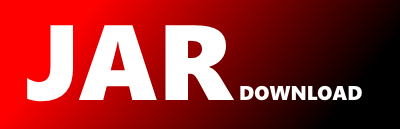
io.github.shiruka.fragment.Fragment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fragment Show documentation
Show all versions of fragment Show documentation
A joint project that helps developers to make fragments for Shiru ka.
/*
* MIT License
*
* Copyright (c) 2020 Shiru ka
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*
*/
package io.github.shiruka.fragment;
import io.github.shiruka.conf.Config;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.Files;
import java.nio.file.StandardCopyOption;
import java.util.jar.JarFile;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.simpleyaml.configuration.file.FileConfiguration;
/**
* the main class of all the fragments.
*/
public abstract class Fragment {
/**
* The fragment's class loader
*/
@NotNull
private final FragmentClassLoader fragmentClassLoader;
/**
* The fragment's config.
*/
@Nullable
private Config config;
/**
* The fragment's data folder.
*/
@Nullable
private File dataFolder;
/**
* ctor.
*/
protected Fragment() {
this.fragmentClassLoader = (FragmentClassLoader) this.getClass().getClassLoader();
}
/**
* gets the fragment's description.
*
* @return the description.
*/
@NotNull
public final FragmentDescription getDescription() {
return this.fragmentClassLoader.getFragmentDescription();
}
/**
* gets the config.
*
* @return the config.
*/
@NotNull
public final FileConfiguration getConfig() {
return this.getFragmentConfig().getConfiguration();
}
/**
* get the fragment manager.
*
* @return the fragment manager.
*/
@NotNull
public final FragmentManager getFragmentManager() {
return this.fragmentClassLoader.getFragmentManager();
}
/**
* gets the {@link Config} of the fragment.
*
* @return the {@link Config}.
*/
@NotNull
public final Config getFragmentConfig() {
if (this.config == null) {
this.setupConfig();
}
return this.config;
}
/**
* setups the config
*/
public final void setupConfig() {
this.config = this.createConfig();
}
/**
* reloads the config.
*/
public final void reloadConfig() {
this.getFragmentConfig().reload();
}
/**
* saves the config.
*/
public final void saveConfig() {
this.getFragmentConfig().save();
}
/**
* gets the fragment's folder.
*
* @return the directory for the fragment.
*/
@NotNull
public final File getDataFolder() {
if (this.dataFolder == null) {
this.dataFolder = new File(this.getFragmentManager().getFragmentsDir(), this.getDescription().getName());
}
if (!this.dataFolder.exists()) {
this.dataFolder.mkdirs();
}
return this.dataFolder;
}
/**
* copies the resource from the fragment's jar.
*
* @param path path to resource.
* @param replace whether it replaces the existed one.
*/
public final void saveResource(@NotNull final String path, final boolean replace) {
if (path.isEmpty()) {
throw new IllegalArgumentException("Path cannot be empty!");
}
final var newPath = path.replace('\\', '/');
try (final var jar = new JarFile(this.fragmentClassLoader.getFile())) {
final var jarConfig = jar.getJarEntry(newPath);
if (jarConfig != null) {
try (final var in = jar.getInputStream(jarConfig)) {
if (in == null) {
throw new IllegalArgumentException(
"The embedded resource '" + newPath + "' cannot be found");
}
final var out = new File(this.getDataFolder(), newPath);
out.getParentFile().mkdirs();
if (!out.exists() || replace) {
Files.copy(in, out.toPath(), StandardCopyOption.REPLACE_EXISTING);
}
}
} else {
throw new IllegalArgumentException("The embedded resource '" + newPath + "' cannot be found");
}
} catch (final IOException e) {
this.getFragmentManager().getLogger().warn("Could not load from jar file. " + newPath);
}
}
/**
* gets the resource from the fragment's jar.
*
* @param path path to resource.
*
* @return the InputStream of the resource, or null if it's not found.
*/
@Nullable
public final InputStream getResource(@NotNull final String path) {
if (path.isEmpty()) {
throw new IllegalArgumentException("Path cannot be empty!");
}
final var newPath = path.replace('\\', '/');
try (final var jar = new JarFile(this.fragmentClassLoader.getFile())) {
final var jarConfig = jar.getJarEntry(newPath);
if (jarConfig != null) {
try (final var in = jar.getInputStream(jarConfig)) {
return in;
}
}
} catch (final IOException e) {
this.getFragmentManager().getLogger().warn("Could not load from jar file. " + newPath);
}
return null;
}
/**
* gets the class loader.
*
* @return the class loader.
*/
@NotNull
protected final FragmentClassLoader getClassLoader() {
return this.fragmentClassLoader;
}
/**
* checks if the fragment pass the load.
*
* @return {@code true} if the fragment pass the load.
*/
protected boolean preLoad() {
return true;
}
/**
* checks if the fragment pass the enable.
*
* @return {@code true} if the fragment pass the enable.
*/
protected boolean preEnable() {
return true;
}
/**
* checks if the fragment pass the disable.
*
* @return {@code true} if the fragment pass the disable.
*/
protected boolean preDisable() {
return true;
}
/**
* checks if the fragment pass the reload.
*
* @return {@code true} if the fragment pass the reload.
*/
protected boolean preReload() {
return true;
}
/**
* creates the config.
*/
@NotNull
protected abstract Config createConfig();
/**
* runs when the fragment loads.
*/
protected abstract void postLoad();
/**
* runs when the fragment enables.
*/
protected abstract void postEnable();
/**
* runs when the fragment disables.
*/
protected abstract void postDisable();
/**
* runs when the fragment reloads.
*/
protected abstract void postReload();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy