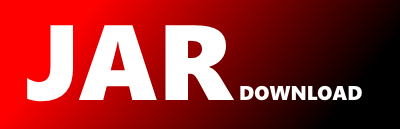
shz.cache.MapCache Maven / Gradle / Ivy
package shz.cache;
import java.lang.ref.Reference;
import java.lang.ref.ReferenceQueue;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
public final class MapCache extends LocalCache {
private final ConcurrentHashMap> cache;
public MapCache(int capacity, RefType refType, ReferenceQueue super V> queue) {
super(refType, queue);
cache = new ConcurrentHashMap<>(capacity);
}
public MapCache(int capacity, RefType refType) {
this(capacity, refType, null);
}
public MapCache(int capacity) {
this(capacity, RefType.SOFT);
}
@Override
public V get(K key) {
Reference ref = cache.get(key);
if (ref == null || ref.isEnqueued()) {
cache.remove(key);
return null;
}
return ref.get();
}
@Override
public void put(K key, V val) {
cache.put(key, getReference(val));
}
@Override
public boolean containsKey(K key) {
return cache.containsKey(key);
}
@Override
public int size() {
int size = 0;
for (Map.Entry> kv : cache.entrySet()) {
Reference ref = kv.getValue();
if (ref == null || ref.isEnqueued()) cache.remove(kv.getKey());
else ++size;
}
return size;
}
@Override
public void delete(K key) {
Reference ref = cache.get(key);
if (ref != null) ref.clear();
cache.remove(key);
}
@Override
public void clear() {
cache.forEach((key, ref) -> {
if (ref != null) ref.clear();
});
cache.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy