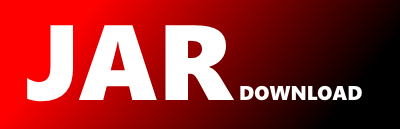
io.github.sinri.keel.cache.impl.KeelCacheBet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Keel Show documentation
Show all versions of Keel Show documentation
A website framework with VERT.X for ex-PHP-ers, exactly Ark Framework Users.
The newest version!
package io.github.sinri.keel.cache.impl;
import io.github.sinri.keel.cache.KeelAsyncCacheInterface;
import io.github.sinri.keel.cache.ValueWrapper;
import io.vertx.core.Future;
import javax.annotation.Nonnull;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import java.util.function.Function;
public class KeelCacheBet implements KeelAsyncCacheInterface {
private final ConcurrentMap> map;
public KeelCacheBet() {
this.map = new ConcurrentHashMap<>();
}
@Override
public Future save(@Nonnull K key, V value, long lifeInSeconds) {
this.map.put(key, new ValueWrapper<>(value, lifeInSeconds));
return Future.succeededFuture();
}
@Override
public Future read(@Nonnull K key) {
ValueWrapper vw = this.map.get(key);
if (vw == null || !vw.isAliveNow()) {
return Future.failedFuture(new NotCached(key.toString()));
}
return Future.succeededFuture(vw.getValue());
}
@Override
public Future read(@Nonnull K key, V fallbackValue) {
ValueWrapper vw = this.map.get(key);
if (vw == null) {
return Future.succeededFuture(fallbackValue);
} else {
if (vw.isAliveNow()) {
return Future.succeededFuture(vw.getValue());
} else {
return Future.succeededFuture(fallbackValue);
}
}
}
@Override
public Future read(@Nonnull K key, Function> generator, long lifeInSeconds) {
// i.e. computeIfAbsent
ValueWrapper vw = this.map.get(key);
if (vw != null && vw.isAliveNow()) {
return Future.succeededFuture(vw.getValue());
} else {
return generator.apply(key)
.compose(v -> {
return save(key, v, lifeInSeconds)
.compose(saved -> {
return Future.succeededFuture(v);
});
});
}
}
@Override
public Future remove(@Nonnull K key) {
this.map.remove(key);
return Future.succeededFuture();
}
@Override
public Future removeAll() {
this.map.clear();
return Future.succeededFuture();
}
@Override
public Future cleanUp() {
this.map.keySet().forEach(key -> {
ValueWrapper vw = this.map.get(key);
if (vw != null) {
if (!vw.isAliveNow()) {
this.map.remove(key, vw);
}
}
});
return Future.succeededFuture();
}
@Override
public Future> getSnapshotMap() {
ConcurrentMap snapshot = new ConcurrentHashMap<>();
this.map.keySet().forEach(key -> {
ValueWrapper vw = this.map.get(key);
if (vw != null) {
if (vw.isAliveNow()) {
snapshot.put(key, vw.getValue());
}
}
});
return Future.succeededFuture(snapshot);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy