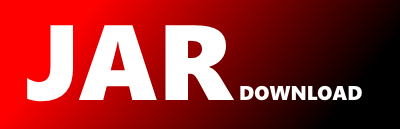
com.taotao.boot.monitor.collect.task.RocketMQCollectTask Maven / Gradle / Ivy
/*
* Copyright (c) 2020-2030, Shuigedeng ([email protected] & https://blog.taotaocloud.top/).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.taotao.boot.monitor.collect.task;
import com.taotao.boot.common.exception.BaseException;
import com.taotao.boot.common.utils.context.ContextUtils;
import com.taotao.boot.common.utils.reflect.ClassUtils;
import com.taotao.boot.common.utils.reflect.ReflectionUtils;
import com.taotao.boot.core.support.Collector;
import com.taotao.boot.monitor.annotation.FieldReport;
import com.taotao.boot.monitor.collect.AbstractCollectTask;
import com.taotao.boot.monitor.collect.CollectInfo;
import com.taotao.boot.monitor.properties.CollectTaskProperties;
import java.lang.management.ManagementFactory;
import java.lang.management.ThreadMXBean;
import java.util.HashMap;
public class RocketMQCollectTask extends AbstractCollectTask {
private static final String TASK_NAME = "ttc.monitor.collect.rocket";
private final ThreadMXBean threadMXBean;
private final CollectTaskProperties properties;
private HashMap lastThreadUserTime = new HashMap<>();
private final boolean classExist;
public RocketMQCollectTask(CollectTaskProperties properties) {
threadMXBean = ManagementFactory.getThreadMXBean();
this.properties = properties;
this.classExist = ClassUtils.isExist("com.taotao.boot.monitor.rocketmq.RocketMQConsumerProvider");
}
@Override
public int getTimeSpan() {
return properties.getRocketMQTimeSpan();
}
@Override
public boolean getEnabled() {
return properties.isRocketMQEnabled() && classExist;
}
@Override
public String getDesc() {
return this.getClass().getName();
}
@Override
public String getName() {
return TASK_NAME;
}
@Override
protected CollectInfo getData() {
RocketMQInfo data = new RocketMQInfo();
if (ContextUtils.getBean(
ReflectionUtils.tryClassForName("com.taotao.boot.monitor.rocketmq.RocketMQConsumerProvider"),
false)
!= null) {
Collector.Hook hook = getCollectorHook("com.taotao.boot.monitor.rocketmq.RocketMQMonitor");
data.consumerHookCurrent = hook.getCurrent();
data.consumerHookError = hook.getLastErrorPerSecond();
data.consumerHookSuccess = hook.getLastSuccessPerSecond();
data.consumerHookList = hook.getMaxTimeSpanList().toText();
data.consumerHookListPerMinute = hook.getMaxTimeSpanListPerMinute().toText();
}
if (ContextUtils.getBean(
ReflectionUtils.tryClassForName("com.taotao.boot.monitor.rocketmq.RocketMQProducerProvider"),
false)
!= null) {
Collector.Hook hook = getCollectorHook("com.taotao.boot.monitor.rocketmq.RocketMQMonitor");
data.producerHookCurrent = hook.getCurrent();
data.producerHookError = hook.getLastErrorPerSecond();
data.producerHookSuccess = hook.getLastSuccessPerSecond();
data.producerHookList = hook.getMaxTimeSpanList().toText();
data.producerHookListPerMinute = hook.getMaxTimeSpanListPerMinute().toText();
}
return data;
}
private Collector.Hook getCollectorHook(String className) {
Class> monitor = ReflectionUtils.classForName(className);
try {
return (Collector.Hook) ReflectionUtils.findMethod(monitor, "hook").invoke(null);
} catch (Exception e) {
throw new BaseException(e);
}
}
private static class RocketMQInfo implements CollectInfo {
@FieldReport(name = "rocketmq.consumer.hook.error", desc = "Consumer拦截上一次每秒出错次数")
private Long consumerHookError;
@FieldReport(name = "rocketmq.consumer.hook.success", desc = "Consumer拦截上一次每秒成功次数")
private Long consumerHookSuccess;
@FieldReport(name = "rocketmq.consumer.hook.current", desc = "Consumer拦截当前执行任务数")
private Long consumerHookCurrent;
@FieldReport(name = "rocketmq.consumer.hook.list.detail", desc = "Consumer拦截历史最大耗时任务列表")
private String consumerHookList;
@FieldReport(name = "rocketmq.consumer.hook.list.minute.detail", desc = "Consumer拦截历史最大耗时任务列表(每分钟)")
private String consumerHookListPerMinute;
@FieldReport(name = "rocketmq.producer.hook.error", desc = "Producer拦截上一次每秒出错次数")
private Long producerHookError;
@FieldReport(name = "rocketmq.producer.hook.success", desc = "Producer拦截上一次每秒成功次数")
private Long producerHookSuccess;
@FieldReport(name = "rocketmq.producer.hook.current", desc = "Producer拦截当前执行任务数")
private Long producerHookCurrent;
@FieldReport(name = "rocketmq.producer.hook.list.detail", desc = "Producer拦截历史最大耗时任务列表")
private String producerHookList;
@FieldReport(name = "rocketmq.producer.hook.list.minute.detail", desc = "Producer拦截历史最大耗时任务列表(每分钟)")
private String producerHookListPerMinute;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy