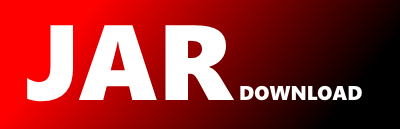
toolgood.algorithm.mathNet.Distributions.Binomial Maven / Gradle / Ivy
package toolgood.algorithm.mathNet.Distributions;
import toolgood.algorithm.mathNet.SpecialFunctions;
///
/// Discrete Univariate Binomial distribution.
/// For details about this distribution, see
/// Wikipedia - Binomial distribution.
///
///
/// The distribution is parameterized by a probability (between 0.0 and 1.0).
///
public class Binomial {
///
/// Computes the probability mass (PMF) at k, i.e. P(X = k).
///
/// The location in the domain where we want to evaluate the
/// probability mass function.
/// The success probability (p) in each trial. Range: 0 ≤ p ≤
/// 1.
/// The number of trials (n). Range: n ≥ 0.
/// the probability mass at location .
public static double PMF(double p, int n, int k) {
// if (!(p >= 0.0 && p <= 1.0 && n >= 0)) {
// throw new ArgumentException("InvalidDistributionParameters");
// }
if (k < 0 || k > n) {
return 0.0;
}
if (p == 0.0) {
return k == 0 ? 1.0 : 0.0;
}
if (p == 1.0) {
return k == n ? 1.0 : 0.0;
}
return Math.exp(SpecialFunctions.BinomialLn(n, k) + (k * Math.log(p)) + ((n - k) * Math.log(1.0 - p)));
}
///
/// Computes the cumulative distribution (CDF) of the distribution at x, i.e.
/// P(X ≤ x).
///
/// The location at which to compute the cumulative distribution
/// function.
/// The success probability (p) in each trial. Range: 0 ≤ p ≤
/// 1.
/// The number of trials (n). Range: n ≥ 0.
/// the cumulative distribution at location .
/////
public static double CDF(double p, int n, double x) {
// if (!(p >= 0.0 && p <= 1.0 && n >= 0)) {
// throw new ArgumentException("InvalidDistributionParameters");
// }
if (x < 0.0) {
return 0.0;
}
if (x > n) {
return 1.0;
}
double k = Math.floor(x);
return SpecialFunctions.BetaRegularized(n - k, k + 1, 1 - p);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy