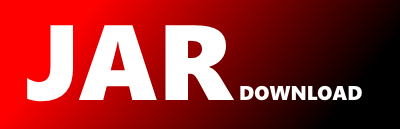
com.rapidapi.p.wordsapiv1.models.SynonymsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of words-api-sdk Show documentation
Show all versions of words-api-sdk Show documentation
This is a client SDK for words API
/*
* WordsAPILib
*
* This file was automatically generated by APIMATIC v3.0 ( https://www.apimatic.io ).
*/
package com.rapidapi.p.wordsapiv1.models;
import com.fasterxml.jackson.annotation.JsonGetter;
import com.fasterxml.jackson.annotation.JsonSetter;
import java.util.List;
/**
* This is a model class for SynonymsResponse type.
*/
public class SynonymsResponse {
private String word;
private List synonyms;
/**
* Default constructor.
*/
public SynonymsResponse() {
}
/**
* Initialization constructor.
* @param word String value for word.
* @param synonyms List of String value for synonyms.
*/
public SynonymsResponse(
String word,
List synonyms) {
this.word = word;
this.synonyms = synonyms;
}
/**
* Getter for Word.
* The word that is searched.
* @return Returns the String
*/
@JsonGetter("word")
public String getWord() {
return word;
}
/**
* Setter for Word.
* The word that is searched.
* @param word Value for String
*/
@JsonSetter("word")
public void setWord(String word) {
this.word = word;
}
/**
* Getter for Synonyms.
* The synonyms of the searched word.
* @return Returns the List of String
*/
@JsonGetter("synonyms")
public List getSynonyms() {
return synonyms;
}
/**
* Setter for Synonyms.
* The synonyms of the searched word.
* @param synonyms Value for List of String
*/
@JsonSetter("synonyms")
public void setSynonyms(List synonyms) {
this.synonyms = synonyms;
}
/**
* Converts this SynonymsResponse into string format.
* @return String representation of this class
*/
@Override
public String toString() {
return "SynonymsResponse [" + "word=" + word + ", synonyms=" + synonyms + "]";
}
/**
* Builds a new {@link SynonymsResponse.Builder} object.
* Creates the instance with the state of the current model.
* @return a new {@link SynonymsResponse.Builder} object
*/
public Builder toBuilder() {
Builder builder = new Builder(word, synonyms);
return builder;
}
/**
* Class to build instances of {@link SynonymsResponse}.
*/
public static class Builder {
private String word;
private List synonyms;
/**
* Initialization constructor.
*/
public Builder() {
}
/**
* Initialization constructor.
* @param word String value for word.
* @param synonyms List of String value for synonyms.
*/
public Builder(String word, List synonyms) {
this.word = word;
this.synonyms = synonyms;
}
/**
* Setter for word.
* @param word String value for word.
* @return Builder
*/
public Builder word(String word) {
this.word = word;
return this;
}
/**
* Setter for synonyms.
* @param synonyms List of String value for synonyms.
* @return Builder
*/
public Builder synonyms(List synonyms) {
this.synonyms = synonyms;
return this;
}
/**
* Builds a new {@link SynonymsResponse} object using the set fields.
* @return {@link SynonymsResponse}
*/
public SynonymsResponse build() {
return new SynonymsResponse(word, synonyms);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy