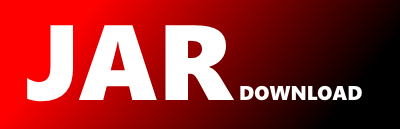
io.github.sinri.keel.mysql.matrix.ResultMatrix Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Keel Show documentation
Show all versions of Keel Show documentation
A website framework with VERT.X for ex-PHP-ers, exactly Ark Framework Users.
The newest version!
package io.github.sinri.keel.mysql.matrix;
import io.github.sinri.keel.helper.KeelHelpersInterface;
import io.github.sinri.keel.mysql.exception.KeelSQLResultRowIndexError;
import io.vertx.core.Future;
import io.vertx.core.json.JsonArray;
import io.vertx.core.json.JsonObject;
import io.vertx.sqlclient.Row;
import io.vertx.sqlclient.RowSet;
import io.vertx.sqlclient.data.Numeric;
import java.lang.reflect.InvocationTargetException;
import java.util.*;
import java.util.function.BiConsumer;
import java.util.function.Function;
/**
* @since 1.1
* @since 1.8 becomes interface
* May overrides this class to get Customized Data Matrix
*/
public interface ResultMatrix {
/**
* @since 1.10
*/
static T buildTableRow(JsonObject row, Class classOfTableRow) throws NoSuchMethodException, InvocationTargetException, InstantiationException, IllegalAccessException {
return classOfTableRow.getConstructor(JsonObject.class).newInstance(row);
}
/**
* @since 1.10
*/
static List buildTableRowList(List rowList, Class classOfTableRow) throws NoSuchMethodException, InvocationTargetException, InstantiationException, IllegalAccessException {
ArrayList list = new ArrayList<>();
for (var x : rowList) {
list.add(ResultMatrix.buildTableRow(x, classOfTableRow));
}
return list;
}
/**
* @since 2.8
*/
static ResultMatrix create(RowSet rowSet) {
return new ResultMatrixImpl(rowSet);
}
List getRowList();
int getTotalFetchedRows();
int getTotalAffectedRows();
long getLastInsertedID();
JsonArray toJsonArray();
JsonObject getFirstRow() throws KeelSQLResultRowIndexError;
JsonObject getRowByIndex(int index) throws KeelSQLResultRowIndexError;
/**
* @since 1.10
*/
T buildTableRowByIndex(int index, Class classOfTableRow) throws KeelSQLResultRowIndexError;
String getOneColumnOfFirstRowAsDateTime(String columnName) throws KeelSQLResultRowIndexError;
String getOneColumnOfFirstRowAsString(String columnName) throws KeelSQLResultRowIndexError;
Numeric getOneColumnOfFirstRowAsNumeric(String columnName) throws KeelSQLResultRowIndexError;
Integer getOneColumnOfFirstRowAsInteger(String columnName) throws KeelSQLResultRowIndexError;
Long getOneColumnOfFirstRowAsLong(String columnName) throws KeelSQLResultRowIndexError;
List getOneColumnAsDateTime(String columnName);
List getOneColumnAsString(String columnName);
List getOneColumnAsNumeric(String columnName);
List getOneColumnAsLong(String columnName);
List getOneColumnAsInteger(String columnName);
/**
* @throws RuntimeException 封装类的时候可能会抛出异常
* @since 1.10
*/
List buildTableRowList(Class classOfTableRow);
/**
* @since 2.9.4
*/
default Future
© 2015 - 2024 Weber Informatics LLC | Privacy Policy