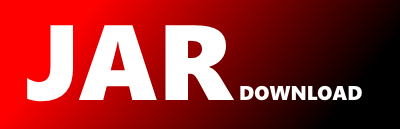
io.github.stavshamir.springwolf.asyncapi.MessageHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of springwolf-core Show documentation
Show all versions of springwolf-core Show documentation
Automated JSON API documentation for async APIs (Kafka etc.) interfaces built with Spring
package io.github.stavshamir.springwolf.asyncapi;
import io.github.stavshamir.springwolf.asyncapi.types.channel.operation.message.Message;
import lombok.extern.slf4j.Slf4j;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
import java.util.function.Supplier;
import java.util.stream.Collectors;
@Slf4j
public class MessageHelper {
private static final String ONE_OF = "oneOf";
private static final Comparator byMessageName = Comparator.comparing(Message::getName);
private static final Supplier> messageSupplier = () -> new TreeSet<>(byMessageName);
public static Object toMessageObjectOrComposition(Set messages) {
return switch (messages.size()) {
case 0 -> throw new IllegalArgumentException("messages must not be empty");
case 1 -> messages.toArray()[0];
default -> Map.of(
ONE_OF, new ArrayList<>(messages.stream().collect(Collectors.toCollection(messageSupplier))));
};
}
@SuppressWarnings("unchecked")
public static Set messageObjectToSet(Object messageObject) {
if (messageObject instanceof Message message) {
return new HashSet<>(Collections.singletonList(message));
}
if (messageObject instanceof Map) {
List messages = ((Map>) messageObject).get(ONE_OF);
return new HashSet<>(messages);
}
log.warn(
"Message object must contain either a Message or a Map, but contained: {}",
messageObject.getClass());
return new HashSet<>();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy