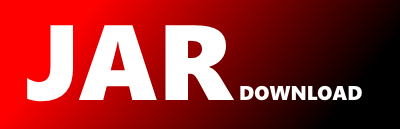
com.taotao.cloud.openapi.configuration.OpenapiAutoConfiguration Maven / Gradle / Ivy
/*
* Copyright 2002-2021 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.taotao.cloud.openapi.configuration;
import com.taotao.cloud.common.constant.StarterNameConstant;
import com.taotao.cloud.common.utils.LogUtil;
import com.taotao.cloud.core.utils.PropertyUtil;
import io.swagger.v3.oas.models.Components;
import io.swagger.v3.oas.models.ExternalDocumentation;
import io.swagger.v3.oas.models.OpenAPI;
import io.swagger.v3.oas.models.Paths;
import io.swagger.v3.oas.models.headers.Header;
import io.swagger.v3.oas.models.info.Contact;
import io.swagger.v3.oas.models.info.Info;
import io.swagger.v3.oas.models.info.License;
import io.swagger.v3.oas.models.media.IntegerSchema;
import io.swagger.v3.oas.models.media.StringSchema;
import io.swagger.v3.oas.models.security.SecurityScheme;
import io.swagger.v3.oas.models.security.SecurityScheme.In;
import io.swagger.v3.oas.models.servers.Server;
import java.util.ArrayList;
import java.util.List;
import com.taotao.cloud.core.properties.CoreProperties;
import org.springdoc.core.GroupedOpenApi;
import org.springdoc.core.customizers.OpenApiCustomiser;
import org.springframework.beans.factory.BeanFactory;
import org.springframework.beans.factory.BeanFactoryAware;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.context.EnvironmentAware;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.env.Environment;
import org.springframework.http.HttpHeaders;
/**
* SwaggerAutoConfiguration
*
* @author shuigedeng
* @version 1.0.0
* @since 2020/4/30 10:10
*/
@Configuration
public class OpenapiAutoConfiguration implements BeanFactoryAware, InitializingBean,
EnvironmentAware {
private static final String AUTH_KEY = "Authorization";
private BeanFactory beanFactory;
private Environment environment;
@Override
public void setBeanFactory(BeanFactory beanFactory) {
this.beanFactory = beanFactory;
}
@Override
public void setEnvironment(Environment environment) {
this.environment = environment;
}
@Override
public void afterPropertiesSet() throws Exception {
LogUtil.started(OpenapiAutoConfiguration.class, StarterNameConstant.OPENAPI_STARTER);
}
@Bean
public GroupedOpenApi groupedOpenApi() {
LogUtil.started(GroupedOpenApi.class, StarterNameConstant.OPENAPI_STARTER);
String applicationName = environment.getProperty(CoreProperties.SpringApplicationName, "");
return GroupedOpenApi
.builder()
.group(applicationName)
.pathsToMatch("/**")
.build();
}
@Bean
public OpenApiCustomiser consumerTypeHeaderOpenAPICustomiser() {
LogUtil.started(OpenApiCustomiser.class, StarterNameConstant.OPENAPI_STARTER);
String applicationName = environment.getProperty(CoreProperties.SpringApplicationName, "");
String[] split = applicationName.split("-");
String name = split[split.length - 1];
return openApi -> {
final Paths paths = openApi.getPaths();
String taotaoCloudVersion = PropertyUtil.getProperty("taotaoCloudVersion");
Paths newPaths = new Paths();
paths.keySet()
.forEach(e -> newPaths.put("/api/v" + taotaoCloudVersion + "/" + name + e,
paths.get(e)));
openApi.setPaths(newPaths);
openApi.getPaths().values().stream()
.flatMap(pathItem -> pathItem.readOperations().stream())
.forEach(operation -> {
});
};
}
@Bean
public OpenAPI openApi() {
LogUtil.started(OpenAPI.class, StarterNameConstant.OPENAPI_STARTER);
Components components = new Components();
// 添加auth认证header
components.addSecuritySchemes("token",
new SecurityScheme()
.description("token")
.type(SecurityScheme.Type.HTTP)
.name("token")
.in(In.HEADER)
.scheme("basic")
);
components.addSecuritySchemes("bearer",
new SecurityScheme()
.name(HttpHeaders.AUTHORIZATION)
.type(SecurityScheme.Type.HTTP)
.in(In.HEADER)
.scheme("bearer")
.bearerFormat("JWT")
);
// 添加全局header
components.addHeaders("taotao-cloud-request-version-header",
new Header()
.description("版本号")
.schema(new StringSchema())
);
components.addHeaders("taotao-cloud-request-weight-header",
new Header()
.description("权重")
.schema(new IntegerSchema())
);
String applicationName = environment.getProperty("spring.application.name", "");
String taotaoCloudVersion = environment.getProperty("taotaoCloudVersion");
String ip = environment.getProperty("spring.cloud.client.ip-address",
"0.0.0.0");
List servers = new ArrayList<>();
Server s1 = new Server();
s1.setUrl("http://" + ip + ":9999/");
s1.setDescription("本地地址");
servers.add(s1);
Server s2 = new Server();
s2.setUrl("http://dev.taotaocloud.top/");
s2.setDescription("测试环境地址");
servers.add(s2);
Server s3 = new Server();
s3.setUrl("http://pre.taotaocloud.top/");
s3.setDescription("预上线环境地址");
servers.add(s3);
Server s4 = new Server();
s4.setUrl("http://pro.taotaocloud.top/");
s4.setDescription("生产环境地址");
servers.add(s4);
Info info = new Info()
.title(applicationName.toUpperCase() + " API")
.description("TAOTAO CLOUD 电商及大数据平台")
.version(taotaoCloudVersion)
.contact(new Contact()
.name("dengtao")
.email("[email protected]")
.url("https://github.com/shuigedeng/taotao-cloud-project")
)
.termsOfService(applicationName)
.license(new License()
.name("Apache 2.0")
.url(
"https://github.com/shuigedeng/taotao-cloud-project/blob/master/LICENSE.txt")
);
ExternalDocumentation externalDocumentation = new ExternalDocumentation()
.description("TaoTao Cloud Wiki Documentation")
.url("https://github.com/shuigedeng/taotao-cloud-project/wiki");
return new OpenAPI()
.components(components)
.openapi("3.0.1")
.info(info)
.servers(servers)
.externalDocs(externalDocumentation);
}
// @Bean
// @ConditionalOnMissingBean
// @ConditionalOnProperty(name = "taotao.cloud.springdoc.enabled", matchIfMissing = true)
// public List createRestApi(SpringdocProperties springdocProperties) {
// ConfigurableBeanFactory configurableBeanFactory = (ConfigurableBeanFactory) beanFactory;
// List docketList = new LinkedList<>();
//
// // 没有分组
// if (springdocProperties.getDocket().size() == 0) {
// final Docket docket = createDocket(springdocProperties);
// configurableBeanFactory.registerSingleton("defaultDocket", docket);
// docketList.add(docket);
// return docketList;
// }
//
// // 分组创建
// for (String groupName : springdocProperties.getDocket().keySet()) {
// SpringdocProperties.DocketInfo docketInfo = springdocProperties.getDocket()
// .get(groupName);
//
// ApiInfo apiInfo = new ApiInfoBuilder()
// .title(docketInfo.getTitle().isEmpty() ? springdocProperties.getTitle()
// : docketInfo.getTitle())
// .description(
// docketInfo.getDescription().isEmpty() ? springdocProperties.getDescription()
// : docketInfo.getDescription())
// .version(docketInfo.getVersion().isEmpty() ? springdocProperties.getVersion()
// : docketInfo.getVersion())
// .license(docketInfo.getLicense().isEmpty() ? springdocProperties.getLicense()
// : docketInfo.getLicense())
// .licenseUrl(
// docketInfo.getLicenseUrl().isEmpty() ? springdocProperties.getLicenseUrl()
// : docketInfo.getLicenseUrl())
// .contact(
// new Contact(
// docketInfo.getContact().getName().isEmpty() ? springdocProperties
// .getContact().getName() : docketInfo.getContact().getName(),
// docketInfo.getContact().getUrl().isEmpty() ? springdocProperties
// .getContact()
// .getUrl() : docketInfo.getContact().getUrl(),
// docketInfo.getContact().getEmail().isEmpty() ? springdocProperties
// .getContact().getEmail() : docketInfo.getContact().getEmail()
// )
// )
// .termsOfServiceUrl(docketInfo.getTermsOfServiceUrl().isEmpty() ? springdocProperties
// .getTermsOfServiceUrl() : docketInfo.getTermsOfServiceUrl())
// .build();
//
// // base-path处理
// // 当没有配置任何path的时候,解析/**
// if (docketInfo.getBasePath().isEmpty()) {
// docketInfo.getBasePath().add("/**");
// }
//
// List> basePath = new ArrayList<>(docketInfo.getBasePath().size());
// for (String path : docketInfo.getBasePath()) {
// basePath.add(PathSelectors.ant(path));
// }
//
// // exclude-path处理
// List> excludePath = new ArrayList<>(
// docketInfo.getExcludePath().size());
// for (String path : docketInfo.getExcludePath()) {
// excludePath.add(PathSelectors.ant(path));
// }
//
// Docket docket = new Docket(DocumentationType.SWAGGER_2)
// .host(springdocProperties.getHost())
// .apiInfo(apiInfo)
// .globalOperationParameters(assemblyGlobalOperationParameters(
// springdocProperties.getGlobalOperationParameters(),
// docketInfo.getGlobalOperationParameters()))
// .groupName(groupName)
// .select()
// .apis(RequestHandlerSelectors.basePackage(docketInfo.getBasePackage()))
// .paths(
// Predicates.and(
// Predicates.not(Predicates.or(excludePath)),
// Predicates.or(basePath)
// )
// )
// .build()
// .securitySchemes(securitySchemes())
// .securityContexts(securityContexts());
//
// configurableBeanFactory.registerSingleton(groupName, docket);
// docketList.add(docket);
// }
// return docketList;
// }
//
// /**
// * 创建 Docket对象
// *
// * @param springdocProperties swagger配置
// * @return Docket
// */
// private Docket createDocket(final SpringdocProperties springdocProperties) {
// ApiInfo apiInfo = new ApiInfoBuilder()
// .title(springdocProperties.getTitle())
// .description(springdocProperties.getDescription())
// .version(springdocProperties.getVersion())
// .license(springdocProperties.getLicense())
// .licenseUrl(springdocProperties.getLicenseUrl())
// .contact(new Contact(springdocProperties.getContact().getName(),
// springdocProperties.getContact().getUrl(),
// springdocProperties.getContact().getEmail()))
// .termsOfServiceUrl(springdocProperties.getTermsOfServiceUrl())
// .build();
//
// // base-path处理
// // 当没有配置任何path的时候,解析/**
// if (springdocProperties.getBasePath().isEmpty()) {
// springdocProperties.getBasePath().add("/**");
// }
// List> basePath = new ArrayList<>();
// for (String path : springdocProperties.getBasePath()) {
// basePath.add(PathSelectors.ant(path));
// }
//
// // exclude-path处理
// List> excludePath = new ArrayList<>();
// for (String path : springdocProperties.getExcludePath()) {
// excludePath.add(PathSelectors.ant(path));
// }
//
// return new Docket(DocumentationType.SWAGGER_2)
// .host(springdocProperties.getHost())
// .apiInfo(apiInfo)
// .globalOperationParameters(buildGlobalOperationParametersFromSwaggerProperties(
// springdocProperties.getGlobalOperationParameters()))
// .select()
// .apis(RequestHandlerSelectors.basePackage(springdocProperties.getBasePackage()))
// .paths(
// Predicates.and(
// Predicates.not(Predicates.or(excludePath)),
// Predicates.or(basePath)
// )
// )
// .build()
// .securitySchemes(securitySchemes())
// .securityContexts(securityContexts());
// }
//
//
// private List securityContexts() {
// List contexts = new ArrayList<>(1);
// SecurityContext securityContext = SecurityContext.builder()
// .securityReferences(defaultAuth())
// //.forPaths(PathSelectors.regex("^(?!auth).*$"))
// .build();
// contexts.add(securityContext);
// return contexts;
// }
//
// private List defaultAuth() {
// AuthorizationScope authorizationScope = new AuthorizationScope("global",
// "accessEverything");
// AuthorizationScope[] authorizationScopes = new AuthorizationScope[1];
// authorizationScopes[0] = authorizationScope;
// List references = new ArrayList<>(1);
// references.add(new SecurityReference(AUTH_KEY, authorizationScopes));
// return references;
// }
//
// private List securitySchemes() {
// List apiKeys = new ArrayList<>(1);
// ApiKey apiKey = new ApiKey(AUTH_KEY, AUTH_KEY, "header");
// apiKeys.add(apiKey);
// return apiKeys;
// }
//
// private List buildGlobalOperationParametersFromSwaggerProperties(
// List globalOperationParameters) {
// List parameters = Lists.newArrayList();
//
// if (Objects.isNull(globalOperationParameters)) {
// return parameters;
// }
// for (SpringdocProperties.GlobalOperationParameter globalOperationParameter : globalOperationParameters) {
// parameters.add(new ParameterBuilder()
// .name(globalOperationParameter.getName())
// .description(globalOperationParameter.getDescription())
// .modelRef(new ModelRef(globalOperationParameter.getModelRef()))
// .parameterType(globalOperationParameter.getParameterType())
// .required(Boolean.parseBoolean(globalOperationParameter.getRequired()))
// .build());
// }
// return parameters;
// }
//
// /**
// * 按照name覆盖局部参数
// *
// * @param globalOperationParameters globalOperationParameters
// * @param docketOperationParameters docketOperationParameters
// * @return java.util.List
// * @author shuigedeng
// * @since 2020/4/30 10:10
// */
// private List assemblyGlobalOperationParameters(
// List globalOperationParameters,
// List docketOperationParameters) {
//
// if (Objects.isNull(docketOperationParameters) || docketOperationParameters.isEmpty()) {
// return buildGlobalOperationParametersFromSwaggerProperties(globalOperationParameters);
// }
//
// Set docketNames = docketOperationParameters.stream()
// .map(SpringdocProperties.GlobalOperationParameter::getName)
// .collect(Collectors.toSet());
//
// List resultOperationParameters = Lists
// .newArrayList();
//
// if (Objects.nonNull(globalOperationParameters)) {
// for (SpringdocProperties.GlobalOperationParameter parameter : globalOperationParameters) {
// if (!docketNames.contains(parameter.getName())) {
// resultOperationParameters.add(parameter);
// }
// }
// }
//
// resultOperationParameters.addAll(docketOperationParameters);
// return buildGlobalOperationParametersFromSwaggerProperties(resultOperationParameters);
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy