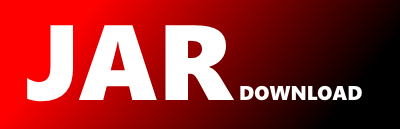
org.springframework.social.partnercenter.api.PagingResourceTemplate Maven / Gradle / Ivy
package org.springframework.social.partnercenter.api;
import static org.springframework.social.partnercenter.http.PartnerCenterHttpHeaders.MS_CONTINUATION_TOKEN;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.http.ResponseEntity;
import org.springframework.social.partnercenter.PartnerCenter;
import org.springframework.social.partnercenter.http.client.RestResource;
public class PagingResourceTemplate extends AbstractTemplate implements PagingResourceOperations{
private final RestResource restResource;
private ParameterizedTypeReference> typeReference;
protected PagingResourceTemplate(RestResource restResource, boolean isAuthorized, ParameterizedTypeReference> typeReference) {
super(isAuthorized);
this.restResource = restResource;
this.typeReference = typeReference;
}
@Override
public ResponseEntity> next(String continuationToken){
return restResource.request()
.header(MS_CONTINUATION_TOKEN, continuationToken)
.queryParam("seekOperation", SeekOperation.NEXT.getValue())
.get(typeReference);
}
@Override
public ResponseEntity> previous(String continuationToken) {
return restResource.request()
.header(MS_CONTINUATION_TOKEN, continuationToken)
.queryParam("seekOperation", SeekOperation.PREVIOUS.getValue())
.get(typeReference);
}
@Override
public ResponseEntity> first(String continuationToken) {
return restResource.request()
.header(MS_CONTINUATION_TOKEN, continuationToken)
.queryParam("seekOperation", SeekOperation.FIRST.getValue())
.get(typeReference);
}
@Override
public ResponseEntity> last(String continuationToken) {
return restResource.request()
.header(MS_CONTINUATION_TOKEN, continuationToken)
.queryParam("seekOperation", SeekOperation.LAST.getValue())
.get(typeReference);
}
@Override
public ResponseEntity> page(String continuationToken, int pageIndex) {
return restResource.request()
.header(MS_CONTINUATION_TOKEN, continuationToken)
.queryParam("seekOperation", SeekOperation.PAGE_INDEX.getValue())
.queryParam("page", pageIndex)
.get(typeReference);
}
@Override
protected String getProviderId() {
return PartnerCenter.PROVIDER_ID;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy