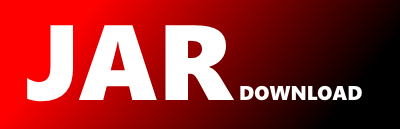
io.github.stewseo.client.yelpfusion.business.search.SearchBusinessResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yelp-fusion-client Show documentation
Show all versions of yelp-fusion-client Show documentation
java client to build api objects, handle http transport, and parse/deserialize/serialize json to/from json
package io.github.stewseo.client.yelpfusion.business.search;
import io.github.stewseo.client._types.aggregations.Aggregate;
import io.github.stewseo.client.json.JsonpDeserializable;
import io.github.stewseo.client.json.JsonpDeserializer;
import io.github.stewseo.client.json.JsonpMapper;
import io.github.stewseo.client.json.JsonpSerializable;
import io.github.stewseo.client.json.JsonpUtils;
import io.github.stewseo.client.json.ObjectBuilderDeserializer;
import io.github.stewseo.client.json.ObjectDeserializer;
import io.github.stewseo.client.util.ApiTypeHelper;
import io.github.stewseo.client.util.ObjectBuilder;
import io.github.stewseo.client.util.WithJsonObjectBuilderBase;
import io.github.stewseo.client.yelpfusion.business.Region;
import jakarta.json.stream.JsonGenerator;
import javax.annotation.Nullable;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
@JsonpDeserializable
public class SearchBusinessResponse implements JsonpSerializable {
private final List businesses;
@Nullable
private final Integer total;
@Nullable
private final Region region;
public List businesses() {
return this.businesses;
}
public Integer total() {
return this.total;
}
public Region region() {
return this.region;
}
protected SearchBusinessResponse(Builder builder) {
this.businesses = builder.businesses;
this.total = builder.total;
this.region = builder.region;
}
public void serialize(JsonGenerator generator, JsonpMapper mapper) {
generator.writeStartObject();
serializeInternal(generator, mapper);
generator.writeEnd();
}
protected void serializeInternal(JsonGenerator generator, JsonpMapper mapper) {
if(this.businesses != null) {
generator.writeKey("businesses");
generator.writeStartArray();
for(SearchBusiness business: businesses) {
business.serialize(generator, mapper);
}
generator.writeEnd();
}
if (this.total != null) {
generator.writeKey("total");
generator.write(this.total);
}
if (this.region != null) {
generator.writeKey("region");
region.serialize(generator, mapper);
}
}
public String toString() {
return JsonpUtils.toString(this);
}
public static class Builder extends WithJsonObjectBuilderBase
implements
ObjectBuilder {
private List businesses;
@Nullable
private Integer total;
@Nullable
private Region region;
public final Builder total(@Nullable Integer value) {
this.total = value;
return this;
}
public final Builder region(@Nullable Region value) {
this.region = value;
return this;
}
public final Builder businesses(List list) {
this.businesses = _listAddAll(this.businesses, list);
return this;
}
public final Builder businesses(SearchBusiness value, SearchBusiness... values) {
this.businesses = _listAdd(this.businesses, value, values);
return this;
}
public final Builder businesses(Function> fn) {
return businesses(fn.apply(new SearchBusiness.Builder()).build());
}
@Override
protected SearchBusinessResponse.Builder self() {
return this;
}
public SearchBusinessResponse build() {
_checkSingleUse();
return new SearchBusinessResponse(this);
}
}
public static final JsonpDeserializer _DESERIALIZER = ObjectBuilderDeserializer.lazy(SearchBusinessResponse.Builder::new,
SearchBusinessResponse::setupBusinessSearchResponse_Deserializer);
protected static void setupBusinessSearchResponse_Deserializer(ObjectDeserializer op) {
op.add(Builder::total,JsonpDeserializer.integerDeserializer(), "total");
op.add(Builder::businesses, JsonpDeserializer.arrayDeserializer(SearchBusiness._DESERIALIZER), "businesses");
op.add(Builder::region, Region._DESERIALIZER, "region");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy