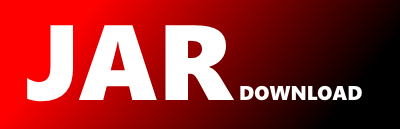
org.sugarcubes.cloner.ReflectionCopierProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sugar-cubes-cloner Show documentation
Show all versions of sugar-cubes-cloner Show documentation
Deep cloning of any objects
/*
* Copyright 2017-2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.sugarcubes.cloner;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Supplier;
/**
* Copier provider implementation.
*
* @author Maxim Butov
*/
public class ReflectionCopierProvider implements CopierProvider {
/**
* Object policy.
*/
private final CopyPolicy
© 2015 - 2025 Weber Informatics LLC | Privacy Policy