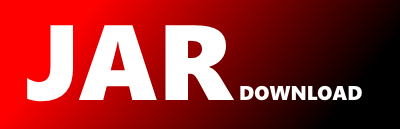
com.taotao.cloud.openfeign.aspect.FeignRetryAspect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of taotao-cloud-starter-openfeign Show documentation
Show all versions of taotao-cloud-starter-openfeign Show documentation
taotao-cloud-starter-openfeign
/*
* Copyright (c) 2020-2030, Shuigedeng ([email protected] & https://blog.taotaocloud.top/).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.taotao.cloud.openfeign.aspect;
import com.taotao.cloud.common.exception.BaseException;
import com.taotao.cloud.common.utils.log.LogUtils;
import com.taotao.cloud.openfeign.annotation.FeignRetry;
import feign.RetryableException;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.Map;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.reflect.MethodSignature;
import org.springframework.retry.backoff.BackOffPolicy;
import org.springframework.retry.backoff.ExponentialBackOffPolicy;
import org.springframework.retry.backoff.FixedBackOffPolicy;
import org.springframework.retry.policy.SimpleRetryPolicy;
import org.springframework.retry.support.RetryTemplate;
import org.springframework.stereotype.Component;
/**
* 假装重试方面
*
* @author shuigedeng
* @version 2023.07
* @since 2023-07-06 13:41:00
*/
@Aspect
@Component
public class FeignRetryAspect {
/**
* 重试
*
* @param joinPoint 连接点
* @return {@link Object }
* @since 2023-07-06 13:41:00
*/
@Around("@annotation(com.taotao.cloud.openfeign.annotation.FeignRetry)")
public Object retry(ProceedingJoinPoint joinPoint) throws Throwable {
Method method = getCurrentMethod(joinPoint);
FeignRetry feignRetry = method.getAnnotation(FeignRetry.class);
RetryTemplate retryTemplate = new RetryTemplate();
retryTemplate.setBackOffPolicy(prepareBackOffPolicy(feignRetry));
retryTemplate.setRetryPolicy(prepareSimpleRetryPolicy(feignRetry));
// 重试
return retryTemplate.execute(retryCallback -> {
int retryCount = retryCallback.getRetryCount();
LogUtils.info(
"Sending request method: {}, max attempt: {}, delay: {}, retryCount:" + " {}",
method.getName(),
feignRetry.maxAttempt(),
feignRetry.backoff().delay(),
retryCount);
return joinPoint.proceed(joinPoint.getArgs());
});
}
/**
* 准备退出政策
*
* @param feignRetry 假装重试
* @return {@link BackOffPolicy }
* @since 2023-07-06 13:41:00
*/
private BackOffPolicy prepareBackOffPolicy(FeignRetry feignRetry) {
if (feignRetry.backoff().multiplier() != 0) {
ExponentialBackOffPolicy backOffPolicy = new ExponentialBackOffPolicy();
backOffPolicy.setInitialInterval(feignRetry.backoff().delay());
backOffPolicy.setMaxInterval(feignRetry.backoff().maxDelay());
backOffPolicy.setMultiplier(feignRetry.backoff().multiplier());
return backOffPolicy;
} else {
FixedBackOffPolicy fixedBackOffPolicy = new FixedBackOffPolicy();
fixedBackOffPolicy.setBackOffPeriod(feignRetry.backoff().delay());
return fixedBackOffPolicy;
}
}
/**
* 准备简单重试策略
*
* @param feignRetry 假装重试
* @return {@link SimpleRetryPolicy }
* @since 2023-07-06 13:41:00
*/
private SimpleRetryPolicy prepareSimpleRetryPolicy(FeignRetry feignRetry) {
Map, Boolean> policyMap = new HashMap<>();
policyMap.put(RetryableException.class, true); // Connection refused or time out
// policyMap.put(ClientException.class, true); // Load balance does not available (cause
// of RunTimeException)
policyMap.put(BaseException.class, true); // Load balance does not available (cause of RunTimeException)
for (Class extends Throwable> t : feignRetry.include()) {
policyMap.put(t, true);
}
return new SimpleRetryPolicy(feignRetry.maxAttempt(), policyMap, true);
}
/**
* 获取当前方法
*
* @param joinPoint 连接点
* @return {@link Method }
* @since 2023-07-06 13:41:00
*/
private Method getCurrentMethod(JoinPoint joinPoint) {
MethodSignature signature = (MethodSignature) joinPoint.getSignature();
return signature.getMethod();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy