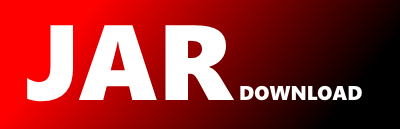
shz.st.bst.jxx.JJRedBlackBST Maven / Gradle / Ivy
package shz.st.bst.jxx;
/**
* 健为long类型,值为long类型的红黑树
*
* 8+56*n(n为元素个数)
*
* B=24+56*n
*/
public class JJRedBlackBST extends JXXRedBlackBST {
/**
* 8+29+对齐填充=40
*
* B=56
*/
protected static final class Node extends JXXRedBlackBST.Node {
public long val;
public Node(long key, long val, boolean red) {
super(key, red);
this.val = val;
}
public Node(long key, long val) {
this(key, val, true);
}
}
protected JJRedBlackBST(long key, long val) {
super(new Node(key, val, false));
}
public static JJRedBlackBST of(long key, long val) {
return new JJRedBlackBST(key, val);
}
public static JJRedBlackBST of(long key) {
return of(key, 0L);
}
public final void put(long key, long val) {
root = put(root(), key, val);
root.red = false;
}
protected final Node put(Node h, long key, long val) {
if (h == null) return new Node(key, val);
if (key < h.key) h.left = put(h.left(), key, val);
else if (key > h.key) h.right = put(h.right(), key, val);
else h.val = val;
//如果右子结点是红色的而左子结点是黑色的,进行左旋转
if (isRed(h.right) && !isRed(h.left)) h = rotateLeft(h);
//如果左子结点是红色的且它的左子结点也是红色的,进行右旋转
if (isRed(h.left) && isRed(h.left.left)) h = rotateRight(h);
//如果左右子结点均为红色,进行颜色转换
if (isRed(h.left) && isRed(h.right)) flipColors(h);
h.size = size(h.left) + size(h.right) + 1;
return h;
}
public final Long get(long key) {
Node h = get(root(), key);
return h == null ? null : h.val;
}
protected final Node get(Node h, long key) {
while (h != null) {
if (key == h.key) break;
h = key < h.key ? h.left() : h.right();
}
return h;
}
public final void delete(long key) {
if (root == null) return;
if (!isRed(root.left) && !isRed(root.right)) root.red = true;
root = delete(root(), key);
if (root == null) return;
if (!isEmpty()) root.red = false;
}
protected final Node delete(Node h, long key) {
if (key < h.key) {
if (!isRed(h.left) && !isRed(h.left.left)) h = moveRedLeft(h);
h.left = delete(h.left(), key);
} else {
if (isRed(h.left)) h = rotateRight(h);
if (key == h.key && h.right == null) return null;
if (!isRed(h.right) && !isRed(h.right.left)) h = moveRedRight(h);
if (key == h.key) {
Node min = min(h.right);
h.val = get(h.right(), min.key).val;
h.key = min.key;
h.right = deleteMin(h.right);
} else h.right = delete(h.right(), key);
}
return balance(h);
}
}