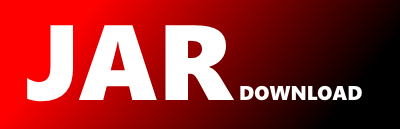
com.taotao.boot.sms.tencentv3.TencentV3SendHandler Maven / Gradle / Ivy
/*
* Copyright (c) 2020-2030, Shuigedeng ([email protected] & https://blog.taotaocloud.top/).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.taotao.boot.sms.tencentv3;
import com.taotao.boot.common.utils.log.LogUtils;
import com.taotao.boot.sms.common.exception.SendFailedException;
import com.taotao.boot.sms.common.handler.AbstractSendHandler;
import com.taotao.boot.sms.common.model.NoticeData;
import com.tencentcloudapi.common.Credential;
import com.tencentcloudapi.sms.v20190711.SmsClient;
import com.tencentcloudapi.sms.v20190711.models.SendSmsRequest;
import com.tencentcloudapi.sms.v20190711.models.SendSmsResponse;
import com.tencentcloudapi.sms.v20190711.models.SendStatus;
import org.springframework.context.ApplicationEventPublisher;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Map;
/**
* 腾讯云发送处理
*
* @author shuigedeng
* @version 2022.04
* @since 2022-04-27 17:52:00
*/
public class TencentV3SendHandler extends AbstractSendHandler {
private static final String SUCCESS_CODE = "OK";
private final SmsClient sender;
public TencentV3SendHandler(TencentV3Properties properties, ApplicationEventPublisher eventPublisher) {
super(properties, eventPublisher);
Credential credential = new Credential(properties.getSecretId(), properties.getSecretKey());
sender = new SmsClient(credential, properties.getRegion());
}
public static List> split(Collection collection, int size) {
final List> result = new ArrayList<>();
if (collection == null || collection.isEmpty()) {
return result;
}
ArrayList subList = new ArrayList<>(size);
for (T t : collection) {
if (subList.size() >= size) {
result.add(subList);
subList = new ArrayList<>(size);
}
subList.add(t);
}
result.add(subList);
return result;
}
@Override
public String getChannelName() {
return "qCloudV3";
}
@Override
public boolean send(NoticeData noticeData, Collection phones) {
String type = noticeData.getType();
String templateId = properties.getTemplates(type);
if (templateId == null) {
LogUtils.debug("templateId invalid");
publishSendFailEvent(noticeData, phones, new SendFailedException("templateId invalid"), null);
return false;
}
List paramsOrder = properties.getParamsOrder(type);
ArrayList params = new ArrayList<>();
if (!paramsOrder.isEmpty()) {
Map paramMap = noticeData.getParams();
for (String paramName : paramsOrder) {
String paramValue = paramMap.get(paramName);
params.add(paramValue);
}
}
return split(phones, 200).parallelStream()
.allMatch(subPhones -> send0(noticeData, templateId, params, subPhones));
}
private boolean send0(
NoticeData noticeData, String templateId, ArrayList params, Collection phones) {
SendSmsResponse result = null;
try {
SendSmsRequest request = new SendSmsRequest();
request.setSmsSdkAppid(properties.getSmsAppId());
request.setSign(properties.getSmsSign());
request.setTemplateID(templateId);
request.setTemplateParamSet(params.toArray(new String[0]));
request.setPhoneNumberSet(phones.toArray(new String[0]));
result = sender.SendSms(request);
if (result.getSendStatusSet() == null) {
return false;
}
ArrayList success = new ArrayList<>(result.getSendStatusSet().length);
String phone;
String code;
String message;
for (SendStatus sendStatus : result.getSendStatusSet()) {
phone = sendStatus.getPhoneNumber();
code = sendStatus.getCode();
if (SUCCESS_CODE.equals(code)) {
success.add(phone);
} else {
message = sendStatus.getMessage();
LogUtils.debug("send fail[phone={}, code={}, errMsg={}]", phone, code, message);
publishSendFailEvent(
noticeData, Collections.singleton(phone), new SendFailedException(message), result);
}
}
if (!success.isEmpty()) {
publishSendSuccessEvent(noticeData, success, result);
}
return !success.isEmpty();
} catch (Exception e) {
LogUtils.debug(e.getMessage(), e);
publishSendFailEvent(noticeData, phones, e, result);
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy