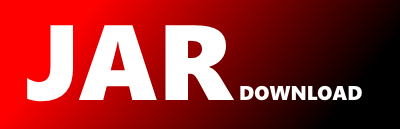
io.github.sinri.keel.cache.impl.KeelCacheAlef Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Keel Show documentation
Show all versions of Keel Show documentation
A website framework with VERT.X for ex-PHP-ers, exactly Ark Framework Users.
The newest version!
package io.github.sinri.keel.cache.impl;
import io.github.sinri.keel.cache.KeelCacheInterface;
import io.github.sinri.keel.cache.ValueWrapper;
import javax.annotation.Nonnull;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
/**
* An implementation of KeelCacheInterface, using ConcurrentHashMap.
*
* @since 2.5
*/
public class KeelCacheAlef implements KeelCacheInterface {
private final ConcurrentMap> map;
private long defaultLifeInSeconds = 1000L;
public KeelCacheAlef() {
this.map = new ConcurrentHashMap<>();
}
@Override
public long getDefaultLifeInSeconds() {
return defaultLifeInSeconds;
}
@Override
public KeelCacheInterface setDefaultLifeInSeconds(long lifeInSeconds) {
defaultLifeInSeconds = lifeInSeconds;
return this;
}
@Override
public void save(@Nonnull K key, V value, long lifeInSeconds) {
this.map.put(key, new ValueWrapper<>(value, lifeInSeconds));
}
@Override
public V read(@Nonnull K key, V fallbackValue) {
ValueWrapper vw = this.map.get(key);
if (vw == null) {
return fallbackValue;
}
if (vw.isAliveNow()) {
return vw.getValue();
} else {
return fallbackValue;
}
}
@Override
public void remove(@Nonnull K key) {
this.map.remove(key);
}
@Override
public void removeAll() {
this.map.clear();
}
@Override
public void cleanUp() {
this.map.keySet().forEach(key -> {
ValueWrapper vw = this.map.get(key);
if (vw != null) {
if (!vw.isAliveNow()) {
this.map.remove(key, vw);
}
}
});
}
@Override
@Nonnull
public ConcurrentMap getSnapshotMap() {
ConcurrentMap snapshot = new ConcurrentHashMap<>();
this.map.keySet().forEach(key -> {
ValueWrapper vw = this.map.get(key);
if (vw != null) {
if (vw.isAliveNow()) {
snapshot.put(key, vw.getValue());
}
}
});
return snapshot;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy