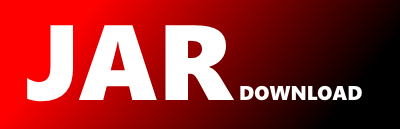
com.taotao.boot.monitor.utils.ExceptionUtils Maven / Gradle / Ivy
/*
* Copyright (c) 2020-2030, Shuigedeng ([email protected] & https://blog.taotaocloud.top/).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.taotao.boot.monitor.utils;
import com.taotao.boot.common.constant.CommonConstant;
import com.taotao.boot.common.utils.common.PropertyUtils;
import com.taotao.boot.common.utils.context.ContextUtils;
import com.taotao.boot.common.utils.servlet.RequestUtils;
import com.taotao.boot.core.enums.ExceptionTypeEnum;
import com.taotao.boot.core.http.DefaultHttpClient;
import com.taotao.boot.core.http.HttpClient;
import com.taotao.boot.monitor.Monitor;
import com.taotao.boot.monitor.enums.WarnLevelEnum;
import com.taotao.boot.monitor.enums.WarnTypeEnum;
import com.taotao.boot.monitor.model.Message;
import org.apache.commons.lang3.StringUtils;
import org.apache.hc.core5.http.ContentType;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.concurrent.atomic.AtomicReference;
/**
* ExceptionUtils
*
* @author shuigedeng
* @version 2021.9
* @since 2021-09-10 16:35:39
*/
public class ExceptionUtils {
private static final String exceptionUrl = "taotao.boot.monitor.report.exception.url";
/**
* 上报异常
*
* @param message message
* @param applicationName applictionName
* @since 2021-09-10 16:35:51
*/
public static void reportException(Message message, String applicationName) {
if (message.getWarnType() == WarnTypeEnum.ERROR) {
AtomicReference title = new AtomicReference<>(message.getTitle());
Monitor monitorThreadPool = ContextUtils.getBean(Monitor.class, false);
if (Objects.nonNull(monitorThreadPool)) {
monitorThreadPool.monitorSubmit("系统任务: reportException 异常上报", () -> {
Map param = new HashMap<>();
param.put("exceptionTitle", title.get());
param.put("exceptionType", message.getExceptionType().getCode());
param.put("exceptionLevel", message.getLevelType().getLevel());
if (StringUtils.isNotBlank(message.getExceptionCode())) {
param.put("exceptionCode", message.getExceptionCode());
}
if (StringUtils.isNotBlank(message.getBizScope())) {
param.put("bizScope", message.getBizScope());
}
param.put(
"exceptionContent",
String.format(
"[%s][%s][%s]%s",
RequestUtils.getIpAddress(),
PropertyUtils.getProperty(CommonConstant.SPRING_APP_NAME_KEY),
PropertyUtils.getProperty(CommonConstant.SPRING_APP_NAME_KEY),
message.getContent()));
if (StringUtils.isNotBlank(applicationName)) {
param.put("applicationName", applicationName);
} else {
param.put("applicationName", PropertyUtils.getProperty(CommonConstant.SPRING_APP_NAME_KEY));
}
HttpClient.Params params = HttpClient.Params.custom()
.setContentType(ContentType.APPLICATION_JSON)
.add(param)
.build();
DefaultHttpClient defaultHttpClient = ContextUtils.getBean(DefaultHttpClient.class, false);
if (Objects.nonNull(defaultHttpClient)) {
defaultHttpClient.post(PropertyUtils.getPropertyCache(exceptionUrl, StringUtils.EMPTY), params);
}
});
}
}
}
/**
* 上报异常
*
* @param message message
* @since 2021-09-10 16:36:04
*/
public static void reportException(Message message) {
reportException(message, null);
}
/**
* 上报异常
*
* @param warnLevelEnum warnLevelEnum
* @param title title
* @param content content
* @since 2021-09-10 16:36:09
*/
public static void reportException(WarnLevelEnum warnLevelEnum, String title, String content) {
reportException(
new Message(WarnTypeEnum.ERROR, title, content, warnLevelEnum, ExceptionTypeEnum.BE, null, null), null);
}
/**
* 上报异常
*
* @param warnLevelEnumType levelEnumType
* @param title title
* @param content content
* @param applicationName applicationName
* @since 2021-09-10 16:36:15
*/
public static void reportException(
WarnLevelEnum warnLevelEnumType, String title, String content, String applicationName) {
reportException(
new Message(WarnTypeEnum.ERROR, title, content, warnLevelEnumType, ExceptionTypeEnum.BE, null, null),
applicationName);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy