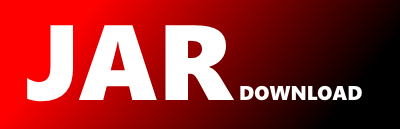
io.github.stewseo.client.util.Pair Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yelp-fusion-client Show documentation
Show all versions of yelp-fusion-client Show documentation
java client to build api objects, handle http transport, and parse/deserialize/serialize json to/from json
package io.github.stewseo.client.util;
import io.github.stewseo.client.json.JsonpDeserializer;
import io.github.stewseo.client.json.JsonpDeserializerBase;
import io.github.stewseo.client.json.JsonpMapper;
import io.github.stewseo.client.json.JsonpMappingException;
import io.github.stewseo.client.json.JsonpUtils;
import jakarta.json.stream.JsonParser;
import java.util.EnumSet;
import java.util.function.Function;
public class Pair {
private final K name;
private final V value;
public Pair(K name, V value) {
this.name = name;
this.value = value;
}
public K key() {
return this.name;
}
public V value() {
return this.value;
}
public static Pair of(K name, V value) {
return new Pair<>(name, value);
}
public static JsonpDeserializer> deserializer(
Function keyDeserializer, JsonpDeserializer valueDeserializer
) {
return new JsonpDeserializerBase<>(EnumSet.of(JsonParser.Event.START_OBJECT)) {
@Override
public Pair deserialize(JsonParser parser, JsonpMapper mapper, JsonParser.Event event) {
JsonpUtils.expectNextEvent(parser, JsonParser.Event.KEY_NAME);
String name = parser.getString();
try {
K key = keyDeserializer.apply(parser.getString());
V value = valueDeserializer.deserialize(parser, mapper);
JsonpUtils.expectNextEvent(parser, JsonParser.Event.END_OBJECT);
return new Pair<>(key, value);
} catch (Exception e) {
throw JsonpMappingException.from(e, null, name, parser);
}
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy