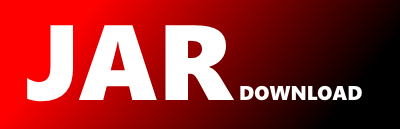
com.taotao.boot.monitor.collect.task.NacosCollectTask Maven / Gradle / Ivy
/*
* Copyright (c) 2020-2030, Shuigedeng ([email protected] & https://blog.taotaocloud.top/).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.taotao.boot.monitor.collect.task;
import com.alibaba.nacos.api.naming.NamingService;
import com.alibaba.nacos.api.naming.pojo.Instance;
import com.alibaba.nacos.api.naming.pojo.ListView;
import com.alibaba.nacos.api.naming.pojo.ServiceInfo;
import com.alibaba.nacos.client.naming.NacosNamingService;
import com.taotao.boot.common.constant.CommonConstant;
import com.taotao.boot.common.utils.common.PropertyUtils;
import com.taotao.boot.common.utils.context.ContextUtils;
import com.taotao.boot.common.utils.log.LogUtils;
import com.taotao.boot.common.utils.reflect.ClassUtils;
import com.taotao.boot.common.utils.reflect.ReflectionUtils;
import com.taotao.boot.core.support.Collector;
import com.taotao.boot.monitor.annotation.FieldReport;
import com.taotao.boot.monitor.collect.AbstractCollectTask;
import com.taotao.boot.monitor.collect.CollectInfo;
import com.taotao.boot.monitor.properties.CollectTaskProperties;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/**
* nacos 客户端性能采集
*/
public class NacosCollectTask extends AbstractCollectTask {
private static final String TASK_NAME = "ttc.monitor.collect.nacos";
private final CollectTaskProperties collectTaskProperties;
private final boolean classExist;
public NacosCollectTask(CollectTaskProperties collectTaskProperties) {
this.collectTaskProperties = collectTaskProperties;
this.classExist = ClassUtils.isExist("com.alibaba.cloud.nacos.NacosServiceManager");
}
@Override
public int getTimeSpan() {
return collectTaskProperties.getNacosTimeSpan();
}
@Override
public String getDesc() {
return this.getClass().getName();
}
@Override
public String getName() {
return TASK_NAME;
}
@Override
public boolean getEnabled() {
return collectTaskProperties.isNacosEnabled() && classExist;
}
@Override
protected CollectInfo getData() {
try {
Collector collector = Collector.getCollector();
Object nacosServiceManager = ContextUtils.getBean("com.alibaba.cloud.nacos.NacosServiceManager", false);
if (Objects.nonNull(collector) && Objects.nonNull(nacosServiceManager)) {
NacosClientInfo info = new NacosClientInfo();
try {
NamingService namingService = ReflectionUtils.getFieldValue(nacosServiceManager, "namingService");
NacosNamingService nacosNamingService = (NacosNamingService) namingService;
info.namespace = ReflectionUtils.getFieldValue(nacosNamingService, "namespace");
// info.endpoint = ReflectionUtils.getFieldValue(nacosNamingService, "endpoint");
// info.serverList = ReflectionUtils.getFieldValue(nacosNamingService,
// "serverList");
// info.cacheDir = ReflectionUtils.getFieldValue(nacosNamingService, "cacheDir");
info.logName = ReflectionUtils.getFieldValue(nacosNamingService, "logName");
info.instances = nacosNamingService.getAllInstances(
PropertyUtils.getProperty(CommonConstant.SPRING_APP_NAME_KEY),
CommonConstant.SPRING_APP_NAME_KEY);
info.servicesOfServer = nacosNamingService.getServicesOfServer(0, Integer.MAX_VALUE);
info.subscribeServices = nacosNamingService.getSubscribeServices();
info.serverStatus = nacosNamingService.getServerStatus();
// HostReactor hostReactor = ReflectionUtil.getFieldValue(nacosNamingService,
// "hostReactor");
// NamingProxy serverProxy = ReflectionUtil.getFieldValue(nacosNamingService,
// "serverProxy");
// BeatReactor beatReactor = nacosNamingService.getBeatReactor();
//
// info.serviceInfoMap = hostReactor.getServiceInfoMap();
} catch (Exception ignored) {
}
return info;
}
} catch (Exception e) {
if (LogUtils.isErrorEnabled()) {
LogUtils.error(e);
}
}
return null;
}
private static class NacosClientInfo implements CollectInfo {
@FieldReport(name = TASK_NAME + ".namespace", desc = "nacos namespace")
private String namespace = "";
@FieldReport(name = TASK_NAME + ".endpoint", desc = "nacos endpoint")
private String endpoint = "";
@FieldReport(name = TASK_NAME + ".serverList", desc = "nacos serverList")
private String serverList = "";
@FieldReport(name = TASK_NAME + ".cacheDir", desc = "nacos cacheDir")
private String cacheDir = "";
@FieldReport(name = TASK_NAME + ".logName", desc = "nacos logName")
private String logName = "";
@FieldReport(name = TASK_NAME + ".serverStatus", desc = "nacos serverStatus")
private String serverStatus = "";
@FieldReport(name = TASK_NAME + ".instances", desc = "nacos instances")
private List instances;
@FieldReport(name = TASK_NAME + ".serviceInfoMap", desc = "nacos serviceInfoMap")
private Map serviceInfoMap;
@FieldReport(name = TASK_NAME + ".servicesOfServer", desc = "nacos servicesOfServer")
private ListView servicesOfServer;
@FieldReport(name = TASK_NAME + ".subscribeServices", desc = "nacos subscribeServices")
private List subscribeServices;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy