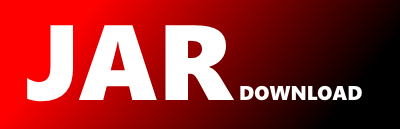
com.tidal.wave.verification.criteria.Criteria Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wave Show documentation
Show all versions of wave Show documentation
Automation Code Repository
package com.tidal.wave.verification.criteria;
import com.tidal.wave.verification.conditions.Verification;
import java.util.function.Supplier;
public abstract class Criteria implements Verification {
/**
* Condition to be satisfied that the element should be VISIBLE.
* Throws TestAssertionError if the condition is not satisfied.
*/
public static final Supplier visible = Criteria::visible;
/**
* Condition to be satisfied that the element should be NOT VISIBLE.
* Throws TestAssertionError if the condition is not satisfied.
*/
public static final Supplier notVisible = Criteria::notVisible;
/**
* Condition to be satisfied that the element should be PRESENT.
* Throws TestAssertionError if the condition is not satisfied.
*/
public static final Supplier present = Criteria::present;
/**
* Condition to be satisfied that the element should be NOT PRESENT.
* Throws TestAssertionError if the condition is not satisfied.
*/
public static final Supplier notPresent = Criteria::notPresent;
/**
* Condition to be satisfied that the element should be ENABLED.
* Throws TestAssertionError if the condition is not satisfied.
*/
public static final Supplier enabled = Criteria::enabled;
/**
* Condition to be satisfied that the element should be NOT ENABLED.
* Throws TestAssertionError if the condition is not satisfied.
*/
public static final Supplier notEnabled = Criteria::notEnabled;
/**
* Please use static variable 'visible'
* Condition to be satisfied that the element should be VISIBLE.
* Throws TestAssertionError if the condition is not satisfied.
*
* @return Instance of Visible Criteria class
*/
private static Criteria visible() {
return new VisibleCriteria();
}
/**
* Please use static variable 'notVisible'
* Condition to be satisfied that the element should be NOT VISIBLE.
* Throws TestAssertionError if the condition is not satisfied.
*
* @return Instance of Invisible Criteria class.
*/
private static Criteria notVisible() {
return new InvisibleCriteria();
}
/**
* Please use static variable 'present'
* Condition to be satisfied that the element should be PRESENT.
* Throws TestAssertionError if the condition is not satisfied.
*
* @return Instance of Present Criteria class.
*/
private static Criteria present() {
return new PresentCriteria();
}
/**
* Please use static variable 'notPresent'
* Condition to be satisfied that the element should be NOT PRESENT.
* Throws TestAssertionError if the condition is not satisfied.
*
* @return Instance of NotPresent Criteria class
*/
private static Criteria notPresent() {
return new NotPresentCriteria();
}
/**
* Please use static variable 'enabled'
* Condition to be satisfied that the element should be ENABLED.
* Throws TestAssertionError if the condition is not satisfied.
*
* @return Instance of Element Enabled Criteria class.
*/
private static Criteria enabled() {
return new ElementEnabled();
}
/**
* Please use static variable 'notEnabled'
* Condition to be satisfied that the element should be NOT ENABLED.
* Throws TestAssertionError if the condition is not satisfied.
*
* @return Instance of Element Disabled Criteria class.
*/
private static Criteria notEnabled() {
return new ElementDisabled();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy