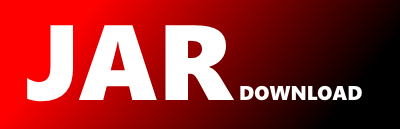
io.github.spair.byond.dmi.SpriteDir Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of byond-dmi-util Show documentation
Show all versions of byond-dmi-util Show documentation
Small set of util classes to work with BYOND dmi files.
package io.github.spair.byond.dmi;
public enum SpriteDir {
SOUTH(2, "S", 1),
NORTH(1, "N", 2),
EAST(4, "E", 3),
WEST(8, "W", 4),
SOUTHEAST(6, "SE", 5),
SOUTHWEST(10, "SW", 6),
NORTHEAST(5, "NE", 7),
NORTHWEST(9, "NW", 8);
/**
* BYOND representation of dir as int value.
*
* - SOUTH - 2
* - NORTH - 1
* - EAST - 4
* - WEST - 8
* - SOUTHEAST - 6
* - SOUTHWEST - 10
* - NORTHEAST - 5
* - NORTHWEST - 9
*
*/
public final int dirValue;
/**
* Shorted string representation of constant values.
*
* - SOUTH - S
* - NORTH - N
* - EAST - E
* - WEST - W
* - SOUTHEAST - SE
* - SOUTHWEST - SW
* - NORTHEAST - NE
* - NORTHWEST - NW
*
*/
public final String shortName;
// Used to sort dirs in BYOND like order.
final int compareWeight;
SpriteDir(final int dirValue, final String shortName, final int compareWeight) {
this.dirValue = dirValue;
this.shortName = shortName;
this.compareWeight = compareWeight;
}
/**
* Returns {@link SpriteDir} equivalent to BYOND dir value. If method got value different from the list below,
* {@link IllegalArgumentException} will be thrown.
*
* - 0,1,3,7 - NORTH
* - 2 - SOUTH
* - 4 - EAST
* - 8 - WEST
* - 5 - NORTHEAST
* - 6 - SOUTHEAST
* - 9 - NORTHWEST
* - 10 - SOUTHWEST
*
*
* @param dirValue BYOND representation of dir
* @return {@link SpriteDir} equivalent of BYOND dir value
*/
@SuppressWarnings("checkstyle:MagicNumber")
public static SpriteDir valueOfByondDir(final int dirValue) {
switch (dirValue) {
case 0: case 1: case 3: case 7:
return NORTH;
case 2:
return SOUTH;
case 4:
return EAST;
case 8:
return WEST;
case 5:
return NORTHEAST;
case 6:
return SOUTHEAST;
case 9:
return NORTHWEST;
case 10:
return SOUTHWEST;
default:
throw new IllegalArgumentException("Illegal value of BYOND dir. Dir value: " + dirValue);
}
}
/**
* Same as {@link #valueOfByondDir(int)}, but accept value as string instead of int.
*
* @param dirValue BYOND representation of dir
* @return {@link SpriteDir} equivalent of BYOND dir value
*/
public static SpriteDir valueOfByondDir(final String dirValue) {
return valueOfByondDir(Integer.parseInt(dirValue));
}
// During DMI slurping all dirs are passed in `for(i = 0; i <= n; i++)` cycle.
// This method determines the order in which dirs are placed in `.dmi` file.
@SuppressWarnings("checkstyle:MagicNumber")
static SpriteDir valueOf(final int dirCount) {
switch (dirCount) {
case 1:
return SOUTH;
case 2:
return NORTH;
case 3:
return EAST;
case 4:
return WEST;
case 5:
return SOUTHEAST;
case 6:
return SOUTHWEST;
case 7:
return NORTHEAST;
case 8:
return NORTHWEST;
default:
return SOUTH;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy