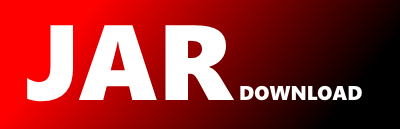
io.github.szhittech.Logc Maven / Gradle / Ivy
package io.github.szhittech;
import io.github.szhittech.format.FormattingTuple;
import io.github.szhittech.format.MessageFormatter;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
public class Logc {
public static final String LINE_BREAK = "\r\n";
public static void d(String message) {
sout(message);
}
public static void d(Throwable tr) {
sout(getThrowable(tr));
}
public static void d(String message, Throwable tr) {
String throwable = getThrowable(tr);
sout(String.format("%s %s",message,throwable));
}
public static void d(String format, Object... arguments) {
FormattingTuple tuple = MessageFormatter.arrayFormat(format,arguments);
sout(tuple.getMessage());
}
public static void i(String message) {
sout(message);
}
public static void i(Throwable tr) {
sout(getThrowable(tr));
}
public static void i(String message, Throwable tr) {
String throwable = getThrowable(tr);
sout(String.format("%s %s",message,throwable));
}
public static void i(String format, Object... arguments) {
FormattingTuple tuple = MessageFormatter.arrayFormat(format,arguments);
sout(tuple.getMessage());
}
private static void sout(String message){
String nameLineMethod = getNameLineMethod();
String timestamp = getTimestamp();
System.out.println(String.format("%s %s:%s",timestamp,nameLineMethod,message));
}
public static void e(String message) {
serr(message);
}
public static void e(Throwable tr) {
serr(getThrowable(tr));
}
public static void e(String message, Throwable tr) {
String throwable = getThrowable(tr);
serr(String.format("%s %s",message,throwable));
}
public static void e(String format, Object... arguments) {
FormattingTuple tuple = MessageFormatter.arrayFormat(format,arguments);
serr(tuple.getMessage());
}
private static void serr(String message){
String nameLineMethod = getNameLineMethod();
String timestamp = getTimestamp();
System.err.println(String.format("%s %s:%s",timestamp,nameLineMethod,message));
}
private static String getNameLineMethod() {
StackTraceElement[] callers = Thread.currentThread().getStackTrace();
StackTraceElement caller = callers[callers.length - 1];
String tag = "(%s:%d).%s"; // 占位符
String callerClazzName = caller.getFileName();
tag = String.format(tag, callerClazzName, caller.getLineNumber(), caller.getMethodName()); // 替换
return tag;
}
private static String getTimestamp(){
Date date = new Date();
SimpleDateFormat dateFormat = new SimpleDateFormat("", Locale.SIMPLIFIED_CHINESE);
dateFormat.applyPattern("yyyy-MM-dd HH:mm:ss");
String time = dateFormat.format(date);
return time;
}
private static String getThrowable(Throwable throwable) {
/* 打印异常 */
StringBuffer sb = new StringBuffer();
if (throwable != null) {
sb.append(LINE_BREAK);
StringWriter stringWriter = new StringWriter();
PrintWriter printWriter = new PrintWriter(stringWriter);
throwable.printStackTrace(printWriter);
sb.append(stringWriter.toString());
}
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy