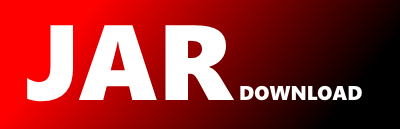
com.rapidapi.p.wordsapiv1.models.WordResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of words-api-sdk Show documentation
Show all versions of words-api-sdk Show documentation
This is a client SDK for words API
/*
* WordsAPILib
*
* This file was automatically generated by APIMATIC v3.0 ( https://www.apimatic.io ).
*/
package com.rapidapi.p.wordsapiv1.models;
import com.fasterxml.jackson.annotation.JsonGetter;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonSetter;
import java.util.List;
/**
* This is a model class for WordResponse type.
*/
public class WordResponse {
private String word;
private List results;
private Object pronunciation;
private Double frequency;
private SyllableDetails syllables;
/**
* Default constructor.
*/
public WordResponse() {
}
/**
* Initialization constructor.
* @param word String value for word.
* @param results List of WordDetails value for results.
* @param pronunciation Object value for pronunciation.
* @param frequency Double value for frequency.
* @param syllables SyllableDetails value for syllables.
*/
public WordResponse(
String word,
List results,
Object pronunciation,
Double frequency,
SyllableDetails syllables) {
this.word = word;
this.results = results;
this.pronunciation = pronunciation;
this.frequency = frequency;
this.syllables = syllables;
}
/**
* Getter for Word.
* The word that is searched.
* @return Returns the String
*/
@JsonGetter("word")
public String getWord() {
return word;
}
/**
* Setter for Word.
* The word that is searched.
* @param word Value for String
*/
@JsonSetter("word")
public void setWord(String word) {
this.word = word;
}
/**
* Getter for Results.
* This field contains detailed information of the word.
* @return Returns the List of WordDetails
*/
@JsonGetter("results")
public List getResults() {
return results;
}
/**
* Setter for Results.
* This field contains detailed information of the word.
* @param results Value for List of WordDetails
*/
@JsonSetter("results")
public void setResults(List results) {
this.results = results;
}
/**
* Getter for Pronunciation.
* This model contains pronunciation details of a specific word.
* @return Returns the Object
*/
@JsonGetter("pronunciation")
@JsonInclude(JsonInclude.Include.NON_NULL)
public Object getPronunciation() {
return pronunciation;
}
/**
* Setter for Pronunciation.
* This model contains pronunciation details of a specific word.
* @param pronunciation Value for Object
*/
@JsonSetter("pronunciation")
public void setPronunciation(Object pronunciation) {
this.pronunciation = pronunciation;
}
/**
* Getter for Frequency.
* The frequency of the word usage.
* @return Returns the Double
*/
@JsonGetter("frequency")
@JsonInclude(JsonInclude.Include.NON_NULL)
public Double getFrequency() {
return frequency;
}
/**
* Setter for Frequency.
* The frequency of the word usage.
* @param frequency Value for Double
*/
@JsonSetter("frequency")
public void setFrequency(Double frequency) {
this.frequency = frequency;
}
/**
* Getter for Syllables.
* This custom type contains the syllable details for word API.
* @return Returns the SyllableDetails
*/
@JsonGetter("syllables")
@JsonInclude(JsonInclude.Include.NON_NULL)
public SyllableDetails getSyllables() {
return syllables;
}
/**
* Setter for Syllables.
* This custom type contains the syllable details for word API.
* @param syllables Value for SyllableDetails
*/
@JsonSetter("syllables")
public void setSyllables(SyllableDetails syllables) {
this.syllables = syllables;
}
/**
* Converts this WordResponse into string format.
* @return String representation of this class
*/
@Override
public String toString() {
return "WordResponse [" + "word=" + word + ", results=" + results + ", pronunciation="
+ pronunciation + ", frequency=" + frequency + ", syllables=" + syllables + "]";
}
/**
* Builds a new {@link WordResponse.Builder} object.
* Creates the instance with the state of the current model.
* @return a new {@link WordResponse.Builder} object
*/
public Builder toBuilder() {
Builder builder = new Builder(word, results)
.pronunciation(getPronunciation())
.frequency(getFrequency())
.syllables(getSyllables());
return builder;
}
/**
* Class to build instances of {@link WordResponse}.
*/
public static class Builder {
private String word;
private List results;
private Object pronunciation;
private Double frequency;
private SyllableDetails syllables;
/**
* Initialization constructor.
*/
public Builder() {
}
/**
* Initialization constructor.
* @param word String value for word.
* @param results List of WordDetails value for results.
*/
public Builder(String word, List results) {
this.word = word;
this.results = results;
}
/**
* Setter for word.
* @param word String value for word.
* @return Builder
*/
public Builder word(String word) {
this.word = word;
return this;
}
/**
* Setter for results.
* @param results List of WordDetails value for results.
* @return Builder
*/
public Builder results(List results) {
this.results = results;
return this;
}
/**
* Setter for pronunciation.
* @param pronunciation Object value for pronunciation.
* @return Builder
*/
public Builder pronunciation(Object pronunciation) {
this.pronunciation = pronunciation;
return this;
}
/**
* Setter for frequency.
* @param frequency Double value for frequency.
* @return Builder
*/
public Builder frequency(Double frequency) {
this.frequency = frequency;
return this;
}
/**
* Setter for syllables.
* @param syllables SyllableDetails value for syllables.
* @return Builder
*/
public Builder syllables(SyllableDetails syllables) {
this.syllables = syllables;
return this;
}
/**
* Builds a new {@link WordResponse} object using the set fields.
* @return {@link WordResponse}
*/
public WordResponse build() {
return new WordResponse(word, results, pronunciation, frequency, syllables);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy