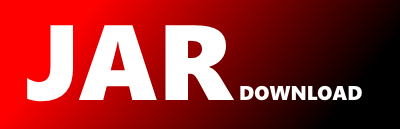
io.github.sinri.keel.cache.impl.KeelCacheDalet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Keel Show documentation
Show all versions of Keel Show documentation
A website framework with VERT.X for ex-PHP-ers, exactly Ark Framework Users.
The newest version!
package io.github.sinri.keel.cache.impl;
import io.github.sinri.keel.cache.KeelEverlastingCacheInterface;
import io.github.sinri.keel.facade.async.KeelAsyncKit;
import io.github.sinri.keel.verticles.KeelVerticleImplPure;
import io.vertx.core.Future;
import io.vertx.core.Promise;
import javax.annotation.Nonnull;
import java.util.Collection;
import java.util.Collections;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
/**
* This implement is to provide:
* 1. initialized cache data;
* 2. everlasting cache till modified;
* 3. regular updating.
*
* @since 3.2.11
*/
abstract public class KeelCacheDalet extends KeelVerticleImplPure implements KeelEverlastingCacheInterface {
private final ConcurrentMap map = new ConcurrentHashMap<>();
/**
* Save the item to cache.
*
* @param key key
* @param value value
*/
@Override
public void save(@Nonnull String key, String value) {
this.map.put(key, value);
}
@Override
public void save(@Nonnull Map appendEntries) {
this.map.putAll(appendEntries);
}
/**
* @param key key
* @param value default value for the situation that key not existed
* @return @return cache value or default when not-existed
*/
@Override
public String read(@Nonnull String key, String value) {
return map.getOrDefault(key, value);
}
/**
* Remove the cached item with key.
*
* @param key key
*/
@Override
public void remove(@Nonnull String key) {
this.map.remove(key);
}
@Override
public void remove(@Nonnull Collection keys) {
keys.forEach(this.map::remove);
}
/**
* Remove all the cached items.
*/
@Override
public void removeAll() {
map.clear();
}
/**
* Replace all entries in cache map with new entries.
*
* @param newEntries new map of entries
*/
@Override
public void replaceAll(@Nonnull Map newEntries) {
newEntries.forEach(map::replace);
}
/**
* @return ConcurrentMap K → V alive value only
* @since 1.14
*/
@Nonnull
@Override
public Map getSnapshotMap() {
return Collections.unmodifiableMap(map);
}
@Override
final protected void startAsPureKeelVerticle() {
// do nothing
}
@Override
protected void startAsPureKeelVerticle(Promise startPromise) {
fullyUpdate()
.onSuccess(updated -> {
if (regularUpdatePeriod() >= 0) {
KeelAsyncKit.endless(() -> {
return Future.succeededFuture()
.compose(v -> {
if (regularUpdatePeriod() == 0) return Future.succeededFuture();
else return KeelAsyncKit.sleep(regularUpdatePeriod());
})
.compose(v -> {
return fullyUpdate();
});
});
}
startPromise.complete();
})
.onFailure(startPromise::fail);
}
abstract public Future fullyUpdate();
/**
* @return a time period to sleep between regular updates. Use minus number to disable regular update.
*/
protected long regularUpdatePeriod() {
return 600_000L;// by default, 10min.
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy