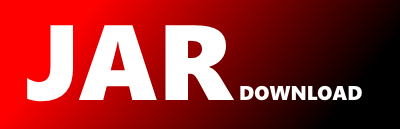
org.springframework.social.partnercenter.http.client.HttpRequestBuilder Maven / Gradle / Ivy
package org.springframework.social.partnercenter.http.client;
import static java.util.Collections.singletonList;
import static org.springframework.http.HttpStatus.FORBIDDEN;
import static org.springframework.http.HttpStatus.UNAUTHORIZED;
import java.net.URI;
import java.util.List;
import java.util.UUID;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.retry.support.RetryTemplate;
import org.springframework.social.partnercenter.api.ApiFaultException;
import org.springframework.social.partnercenter.http.PartnerCenterHttpHeaders;
import org.springframework.social.partnercenter.http.client.retry.HttpRetryService;
import org.springframework.social.partnercenter.http.client.retry.RetryBuilder;
import org.springframework.web.util.UriComponentsBuilder;
public class HttpRequestBuilder {
private PartnerCenterHttpHeaders headers;
private RestClient restResource;
private UriComponentsBuilder uriBuilder;
private HttpRetryService httpRetryService = new HttpRetryService(RetryBuilder.defaultRetry(), FORBIDDEN, UNAUTHORIZED);
HttpRequestBuilder(RestClient restResource, UriComponentsBuilder resourceBaseUri, String msRequestId, String msCorrelationId){
this.headers = new PartnerCenterHttpHeaders();
addMicrosoftTrackingHeaders(msRequestId, msCorrelationId);
this.restResource = restResource;
this.uriBuilder = resourceBaseUri;
}
HttpRequestBuilder(RestClient restResource, UriComponentsBuilder resourceBaseUri){
this.headers = new PartnerCenterHttpHeaders();
addMicrosoftTrackingHeaders();
this.restResource = restResource;
this.uriBuilder = resourceBaseUri;
}
public HttpRequestBuilder header(String key, List value){
headers.put(key, value);
return this;
}
public HttpRequestBuilder header(String key, String value){
headers.put(key, singletonList(value));
return this;
}
public HttpRequestBuilder pathSegment(String ... pathSegments){
uriBuilder.pathSegment(pathSegments);
return this;
}
public HttpRequestBuilder queryParam(String name, Object... values){
uriBuilder.queryParam(name, values);
return this;
}
public HttpRequestBuilder noRetry(){
httpRetryService = null;
return this;
}
public HttpRequestBuilder withRetry(RetryTemplate retryTemplate, HttpStatus ... statusCodeToNotRetry){
httpRetryService = new HttpRetryService(retryTemplate, statusCodeToNotRetry);
return this;
}
public HttpRequestBuilder path(String path){
uriBuilder.path(path);
return this;
}
public ResponseEntity put(T payload, Class aClass) {
HttpEntity entity = new HttpEntity<>(payload, headers);
return execute(uriBuilder.build().toUri(), HttpMethod.PUT, entity, aClass);
}
public ResponseEntity post(T payload, Class aClass) {
HttpEntity entity = new HttpEntity<>(payload, headers);
return execute(uriBuilder.build().toUri(), HttpMethod.POST, entity, aClass);
}
public ResponseEntity patch(T payload, Class aClass) {
HttpEntity entity = new HttpEntity<>(payload, headers);
return execute(uriBuilder.build().toUri(), HttpMethod.PATCH, entity, aClass);
}
public ResponseEntity get(Class aClass) {
return execute(uriBuilder.build().toUri(), HttpMethod.GET, new HttpEntity<>(headers), aClass);
}
public ResponseEntity head() {
return execute(uriBuilder.build().toUri(), HttpMethod.HEAD, new HttpEntity<>(headers), Void.class);
}
public ResponseEntity get(ParameterizedTypeReference aClass) {
return execute(uriBuilder.build().toUri(), HttpMethod.GET, new HttpEntity<>(headers), aClass);
}
public ResponseEntity delete() throws ApiFaultException{
return httpRetryService != null
? httpRetryService.doWithRetry(() -> restResource.delete(uriBuilder.build().toUri(), headers))
: restResource.delete(uriBuilder.build().toUri(), headers);
}
private ResponseEntity execute(URI uri, HttpMethod httpMethod, HttpEntity entity, Class responseType){
return httpRetryService != null
? httpRetryService.doWithRetry(() -> restResource.execute(uri, httpMethod, entity, responseType))
: restResource.execute(uri, httpMethod, entity, responseType);
}
private ResponseEntity execute(URI uri, HttpMethod httpMethod, HttpEntity entity, ParameterizedTypeReference responseType) {
return httpRetryService != null
? httpRetryService.doWithRetry(() -> restResource.execute(uri, httpMethod, entity, responseType))
: restResource.execute(uri, httpMethod, entity, responseType);
}
private void addMicrosoftTrackingHeaders() {
headers.setMsRequestId(UUID.randomUUID().toString());
headers.setMsCorrelationId(UUID.randomUUID().toString());
}
private void addMicrosoftTrackingHeaders(String msRequestId, String msCorrelationId) {
headers.setMsRequestId(msRequestId);
headers.setMsCorrelationId(msCorrelationId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy