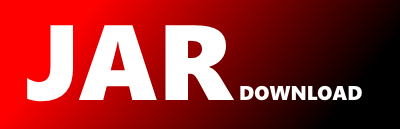
io.github.thewebcode.yplugin.utils.ItemFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of YPlugin Show documentation
Show all versions of YPlugin Show documentation
The Original Y Project by TheWebcode
package io.github.thewebcode.yplugin.utils;
import com.google.common.collect.Lists;
import org.bukkit.Material;
import org.bukkit.inventory.ItemStack;
import org.bukkit.inventory.meta.ItemMeta;
public class ItemFactory {
public static ItemStack create(Material material){
return new ItemStack(material);
}
public static ItemStack create(Material material, int amount){
return new ItemStack(material, amount);
}
public static ItemStack create(Material material, int amount, String name){
ItemStack itemStack = new ItemStack(material, amount);
ItemMeta itemMeta = itemStack.getItemMeta();
itemMeta.setDisplayName(name);
itemStack.setItemMeta(itemMeta);
return itemStack;
}
public static ItemStack create(Material material, int amount, String name, String... lore){
ItemStack itemStack = new ItemStack(material, amount);
ItemMeta itemMeta = itemStack.getItemMeta();
itemMeta.setDisplayName(name);
itemMeta.setLore(Lists.newArrayList(lore));
itemStack.setItemMeta(itemMeta);
return itemStack;
}
public static ItemStack create(Material material, int amount, String name, Iterable lore){
ItemStack itemStack = new ItemStack(material, amount);
ItemMeta itemMeta = itemStack.getItemMeta();
itemMeta.setDisplayName(name);
itemMeta.setLore(Lists.newArrayList(lore));
itemStack.setItemMeta(itemMeta);
return itemStack;
}
public static ItemStack create(Material material, int amount, String name, String lore){
ItemStack itemStack = new ItemStack(material, amount);
ItemMeta itemMeta = itemStack.getItemMeta();
itemMeta.setDisplayName(name);
itemMeta.setLore(Lists.newArrayList(lore));
itemStack.setItemMeta(itemMeta);
return itemStack;
}
public static ItemStack create(Material material, int amount, String name, boolean unbreakable){
ItemStack itemStack = new ItemStack(material, amount);
ItemMeta itemMeta = itemStack.getItemMeta();
itemMeta.setDisplayName(name);
itemMeta.setUnbreakable(unbreakable);
itemStack.setItemMeta(itemMeta);
return itemStack;
}
public static ItemStack create(Material material, int amount, String name, boolean unbreakable, String... lore){
ItemStack itemStack = new ItemStack(material, amount);
ItemMeta itemMeta = itemStack.getItemMeta();
itemMeta.setDisplayName(name);
itemMeta.setLore(Lists.newArrayList(lore));
itemMeta.setUnbreakable(unbreakable);
itemStack.setItemMeta(itemMeta);
return itemStack;
}
public static ItemStack create(Material material, int amount, String name, boolean unbreakable, Iterable lore){
ItemStack itemStack = new ItemStack(material, amount);
ItemMeta itemMeta = itemStack.getItemMeta();
itemMeta.setDisplayName(name);
itemMeta.setLore(Lists.newArrayList(lore));
itemMeta.setUnbreakable(unbreakable);
itemStack.setItemMeta(itemMeta);
return itemStack;
}
public static ItemStack createSkull(String name, String owner){
ItemStack itemStack = new ItemStack(Material.PLAYER_HEAD);
ItemMeta itemMeta = itemStack.getItemMeta();
itemMeta.setDisplayName(name);
itemStack.setItemMeta(itemMeta);
return itemStack;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy