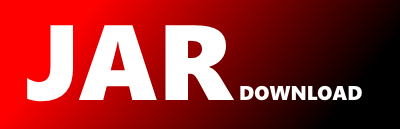
com.sicheng.common.persistence.proxy.PaginationMapperMethod Maven / Gradle / Ivy
/**
* 本作品使用 木兰公共许可证,第2版(Mulan PubL v2) 开源协议,请遵守相关条款,或者联系sicheng.net获取商用授权。
* Copyright (c) 2016 SiCheng.Net
* This software is licensed under Mulan PubL v2.
* You can use this software according to the terms and conditions of the Mulan PubL v2.
* You may obtain a copy of Mulan PubL v2 at:
* http://license.coscl.org.cn/MulanPubL-2.0
* THIS SOFTWARE IS PROVIDED ON AN "AS IS" BASIS, WITHOUT WARRANTIES OF ANY KIND,
* EITHER EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO NON-INFRINGEMENT,
* MERCHANTABILITY OR FIT FOR A PARTICULAR PURPOSE.
* See the Mulan PubL v2 for more details.
*/
package com.sicheng.common.persistence.proxy;
import com.sicheng.common.persistence.Page;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.binding.BindingException;
import org.apache.ibatis.mapping.MappedStatement;
import org.apache.ibatis.mapping.SqlCommandType;
import org.apache.ibatis.session.Configuration;
import org.apache.ibatis.session.RowBounds;
import org.apache.ibatis.session.SqlSession;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
*
* 执行代理类,扩展Mybatis的方式来让其Mapper接口来支持.
*
*
* @author zhaolei
* @version 1.0 2012-05-13 上午10:09
*
*/
public class PaginationMapperMethod {
private final SqlSession sqlSession;
private final Configuration config;
private SqlCommandType type;
private String commandName;
private String commandCountName;
private final Class> declaringInterface;
private final Method method;
private Integer rowBoundsIndex;
private Integer paginationIndex;
private final List paramNames;
private final List paramPositions;
private boolean hasNamedParameters;
public PaginationMapperMethod(Class> declaringInterface, Method method,
SqlSession sqlSession) {
paramNames = new ArrayList();
paramPositions = new ArrayList();
this.sqlSession = sqlSession;
this.method = method;
this.config = sqlSession.getConfiguration();
this.declaringInterface = declaringInterface;
this.hasNamedParameters = false;
setupFields();
setupMethodSignature();
setupCommandType();
validateStatement();
}
/**
* 代理执行方法。
*
* @param args 参数信息
* @return 执行结果
*/
@SuppressWarnings("unchecked")
public Object execute(Object[] args) {
final Object param = getParam(args);
Page
© 2015 - 2025 Weber Informatics LLC | Privacy Policy