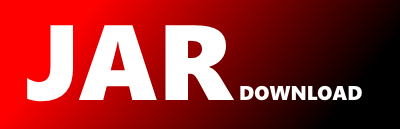
io.github.sinri.keel.elasticsearch.index.ESIndexMixin Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Keel Show documentation
Show all versions of Keel Show documentation
A website framework with VERT.X for ex-PHP-ers, exactly Ark Framework Users.
The newest version!
package io.github.sinri.keel.elasticsearch.index;
import io.github.sinri.keel.elasticsearch.ESApiMixin;
import io.vertx.core.Future;
import io.vertx.core.http.HttpMethod;
import io.vertx.core.json.JsonObject;
import java.nio.charset.StandardCharsets;
import java.util.Objects;
/**
* @since 3.0.7
*/
public interface ESIndexMixin extends ESApiMixin {
/**
* @since 3.2.20
* @see Create Index - Path Parameters - Index Name
*/
static boolean isLegalIndexName(String indexName) {
if (indexName == null) {
return false;
}
return (indexName.getBytes(StandardCharsets.UTF_8).length < 255)
&& Objects.equals(indexName.toLowerCase(), indexName)
&& (!indexName.contains("/") && !indexName.contains("\\")
&& !indexName.contains("*") && !indexName.contains("?")
&& !indexName.contains("\"") && !indexName.contains("<") && !indexName.contains(">")
&& !indexName.contains("|") && !indexName.contains(" ")
&& !indexName.contains(",") && !indexName.contains("#") && !indexName.contains(":"))
&& (!indexName.startsWith("_") && !indexName.startsWith("-")
&& !indexName.startsWith("+") && !indexName.startsWith("."));
}
/**
* @param indexName (Required, string) Comma-separated list of data streams, indices, and aliases used to limit the request. Supports wildcards (*). To target all data streams and indices, omit this parameter or use * or _all.
* @see Get index API
* If the Elasticsearch security features are enabled, you must have the view_index_metadata or manage index privilege for the target data stream, index, or alias.
*/
default Future indexGet(String indexName, ESApiQueries queries) {
return call(HttpMethod.GET, "/" + indexName, queries, null)
.compose(resp -> {
return Future.succeededFuture(new ESIndexGetResponse(resp));
});
}
/**
* @see Create index API
*/
default Future indexCreate(String indexName, ESApiQueries queries, JsonObject requestBody) {
return call(HttpMethod.PUT, "/" + indexName, queries, requestBody.toString())
.compose(resp -> {
return Future.succeededFuture(new ESIndexCreateResponse(resp));
});
}
/**
* @see Delete index API
*/
default Future indexDelete(String indexName, ESApiQueries queries) {
return call(HttpMethod.DELETE, "/" + indexName, queries, null)
.compose(resp -> {
return Future.succeededFuture(new ESIndexDeleteResponse(resp));
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy