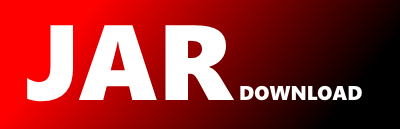
io.github.sinri.keel.web.http.receptionist.KeelWebReceptionistKit Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Keel Show documentation
Show all versions of Keel Show documentation
A website framework with VERT.X for ex-PHP-ers, exactly Ark Framework Users.
The newest version!
package io.github.sinri.keel.web.http.receptionist;
import io.github.sinri.keel.web.http.ApiMeta;
import io.github.sinri.keel.web.http.prehandler.KeelPlatformHandler;
import io.vertx.core.Handler;
import io.vertx.core.http.HttpMethod;
import io.vertx.core.json.JsonArray;
import io.vertx.ext.web.Route;
import io.vertx.ext.web.Router;
import io.vertx.ext.web.RoutingContext;
import io.vertx.ext.web.handler.*;
import javax.annotation.Nonnull;
import java.lang.reflect.Constructor;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Set;
import static io.github.sinri.keel.facade.KeelInstance.Keel;
import static io.github.sinri.keel.helper.KeelHelpersInterface.KeelHelpers;
/**
* @param class of a subclass of KeelWebReceptionist
* @see io.github.sinri.keel.web.http.receptionist.KeelWebReceptionistLoader
* @since 2.9.2
* @since 3.0.0 TEST PASSED
* @deprecated since 3.2.13, use `KeelWebReceptionistLoader` instead.
*/
@Deprecated(since = "3.2.13")
public class KeelWebReceptionistKit {
private final Router router;
private final Class classOfReceptionist;
private final List platformHandlers = new ArrayList<>();
private final List securityPolicyHandlers = new ArrayList<>();
private final List protocolUpgradeHandlers = new ArrayList<>();
private final List multiTenantHandlers = new ArrayList<>();
/**
* Tells who the user is
*/
private final List authenticationHandlers = new ArrayList<>();
private final List inputTrustHandlers = new ArrayList<>();
/**
* Tells what the user is allowed to do
*/
private final List authorizationHandlers = new ArrayList<>();
private final List> userHandlers = new ArrayList<>();
private String uploadDirectory = BodyHandler.DEFAULT_UPLOADS_DIRECTORY;
private String virtualHost = null;
/**
* @since 2.9.2
*/
private Handler failureHandler = null;
public KeelWebReceptionistKit(Class classOfReceptionist, Router router) {
this.classOfReceptionist = classOfReceptionist;
this.router = router;
}
/**
* Note: MAIN and TEST scopes are seperated.
*
* @param packageName the name of the package where the classes extending `R` are.
* @since 3.2.11 Removed org.reflections:reflections, now self-implemented.
*/
public void loadPackage(String packageName) {
Set> allClasses = Keel.reflectionHelper()
.seekClassDescendantsInPackage(packageName, classOfReceptionist);
try {
allClasses.forEach(this::loadClass);
} catch (Exception e) {
Keel.getLogger().exception(e, r -> r.classification(getClass().getName(), "loadPackage"));
}
}
public void loadClass(Class extends R> c) {
ApiMeta[] apiMetaArray = KeelHelpers.reflectionHelper().getAnnotationsOfClass(c, ApiMeta.class);
for (var apiMeta : apiMetaArray) {
loadClass(c, apiMeta);
}
}
private void loadClass(Class extends R> c, @Nonnull ApiMeta apiMeta) {
Keel.getLogger().info(r -> r
.classification(getClass().getName(), "loadClass")
.message("Loading " + c.getName())
.context(j -> {
JsonArray methods = new JsonArray();
Arrays.stream(apiMeta.allowMethods()).forEach(methods::add);
j.put("allowMethods", methods);
j.put("routePath", apiMeta.routePath());
if (apiMeta.isDeprecated()) {
j.put("isDeprecated", true);
}
if (apiMeta.remark() != null && !apiMeta.remark().isEmpty()) {
j.put("remark", apiMeta.remark());
}
})
);
Constructor extends R> receptionistConstructor;
try {
receptionistConstructor = c.getConstructor(RoutingContext.class);
} catch (NoSuchMethodException e) {
Keel.getLogger().exception(e, r -> r.classification(getClass().getName(), "loadClass").message("HANDLER REFLECTION EXCEPTION"));
return;
}
Route route = router.route(apiMeta.routePath());
if (apiMeta.allowMethods() != null) {
for (var methodName : apiMeta.allowMethods()) {
route.method(HttpMethod.valueOf(methodName));
}
}
if (apiMeta.virtualHost() != null && !apiMeta.virtualHost().isEmpty()) {
route.virtualHost(apiMeta.virtualHost());
} else if (this.virtualHost != null && !this.virtualHost.isEmpty()) {
route.virtualHost(this.virtualHost);
}
// === HANDLERS WEIGHT IN ORDER ===
// PLATFORM
route.handler(new KeelPlatformHandler());
if (apiMeta.timeout() > 0) {
// PlatformHandler
route.handler(TimeoutHandler.create(apiMeta.timeout(), apiMeta.statusCodeForTimeout()));
}
route.handler(ResponseTimeHandler.create());
this.platformHandlers.forEach(route::handler);
// SECURITY_POLICY,
// SecurityPolicyHandler
// CorsHandler: Cross Origin Resource Sharing
this.securityPolicyHandlers.forEach(route::handler);
// PROTOCOL_UPGRADE,
protocolUpgradeHandlers.forEach(route::handler);
// BODY,
if (apiMeta.requestBodyNeeded()) {
route.handler(BodyHandler.create(uploadDirectory));
}
// MULTI_TENANT,
multiTenantHandlers.forEach(route::handler);
// AUTHENTICATION,
authenticationHandlers.forEach(route::handler);
// INPUT_TRUST,
inputTrustHandlers.forEach(route::handler);
// AUTHORIZATION,
authorizationHandlers.forEach(route::handler);
// USER
userHandlers.forEach(route::handler);
// finally!
route.handler(routingContext -> {
try {
R receptionist = receptionistConstructor.newInstance(routingContext);
//receptionist.setApiMeta(apiMeta);
receptionist.handle();
} catch (Throwable e) {
routingContext.fail(e);
}
});
// failure handler since 2.9.2
if (failureHandler != null) {
route.failureHandler(failureHandler);
}
}
/**
* @since 2.9.2
*/
public KeelWebReceptionistKit setFailureHandler(Handler failureHandler) {
this.failureHandler = failureHandler;
return this;
}
public KeelWebReceptionistKit addPlatformHandler(PlatformHandler handler) {
this.platformHandlers.add(handler);
return this;
}
public KeelWebReceptionistKit addSecurityPolicyHandler(SecurityPolicyHandler handler) {
this.securityPolicyHandlers.add(handler);
return this;
}
public KeelWebReceptionistKit addProtocolUpgradeHandler(ProtocolUpgradeHandler handler) {
this.protocolUpgradeHandlers.add(handler);
return this;
}
public KeelWebReceptionistKit addMultiTenantHandler(MultiTenantHandler handler) {
this.multiTenantHandlers.add(handler);
return this;
}
/**
* 追加一个认证校验器
*/
public KeelWebReceptionistKit addAuthenticationHandler(AuthenticationHandler handler) {
this.authenticationHandlers.add(handler);
return this;
}
public KeelWebReceptionistKit addInputTrustHandler(InputTrustHandler handler) {
this.inputTrustHandlers.add(handler);
return this;
}
/**
* 追加一个授权校验器
*/
public KeelWebReceptionistKit addAuthorizationHandler(AuthorizationHandler handler) {
this.authorizationHandlers.add(handler);
return this;
}
public KeelWebReceptionistKit addUserHandler(Handler handler) {
this.userHandlers.add(handler);
return this;
}
public KeelWebReceptionistKit setUploadDirectory(String uploadDirectory) {
this.uploadDirectory = uploadDirectory;
return this;
}
/**
* @since 2.9
*/
public KeelWebReceptionistKit setVirtualHost(String virtualHost) {
this.virtualHost = virtualHost;
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy