMilli(timestamp), ZONE_ID);
}
public static ZonedDateTime toZdt(Object obj) {
return toZdt(toLdt(obj));
}
public static Date toDate(LocalDateTime ldt) {
if (ldt == null) return null;
return Timestamp.valueOf(ldt);
}
public static Date toDate(Instant instant) {
if (instant == null) return null;
return Timestamp.from(instant);
}
public static Date toDate(ZonedDateTime zdt) {
if (zdt == null) return null;
return Timestamp.from(zdt.toInstant());
}
public static Date toDate(String s) {
return toDate(toLdt(s));
}
public static Date toDate(Long timestamp) {
if (timestamp == null) return null;
return new Date(timestamp);
}
public static Date toDate(Object obj) {
return toDate(toLdt(obj));
}
public static String format(LocalDateTime ldt, String pattern) {
return ldt.format(DateTimeFormatter.ofPattern(pattern));
}
public static String format(LocalDateTime ldt) {
return ldt.format(DATE_TIME_PATTERN);
}
public static String format(Instant instant, String pattern) {
return LocalDateTime.ofInstant(instant, ZONE_ID).format(DateTimeFormatter.ofPattern(pattern));
}
public static String format(Instant instant) {
return LocalDateTime.ofInstant(instant, ZONE_ID).format(DATE_TIME_PATTERN);
}
public static String format(Date date, String pattern) {
return LocalDateTime.ofInstant(Instant.ofEpochMilli(date.getTime()), ZONE_ID).format(DateTimeFormatter.ofPattern(pattern));
}
public static String format(Date date) {
return LocalDateTime.ofInstant(Instant.ofEpochMilli(date.getTime()), ZONE_ID).format(DATE_TIME_PATTERN);
}
public static String format(Object obj, String pattern) {
if (obj == null) return null;
if (obj instanceof LocalDateTime) return format((LocalDateTime) obj, pattern);
if (obj instanceof Instant) return format((Instant) obj, pattern);
if (obj instanceof Date) return format((Date) obj, pattern);
return null;
}
public static String format(Object obj) {
if (obj == null) return null;
if (obj instanceof LocalDateTime) return format((LocalDateTime) obj);
if (obj instanceof Instant) return format((Instant) obj);
if (obj instanceof Date) return format((Date) obj);
return null;
}
public static LocalDateTime exchange(LocalDateTime ldt, TimeZone src, TimeZone des) {
return LocalDateTime.ofInstant(exchange(ldt.toInstant(ZONE_OFFSET), src, des), ZONE_ID);
}
public static final TimeZone TIME_ZONE = TimeZone.getTimeZone("Asia/Shanghai");
public static LocalDateTime exchange(LocalDateTime ldt) {
return exchange(ldt, TimeZone.getDefault(), TIME_ZONE);
}
public static Instant exchange(Instant instant, TimeZone src, TimeZone des) {
return Instant.ofEpochMilli(instant.toEpochMilli() + des.getRawOffset() - src.getRawOffset());
}
public static Instant exchange(Instant instant) {
return exchange(instant, TimeZone.getDefault(), TIME_ZONE);
}
public static long between(ChronoUnit unit, Temporal t1, Temporal t2) {
return unit.between(t1, t2);
}
public static Duration between(Temporal t1, Temporal t2) {
return Duration.between(t1, t2);
}
public static Period between(LocalDate ld1, LocalDate ld2) {
return Period.between(ld1, ld2);
}
/**
* 获取指定时间凌晨时间
*/
public static LocalDateTime morning(LocalDateTime ldt) {
return LocalDateTime.of(ldt.toLocalDate(), LocalTime.MIDNIGHT);
}
/**
* 获取当日凌晨时间
*/
public static LocalDateTime morning() {
return LocalDateTime.of(LocalDate.now(), LocalTime.MIDNIGHT);
}
/**
* 获取指定时间凌晨时间less
*/
public static LocalDateTime morningLess(LocalDateTime ldt) {
return LocalDateTime.of(ldt.toLocalDate().minusDays(1), LocalTime.MAX);
}
/**
* 获取当日凌晨时间less
*/
public static LocalDateTime morningLess() {
return LocalDateTime.of(LocalDate.now().minusDays(1), LocalTime.MAX);
}
/**
* 获取指定时间凌晨时间more
*/
public static LocalDateTime morningMore(LocalDateTime ldt) {
return LocalDateTime.of(ldt.toLocalDate(), LocalTime.ofNanoOfDay(1));
}
/**
* 获取当日凌晨时间more
*/
public static LocalDateTime morningMore() {
return LocalDateTime.of(LocalDate.now(), LocalTime.ofNanoOfDay(1));
}
public static final DateTimeFormatter DATE_PATTERN = DateTimeFormatter.ofPattern("yyyy-MM-dd");
public static final DateTimeFormatter TIME_PATTERN = DateTimeFormatter.ofPattern("HH:mm:ss");
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy