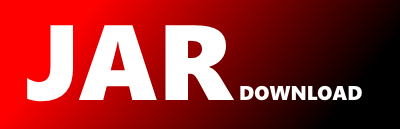
io.github.stewseo.client.transport.endpoints.SimpleEndpoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yelp-fusion-client Show documentation
Show all versions of yelp-fusion-client Show documentation
java client to build api objects, handle http transport, and parse/deserialize/serialize json to/from json
package io.github.stewseo.client.transport.endpoints;
import io.github.stewseo.client._types.ErrorResponse;
import io.github.stewseo.client.json.JsonpDeserializer;
import io.github.stewseo.client.transport.EndpointBase;
import io.github.stewseo.client.transport.JsonEndpoint;
import org.apache.http.client.utils.URLEncodedUtils;
import java.util.Map;
import java.util.function.Function;
public class SimpleEndpoint extends EndpointBase
implements JsonEndpoint {
private final JsonpDeserializer responseParser;
public SimpleEndpoint(
String id,
Function method,
Function requestUrl,
Function> queryParameters,
Function> headers,
boolean hasRequestBody,
JsonpDeserializer responseParser
) {
super(id, method, requestUrl, queryParameters, headers, hasRequestBody);
this.responseParser = responseParser;
}
@Override
public JsonpDeserializer responseDeserializer() {
return this.responseParser;
}
@Override
public JsonpDeserializer errorDeserializer(int statusCode) {
return ErrorResponse._DESERIALIZER;
}
public SimpleEndpoint withResponseDeserializer(
JsonpDeserializer newResponseParser
) {
System.out.println("id: " + id + " method: " + method + " request URL: " + requestUrl+ " queryParms: " + queryParameters);
return new SimpleEndpoint<>(
id,
method,
requestUrl,
queryParameters,
headers,
hasRequestBody,
newResponseParser
);
}
public static RuntimeException noPathTemplateFound(String what) {
return new RuntimeException("Could not find a request " + what + " with this set of properties. " +
"Please check the API documentation, or raise an issue if this should be a valid request.");
}
public static void pathEncode(String src, StringBuilder dest) {
// TODO: avoid dependency on HttpClient here (and use something more efficient)
dest.append(URLEncodedUtils.formatSegments(src).substring(1));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy