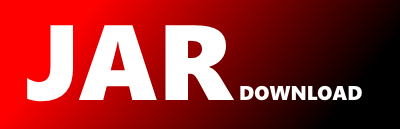
org.tron.p2p.protos.Connect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libp2p Show documentation
Show all versions of libp2p Show documentation
libp2p is a p2p network SDK implemented in java language.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: Connect.proto
// Protobuf Java Version: 3.25.5
package org.tron.p2p.protos;
public final class Connect {
private Connect() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code DisconnectReason}
*/
public enum DisconnectReason
implements com.google.protobuf.ProtocolMessageEnum {
/**
* PEER_QUITING = 0;
*/
PEER_QUITING(0),
/**
* BAD_PROTOCOL = 1;
*/
BAD_PROTOCOL(1),
/**
* TOO_MANY_PEERS = 2;
*/
TOO_MANY_PEERS(2),
/**
* DUPLICATE_PEER = 3;
*/
DUPLICATE_PEER(3),
/**
* DIFFERENT_VERSION = 4;
*/
DIFFERENT_VERSION(4),
/**
* RANDOM_ELIMINATION = 5;
*/
RANDOM_ELIMINATION(5),
/**
* EMPTY_MESSAGE = 6;
*/
EMPTY_MESSAGE(6),
/**
* PING_TIMEOUT = 7;
*/
PING_TIMEOUT(7),
/**
* DISCOVER_MODE = 8;
*/
DISCOVER_MODE(8),
/**
*
*DETECT_COMPLETE = 0x09;
*
*
* NO_SUCH_MESSAGE = 10;
*/
NO_SUCH_MESSAGE(10),
/**
* BAD_MESSAGE = 11;
*/
BAD_MESSAGE(11),
/**
* TOO_MANY_PEERS_WITH_SAME_IP = 12;
*/
TOO_MANY_PEERS_WITH_SAME_IP(12),
/**
* RECENT_DISCONNECT = 13;
*/
RECENT_DISCONNECT(13),
/**
* DUP_HANDSHAKE = 14;
*/
DUP_HANDSHAKE(14),
/**
* UNKNOWN = 255;
*/
UNKNOWN(255),
UNRECOGNIZED(-1),
;
/**
* PEER_QUITING = 0;
*/
public static final int PEER_QUITING_VALUE = 0;
/**
* BAD_PROTOCOL = 1;
*/
public static final int BAD_PROTOCOL_VALUE = 1;
/**
* TOO_MANY_PEERS = 2;
*/
public static final int TOO_MANY_PEERS_VALUE = 2;
/**
* DUPLICATE_PEER = 3;
*/
public static final int DUPLICATE_PEER_VALUE = 3;
/**
* DIFFERENT_VERSION = 4;
*/
public static final int DIFFERENT_VERSION_VALUE = 4;
/**
* RANDOM_ELIMINATION = 5;
*/
public static final int RANDOM_ELIMINATION_VALUE = 5;
/**
* EMPTY_MESSAGE = 6;
*/
public static final int EMPTY_MESSAGE_VALUE = 6;
/**
* PING_TIMEOUT = 7;
*/
public static final int PING_TIMEOUT_VALUE = 7;
/**
* DISCOVER_MODE = 8;
*/
public static final int DISCOVER_MODE_VALUE = 8;
/**
*
*DETECT_COMPLETE = 0x09;
*
*
* NO_SUCH_MESSAGE = 10;
*/
public static final int NO_SUCH_MESSAGE_VALUE = 10;
/**
* BAD_MESSAGE = 11;
*/
public static final int BAD_MESSAGE_VALUE = 11;
/**
* TOO_MANY_PEERS_WITH_SAME_IP = 12;
*/
public static final int TOO_MANY_PEERS_WITH_SAME_IP_VALUE = 12;
/**
* RECENT_DISCONNECT = 13;
*/
public static final int RECENT_DISCONNECT_VALUE = 13;
/**
* DUP_HANDSHAKE = 14;
*/
public static final int DUP_HANDSHAKE_VALUE = 14;
/**
* UNKNOWN = 255;
*/
public static final int UNKNOWN_VALUE = 255;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DisconnectReason valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static DisconnectReason forNumber(int value) {
switch (value) {
case 0: return PEER_QUITING;
case 1: return BAD_PROTOCOL;
case 2: return TOO_MANY_PEERS;
case 3: return DUPLICATE_PEER;
case 4: return DIFFERENT_VERSION;
case 5: return RANDOM_ELIMINATION;
case 6: return EMPTY_MESSAGE;
case 7: return PING_TIMEOUT;
case 8: return DISCOVER_MODE;
case 10: return NO_SUCH_MESSAGE;
case 11: return BAD_MESSAGE;
case 12: return TOO_MANY_PEERS_WITH_SAME_IP;
case 13: return RECENT_DISCONNECT;
case 14: return DUP_HANDSHAKE;
case 255: return UNKNOWN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
DisconnectReason> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public DisconnectReason findValueByNumber(int number) {
return DisconnectReason.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.tron.p2p.protos.Connect.getDescriptor().getEnumTypes().get(0);
}
private static final DisconnectReason[] VALUES = values();
public static DisconnectReason valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private DisconnectReason(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:DisconnectReason)
}
public interface KeepAliveMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:KeepAliveMessage)
com.google.protobuf.MessageOrBuilder {
/**
* int64 timestamp = 1;
* @return The timestamp.
*/
long getTimestamp();
}
/**
* Protobuf type {@code KeepAliveMessage}
*/
public static final class KeepAliveMessage extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:KeepAliveMessage)
KeepAliveMessageOrBuilder {
private static final long serialVersionUID = 0L;
// Use KeepAliveMessage.newBuilder() to construct.
private KeepAliveMessage(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private KeepAliveMessage() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new KeepAliveMessage();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tron.p2p.protos.Connect.internal_static_KeepAliveMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tron.p2p.protos.Connect.internal_static_KeepAliveMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tron.p2p.protos.Connect.KeepAliveMessage.class, org.tron.p2p.protos.Connect.KeepAliveMessage.Builder.class);
}
public static final int TIMESTAMP_FIELD_NUMBER = 1;
private long timestamp_ = 0L;
/**
* int64 timestamp = 1;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (timestamp_ != 0L) {
output.writeInt64(1, timestamp_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (timestamp_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, timestamp_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tron.p2p.protos.Connect.KeepAliveMessage)) {
return super.equals(obj);
}
org.tron.p2p.protos.Connect.KeepAliveMessage other = (org.tron.p2p.protos.Connect.KeepAliveMessage) obj;
if (getTimestamp()
!= other.getTimestamp()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tron.p2p.protos.Connect.KeepAliveMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.KeepAliveMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.KeepAliveMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.KeepAliveMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.KeepAliveMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.KeepAliveMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.KeepAliveMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.KeepAliveMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.KeepAliveMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.KeepAliveMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.KeepAliveMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.KeepAliveMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tron.p2p.protos.Connect.KeepAliveMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code KeepAliveMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:KeepAliveMessage)
org.tron.p2p.protos.Connect.KeepAliveMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tron.p2p.protos.Connect.internal_static_KeepAliveMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tron.p2p.protos.Connect.internal_static_KeepAliveMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tron.p2p.protos.Connect.KeepAliveMessage.class, org.tron.p2p.protos.Connect.KeepAliveMessage.Builder.class);
}
// Construct using org.tron.p2p.protos.Connect.KeepAliveMessage.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
timestamp_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tron.p2p.protos.Connect.internal_static_KeepAliveMessage_descriptor;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.KeepAliveMessage getDefaultInstanceForType() {
return org.tron.p2p.protos.Connect.KeepAliveMessage.getDefaultInstance();
}
@java.lang.Override
public org.tron.p2p.protos.Connect.KeepAliveMessage build() {
org.tron.p2p.protos.Connect.KeepAliveMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.KeepAliveMessage buildPartial() {
org.tron.p2p.protos.Connect.KeepAliveMessage result = new org.tron.p2p.protos.Connect.KeepAliveMessage(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.tron.p2p.protos.Connect.KeepAliveMessage result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.timestamp_ = timestamp_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tron.p2p.protos.Connect.KeepAliveMessage) {
return mergeFrom((org.tron.p2p.protos.Connect.KeepAliveMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tron.p2p.protos.Connect.KeepAliveMessage other) {
if (other == org.tron.p2p.protos.Connect.KeepAliveMessage.getDefaultInstance()) return this;
if (other.getTimestamp() != 0L) {
setTimestamp(other.getTimestamp());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
timestamp_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long timestamp_ ;
/**
* int64 timestamp = 1;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
/**
* int64 timestamp = 1;
* @param value The timestamp to set.
* @return This builder for chaining.
*/
public Builder setTimestamp(long value) {
timestamp_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* int64 timestamp = 1;
* @return This builder for chaining.
*/
public Builder clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00000001);
timestamp_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:KeepAliveMessage)
}
// @@protoc_insertion_point(class_scope:KeepAliveMessage)
private static final org.tron.p2p.protos.Connect.KeepAliveMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tron.p2p.protos.Connect.KeepAliveMessage();
}
public static org.tron.p2p.protos.Connect.KeepAliveMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public KeepAliveMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.KeepAliveMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HelloMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:HelloMessage)
com.google.protobuf.MessageOrBuilder {
/**
* .Endpoint from = 1;
* @return Whether the from field is set.
*/
boolean hasFrom();
/**
* .Endpoint from = 1;
* @return The from.
*/
org.tron.p2p.protos.Discover.Endpoint getFrom();
/**
* .Endpoint from = 1;
*/
org.tron.p2p.protos.Discover.EndpointOrBuilder getFromOrBuilder();
/**
* int32 network_id = 2;
* @return The networkId.
*/
int getNetworkId();
/**
* int32 code = 3;
* @return The code.
*/
int getCode();
/**
* int64 timestamp = 4;
* @return The timestamp.
*/
long getTimestamp();
/**
* int32 version = 5;
* @return The version.
*/
int getVersion();
}
/**
* Protobuf type {@code HelloMessage}
*/
public static final class HelloMessage extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:HelloMessage)
HelloMessageOrBuilder {
private static final long serialVersionUID = 0L;
// Use HelloMessage.newBuilder() to construct.
private HelloMessage(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HelloMessage() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HelloMessage();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tron.p2p.protos.Connect.internal_static_HelloMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tron.p2p.protos.Connect.internal_static_HelloMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tron.p2p.protos.Connect.HelloMessage.class, org.tron.p2p.protos.Connect.HelloMessage.Builder.class);
}
private int bitField0_;
public static final int FROM_FIELD_NUMBER = 1;
private org.tron.p2p.protos.Discover.Endpoint from_;
/**
* .Endpoint from = 1;
* @return Whether the from field is set.
*/
@java.lang.Override
public boolean hasFrom() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .Endpoint from = 1;
* @return The from.
*/
@java.lang.Override
public org.tron.p2p.protos.Discover.Endpoint getFrom() {
return from_ == null ? org.tron.p2p.protos.Discover.Endpoint.getDefaultInstance() : from_;
}
/**
* .Endpoint from = 1;
*/
@java.lang.Override
public org.tron.p2p.protos.Discover.EndpointOrBuilder getFromOrBuilder() {
return from_ == null ? org.tron.p2p.protos.Discover.Endpoint.getDefaultInstance() : from_;
}
public static final int NETWORK_ID_FIELD_NUMBER = 2;
private int networkId_ = 0;
/**
* int32 network_id = 2;
* @return The networkId.
*/
@java.lang.Override
public int getNetworkId() {
return networkId_;
}
public static final int CODE_FIELD_NUMBER = 3;
private int code_ = 0;
/**
* int32 code = 3;
* @return The code.
*/
@java.lang.Override
public int getCode() {
return code_;
}
public static final int TIMESTAMP_FIELD_NUMBER = 4;
private long timestamp_ = 0L;
/**
* int64 timestamp = 4;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
public static final int VERSION_FIELD_NUMBER = 5;
private int version_ = 0;
/**
* int32 version = 5;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getFrom());
}
if (networkId_ != 0) {
output.writeInt32(2, networkId_);
}
if (code_ != 0) {
output.writeInt32(3, code_);
}
if (timestamp_ != 0L) {
output.writeInt64(4, timestamp_);
}
if (version_ != 0) {
output.writeInt32(5, version_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getFrom());
}
if (networkId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, networkId_);
}
if (code_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, code_);
}
if (timestamp_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, timestamp_);
}
if (version_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, version_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tron.p2p.protos.Connect.HelloMessage)) {
return super.equals(obj);
}
org.tron.p2p.protos.Connect.HelloMessage other = (org.tron.p2p.protos.Connect.HelloMessage) obj;
if (hasFrom() != other.hasFrom()) return false;
if (hasFrom()) {
if (!getFrom()
.equals(other.getFrom())) return false;
}
if (getNetworkId()
!= other.getNetworkId()) return false;
if (getCode()
!= other.getCode()) return false;
if (getTimestamp()
!= other.getTimestamp()) return false;
if (getVersion()
!= other.getVersion()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasFrom()) {
hash = (37 * hash) + FROM_FIELD_NUMBER;
hash = (53 * hash) + getFrom().hashCode();
}
hash = (37 * hash) + NETWORK_ID_FIELD_NUMBER;
hash = (53 * hash) + getNetworkId();
hash = (37 * hash) + CODE_FIELD_NUMBER;
hash = (53 * hash) + getCode();
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tron.p2p.protos.Connect.HelloMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.HelloMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.HelloMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.HelloMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.HelloMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.HelloMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.HelloMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.HelloMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.HelloMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.HelloMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.HelloMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.HelloMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tron.p2p.protos.Connect.HelloMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code HelloMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:HelloMessage)
org.tron.p2p.protos.Connect.HelloMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tron.p2p.protos.Connect.internal_static_HelloMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tron.p2p.protos.Connect.internal_static_HelloMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tron.p2p.protos.Connect.HelloMessage.class, org.tron.p2p.protos.Connect.HelloMessage.Builder.class);
}
// Construct using org.tron.p2p.protos.Connect.HelloMessage.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFromFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
from_ = null;
if (fromBuilder_ != null) {
fromBuilder_.dispose();
fromBuilder_ = null;
}
networkId_ = 0;
code_ = 0;
timestamp_ = 0L;
version_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tron.p2p.protos.Connect.internal_static_HelloMessage_descriptor;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.HelloMessage getDefaultInstanceForType() {
return org.tron.p2p.protos.Connect.HelloMessage.getDefaultInstance();
}
@java.lang.Override
public org.tron.p2p.protos.Connect.HelloMessage build() {
org.tron.p2p.protos.Connect.HelloMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.HelloMessage buildPartial() {
org.tron.p2p.protos.Connect.HelloMessage result = new org.tron.p2p.protos.Connect.HelloMessage(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.tron.p2p.protos.Connect.HelloMessage result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.from_ = fromBuilder_ == null
? from_
: fromBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.networkId_ = networkId_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.code_ = code_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.timestamp_ = timestamp_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.version_ = version_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tron.p2p.protos.Connect.HelloMessage) {
return mergeFrom((org.tron.p2p.protos.Connect.HelloMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tron.p2p.protos.Connect.HelloMessage other) {
if (other == org.tron.p2p.protos.Connect.HelloMessage.getDefaultInstance()) return this;
if (other.hasFrom()) {
mergeFrom(other.getFrom());
}
if (other.getNetworkId() != 0) {
setNetworkId(other.getNetworkId());
}
if (other.getCode() != 0) {
setCode(other.getCode());
}
if (other.getTimestamp() != 0L) {
setTimestamp(other.getTimestamp());
}
if (other.getVersion() != 0) {
setVersion(other.getVersion());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getFromFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
networkId_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
code_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
timestamp_ = input.readInt64();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40: {
version_ = input.readInt32();
bitField0_ |= 0x00000010;
break;
} // case 40
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private org.tron.p2p.protos.Discover.Endpoint from_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tron.p2p.protos.Discover.Endpoint, org.tron.p2p.protos.Discover.Endpoint.Builder, org.tron.p2p.protos.Discover.EndpointOrBuilder> fromBuilder_;
/**
* .Endpoint from = 1;
* @return Whether the from field is set.
*/
public boolean hasFrom() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .Endpoint from = 1;
* @return The from.
*/
public org.tron.p2p.protos.Discover.Endpoint getFrom() {
if (fromBuilder_ == null) {
return from_ == null ? org.tron.p2p.protos.Discover.Endpoint.getDefaultInstance() : from_;
} else {
return fromBuilder_.getMessage();
}
}
/**
* .Endpoint from = 1;
*/
public Builder setFrom(org.tron.p2p.protos.Discover.Endpoint value) {
if (fromBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
from_ = value;
} else {
fromBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .Endpoint from = 1;
*/
public Builder setFrom(
org.tron.p2p.protos.Discover.Endpoint.Builder builderForValue) {
if (fromBuilder_ == null) {
from_ = builderForValue.build();
} else {
fromBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .Endpoint from = 1;
*/
public Builder mergeFrom(org.tron.p2p.protos.Discover.Endpoint value) {
if (fromBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
from_ != null &&
from_ != org.tron.p2p.protos.Discover.Endpoint.getDefaultInstance()) {
getFromBuilder().mergeFrom(value);
} else {
from_ = value;
}
} else {
fromBuilder_.mergeFrom(value);
}
if (from_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
* .Endpoint from = 1;
*/
public Builder clearFrom() {
bitField0_ = (bitField0_ & ~0x00000001);
from_ = null;
if (fromBuilder_ != null) {
fromBuilder_.dispose();
fromBuilder_ = null;
}
onChanged();
return this;
}
/**
* .Endpoint from = 1;
*/
public org.tron.p2p.protos.Discover.Endpoint.Builder getFromBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getFromFieldBuilder().getBuilder();
}
/**
* .Endpoint from = 1;
*/
public org.tron.p2p.protos.Discover.EndpointOrBuilder getFromOrBuilder() {
if (fromBuilder_ != null) {
return fromBuilder_.getMessageOrBuilder();
} else {
return from_ == null ?
org.tron.p2p.protos.Discover.Endpoint.getDefaultInstance() : from_;
}
}
/**
* .Endpoint from = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tron.p2p.protos.Discover.Endpoint, org.tron.p2p.protos.Discover.Endpoint.Builder, org.tron.p2p.protos.Discover.EndpointOrBuilder>
getFromFieldBuilder() {
if (fromBuilder_ == null) {
fromBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tron.p2p.protos.Discover.Endpoint, org.tron.p2p.protos.Discover.Endpoint.Builder, org.tron.p2p.protos.Discover.EndpointOrBuilder>(
getFrom(),
getParentForChildren(),
isClean());
from_ = null;
}
return fromBuilder_;
}
private int networkId_ ;
/**
* int32 network_id = 2;
* @return The networkId.
*/
@java.lang.Override
public int getNetworkId() {
return networkId_;
}
/**
* int32 network_id = 2;
* @param value The networkId to set.
* @return This builder for chaining.
*/
public Builder setNetworkId(int value) {
networkId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 network_id = 2;
* @return This builder for chaining.
*/
public Builder clearNetworkId() {
bitField0_ = (bitField0_ & ~0x00000002);
networkId_ = 0;
onChanged();
return this;
}
private int code_ ;
/**
* int32 code = 3;
* @return The code.
*/
@java.lang.Override
public int getCode() {
return code_;
}
/**
* int32 code = 3;
* @param value The code to set.
* @return This builder for chaining.
*/
public Builder setCode(int value) {
code_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 code = 3;
* @return This builder for chaining.
*/
public Builder clearCode() {
bitField0_ = (bitField0_ & ~0x00000004);
code_ = 0;
onChanged();
return this;
}
private long timestamp_ ;
/**
* int64 timestamp = 4;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
/**
* int64 timestamp = 4;
* @param value The timestamp to set.
* @return This builder for chaining.
*/
public Builder setTimestamp(long value) {
timestamp_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* int64 timestamp = 4;
* @return This builder for chaining.
*/
public Builder clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00000008);
timestamp_ = 0L;
onChanged();
return this;
}
private int version_ ;
/**
* int32 version = 5;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
/**
* int32 version = 5;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(int value) {
version_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* int32 version = 5;
* @return This builder for chaining.
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000010);
version_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:HelloMessage)
}
// @@protoc_insertion_point(class_scope:HelloMessage)
private static final org.tron.p2p.protos.Connect.HelloMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tron.p2p.protos.Connect.HelloMessage();
}
public static org.tron.p2p.protos.Connect.HelloMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HelloMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.HelloMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StatusMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:StatusMessage)
com.google.protobuf.MessageOrBuilder {
/**
* .Endpoint from = 1;
* @return Whether the from field is set.
*/
boolean hasFrom();
/**
* .Endpoint from = 1;
* @return The from.
*/
org.tron.p2p.protos.Discover.Endpoint getFrom();
/**
* .Endpoint from = 1;
*/
org.tron.p2p.protos.Discover.EndpointOrBuilder getFromOrBuilder();
/**
* int32 version = 2;
* @return The version.
*/
int getVersion();
/**
* int32 network_id = 3;
* @return The networkId.
*/
int getNetworkId();
/**
* int32 maxConnections = 4;
* @return The maxConnections.
*/
int getMaxConnections();
/**
* int32 currentConnections = 5;
* @return The currentConnections.
*/
int getCurrentConnections();
/**
* int64 timestamp = 6;
* @return The timestamp.
*/
long getTimestamp();
}
/**
* Protobuf type {@code StatusMessage}
*/
public static final class StatusMessage extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:StatusMessage)
StatusMessageOrBuilder {
private static final long serialVersionUID = 0L;
// Use StatusMessage.newBuilder() to construct.
private StatusMessage(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StatusMessage() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StatusMessage();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tron.p2p.protos.Connect.internal_static_StatusMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tron.p2p.protos.Connect.internal_static_StatusMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tron.p2p.protos.Connect.StatusMessage.class, org.tron.p2p.protos.Connect.StatusMessage.Builder.class);
}
private int bitField0_;
public static final int FROM_FIELD_NUMBER = 1;
private org.tron.p2p.protos.Discover.Endpoint from_;
/**
* .Endpoint from = 1;
* @return Whether the from field is set.
*/
@java.lang.Override
public boolean hasFrom() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .Endpoint from = 1;
* @return The from.
*/
@java.lang.Override
public org.tron.p2p.protos.Discover.Endpoint getFrom() {
return from_ == null ? org.tron.p2p.protos.Discover.Endpoint.getDefaultInstance() : from_;
}
/**
* .Endpoint from = 1;
*/
@java.lang.Override
public org.tron.p2p.protos.Discover.EndpointOrBuilder getFromOrBuilder() {
return from_ == null ? org.tron.p2p.protos.Discover.Endpoint.getDefaultInstance() : from_;
}
public static final int VERSION_FIELD_NUMBER = 2;
private int version_ = 0;
/**
* int32 version = 2;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
public static final int NETWORK_ID_FIELD_NUMBER = 3;
private int networkId_ = 0;
/**
* int32 network_id = 3;
* @return The networkId.
*/
@java.lang.Override
public int getNetworkId() {
return networkId_;
}
public static final int MAXCONNECTIONS_FIELD_NUMBER = 4;
private int maxConnections_ = 0;
/**
* int32 maxConnections = 4;
* @return The maxConnections.
*/
@java.lang.Override
public int getMaxConnections() {
return maxConnections_;
}
public static final int CURRENTCONNECTIONS_FIELD_NUMBER = 5;
private int currentConnections_ = 0;
/**
* int32 currentConnections = 5;
* @return The currentConnections.
*/
@java.lang.Override
public int getCurrentConnections() {
return currentConnections_;
}
public static final int TIMESTAMP_FIELD_NUMBER = 6;
private long timestamp_ = 0L;
/**
* int64 timestamp = 6;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getFrom());
}
if (version_ != 0) {
output.writeInt32(2, version_);
}
if (networkId_ != 0) {
output.writeInt32(3, networkId_);
}
if (maxConnections_ != 0) {
output.writeInt32(4, maxConnections_);
}
if (currentConnections_ != 0) {
output.writeInt32(5, currentConnections_);
}
if (timestamp_ != 0L) {
output.writeInt64(6, timestamp_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getFrom());
}
if (version_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, version_);
}
if (networkId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, networkId_);
}
if (maxConnections_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, maxConnections_);
}
if (currentConnections_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, currentConnections_);
}
if (timestamp_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(6, timestamp_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tron.p2p.protos.Connect.StatusMessage)) {
return super.equals(obj);
}
org.tron.p2p.protos.Connect.StatusMessage other = (org.tron.p2p.protos.Connect.StatusMessage) obj;
if (hasFrom() != other.hasFrom()) return false;
if (hasFrom()) {
if (!getFrom()
.equals(other.getFrom())) return false;
}
if (getVersion()
!= other.getVersion()) return false;
if (getNetworkId()
!= other.getNetworkId()) return false;
if (getMaxConnections()
!= other.getMaxConnections()) return false;
if (getCurrentConnections()
!= other.getCurrentConnections()) return false;
if (getTimestamp()
!= other.getTimestamp()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasFrom()) {
hash = (37 * hash) + FROM_FIELD_NUMBER;
hash = (53 * hash) + getFrom().hashCode();
}
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion();
hash = (37 * hash) + NETWORK_ID_FIELD_NUMBER;
hash = (53 * hash) + getNetworkId();
hash = (37 * hash) + MAXCONNECTIONS_FIELD_NUMBER;
hash = (53 * hash) + getMaxConnections();
hash = (37 * hash) + CURRENTCONNECTIONS_FIELD_NUMBER;
hash = (53 * hash) + getCurrentConnections();
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tron.p2p.protos.Connect.StatusMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.StatusMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.StatusMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.StatusMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.StatusMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.StatusMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.StatusMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.StatusMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.StatusMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.StatusMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.StatusMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.StatusMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tron.p2p.protos.Connect.StatusMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code StatusMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:StatusMessage)
org.tron.p2p.protos.Connect.StatusMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tron.p2p.protos.Connect.internal_static_StatusMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tron.p2p.protos.Connect.internal_static_StatusMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tron.p2p.protos.Connect.StatusMessage.class, org.tron.p2p.protos.Connect.StatusMessage.Builder.class);
}
// Construct using org.tron.p2p.protos.Connect.StatusMessage.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFromFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
from_ = null;
if (fromBuilder_ != null) {
fromBuilder_.dispose();
fromBuilder_ = null;
}
version_ = 0;
networkId_ = 0;
maxConnections_ = 0;
currentConnections_ = 0;
timestamp_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tron.p2p.protos.Connect.internal_static_StatusMessage_descriptor;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.StatusMessage getDefaultInstanceForType() {
return org.tron.p2p.protos.Connect.StatusMessage.getDefaultInstance();
}
@java.lang.Override
public org.tron.p2p.protos.Connect.StatusMessage build() {
org.tron.p2p.protos.Connect.StatusMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.StatusMessage buildPartial() {
org.tron.p2p.protos.Connect.StatusMessage result = new org.tron.p2p.protos.Connect.StatusMessage(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.tron.p2p.protos.Connect.StatusMessage result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.from_ = fromBuilder_ == null
? from_
: fromBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.version_ = version_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.networkId_ = networkId_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.maxConnections_ = maxConnections_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.currentConnections_ = currentConnections_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.timestamp_ = timestamp_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tron.p2p.protos.Connect.StatusMessage) {
return mergeFrom((org.tron.p2p.protos.Connect.StatusMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tron.p2p.protos.Connect.StatusMessage other) {
if (other == org.tron.p2p.protos.Connect.StatusMessage.getDefaultInstance()) return this;
if (other.hasFrom()) {
mergeFrom(other.getFrom());
}
if (other.getVersion() != 0) {
setVersion(other.getVersion());
}
if (other.getNetworkId() != 0) {
setNetworkId(other.getNetworkId());
}
if (other.getMaxConnections() != 0) {
setMaxConnections(other.getMaxConnections());
}
if (other.getCurrentConnections() != 0) {
setCurrentConnections(other.getCurrentConnections());
}
if (other.getTimestamp() != 0L) {
setTimestamp(other.getTimestamp());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getFromFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
version_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
networkId_ = input.readInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
maxConnections_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40: {
currentConnections_ = input.readInt32();
bitField0_ |= 0x00000010;
break;
} // case 40
case 48: {
timestamp_ = input.readInt64();
bitField0_ |= 0x00000020;
break;
} // case 48
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private org.tron.p2p.protos.Discover.Endpoint from_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tron.p2p.protos.Discover.Endpoint, org.tron.p2p.protos.Discover.Endpoint.Builder, org.tron.p2p.protos.Discover.EndpointOrBuilder> fromBuilder_;
/**
* .Endpoint from = 1;
* @return Whether the from field is set.
*/
public boolean hasFrom() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .Endpoint from = 1;
* @return The from.
*/
public org.tron.p2p.protos.Discover.Endpoint getFrom() {
if (fromBuilder_ == null) {
return from_ == null ? org.tron.p2p.protos.Discover.Endpoint.getDefaultInstance() : from_;
} else {
return fromBuilder_.getMessage();
}
}
/**
* .Endpoint from = 1;
*/
public Builder setFrom(org.tron.p2p.protos.Discover.Endpoint value) {
if (fromBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
from_ = value;
} else {
fromBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .Endpoint from = 1;
*/
public Builder setFrom(
org.tron.p2p.protos.Discover.Endpoint.Builder builderForValue) {
if (fromBuilder_ == null) {
from_ = builderForValue.build();
} else {
fromBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .Endpoint from = 1;
*/
public Builder mergeFrom(org.tron.p2p.protos.Discover.Endpoint value) {
if (fromBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
from_ != null &&
from_ != org.tron.p2p.protos.Discover.Endpoint.getDefaultInstance()) {
getFromBuilder().mergeFrom(value);
} else {
from_ = value;
}
} else {
fromBuilder_.mergeFrom(value);
}
if (from_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
* .Endpoint from = 1;
*/
public Builder clearFrom() {
bitField0_ = (bitField0_ & ~0x00000001);
from_ = null;
if (fromBuilder_ != null) {
fromBuilder_.dispose();
fromBuilder_ = null;
}
onChanged();
return this;
}
/**
* .Endpoint from = 1;
*/
public org.tron.p2p.protos.Discover.Endpoint.Builder getFromBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getFromFieldBuilder().getBuilder();
}
/**
* .Endpoint from = 1;
*/
public org.tron.p2p.protos.Discover.EndpointOrBuilder getFromOrBuilder() {
if (fromBuilder_ != null) {
return fromBuilder_.getMessageOrBuilder();
} else {
return from_ == null ?
org.tron.p2p.protos.Discover.Endpoint.getDefaultInstance() : from_;
}
}
/**
* .Endpoint from = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tron.p2p.protos.Discover.Endpoint, org.tron.p2p.protos.Discover.Endpoint.Builder, org.tron.p2p.protos.Discover.EndpointOrBuilder>
getFromFieldBuilder() {
if (fromBuilder_ == null) {
fromBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tron.p2p.protos.Discover.Endpoint, org.tron.p2p.protos.Discover.Endpoint.Builder, org.tron.p2p.protos.Discover.EndpointOrBuilder>(
getFrom(),
getParentForChildren(),
isClean());
from_ = null;
}
return fromBuilder_;
}
private int version_ ;
/**
* int32 version = 2;
* @return The version.
*/
@java.lang.Override
public int getVersion() {
return version_;
}
/**
* int32 version = 2;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(int value) {
version_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* int32 version = 2;
* @return This builder for chaining.
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000002);
version_ = 0;
onChanged();
return this;
}
private int networkId_ ;
/**
* int32 network_id = 3;
* @return The networkId.
*/
@java.lang.Override
public int getNetworkId() {
return networkId_;
}
/**
* int32 network_id = 3;
* @param value The networkId to set.
* @return This builder for chaining.
*/
public Builder setNetworkId(int value) {
networkId_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* int32 network_id = 3;
* @return This builder for chaining.
*/
public Builder clearNetworkId() {
bitField0_ = (bitField0_ & ~0x00000004);
networkId_ = 0;
onChanged();
return this;
}
private int maxConnections_ ;
/**
* int32 maxConnections = 4;
* @return The maxConnections.
*/
@java.lang.Override
public int getMaxConnections() {
return maxConnections_;
}
/**
* int32 maxConnections = 4;
* @param value The maxConnections to set.
* @return This builder for chaining.
*/
public Builder setMaxConnections(int value) {
maxConnections_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* int32 maxConnections = 4;
* @return This builder for chaining.
*/
public Builder clearMaxConnections() {
bitField0_ = (bitField0_ & ~0x00000008);
maxConnections_ = 0;
onChanged();
return this;
}
private int currentConnections_ ;
/**
* int32 currentConnections = 5;
* @return The currentConnections.
*/
@java.lang.Override
public int getCurrentConnections() {
return currentConnections_;
}
/**
* int32 currentConnections = 5;
* @param value The currentConnections to set.
* @return This builder for chaining.
*/
public Builder setCurrentConnections(int value) {
currentConnections_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* int32 currentConnections = 5;
* @return This builder for chaining.
*/
public Builder clearCurrentConnections() {
bitField0_ = (bitField0_ & ~0x00000010);
currentConnections_ = 0;
onChanged();
return this;
}
private long timestamp_ ;
/**
* int64 timestamp = 6;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
/**
* int64 timestamp = 6;
* @param value The timestamp to set.
* @return This builder for chaining.
*/
public Builder setTimestamp(long value) {
timestamp_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* int64 timestamp = 6;
* @return This builder for chaining.
*/
public Builder clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00000020);
timestamp_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:StatusMessage)
}
// @@protoc_insertion_point(class_scope:StatusMessage)
private static final org.tron.p2p.protos.Connect.StatusMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tron.p2p.protos.Connect.StatusMessage();
}
public static org.tron.p2p.protos.Connect.StatusMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StatusMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.StatusMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CompressMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:CompressMessage)
com.google.protobuf.MessageOrBuilder {
/**
* .CompressMessage.CompressType type = 1;
* @return The enum numeric value on the wire for type.
*/
int getTypeValue();
/**
* .CompressMessage.CompressType type = 1;
* @return The type.
*/
org.tron.p2p.protos.Connect.CompressMessage.CompressType getType();
/**
* bytes data = 2;
* @return The data.
*/
com.google.protobuf.ByteString getData();
}
/**
* Protobuf type {@code CompressMessage}
*/
public static final class CompressMessage extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:CompressMessage)
CompressMessageOrBuilder {
private static final long serialVersionUID = 0L;
// Use CompressMessage.newBuilder() to construct.
private CompressMessage(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CompressMessage() {
type_ = 0;
data_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CompressMessage();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tron.p2p.protos.Connect.internal_static_CompressMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tron.p2p.protos.Connect.internal_static_CompressMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tron.p2p.protos.Connect.CompressMessage.class, org.tron.p2p.protos.Connect.CompressMessage.Builder.class);
}
/**
* Protobuf enum {@code CompressMessage.CompressType}
*/
public enum CompressType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* uncompress = 0;
*/
uncompress(0),
/**
* snappy = 1;
*/
snappy(1),
UNRECOGNIZED(-1),
;
/**
* uncompress = 0;
*/
public static final int uncompress_VALUE = 0;
/**
* snappy = 1;
*/
public static final int snappy_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CompressType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static CompressType forNumber(int value) {
switch (value) {
case 0: return uncompress;
case 1: return snappy;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
CompressType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public CompressType findValueByNumber(int number) {
return CompressType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.tron.p2p.protos.Connect.CompressMessage.getDescriptor().getEnumTypes().get(0);
}
private static final CompressType[] VALUES = values();
public static CompressType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private CompressType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:CompressMessage.CompressType)
}
public static final int TYPE_FIELD_NUMBER = 1;
private int type_ = 0;
/**
* .CompressMessage.CompressType type = 1;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .CompressMessage.CompressType type = 1;
* @return The type.
*/
@java.lang.Override public org.tron.p2p.protos.Connect.CompressMessage.CompressType getType() {
org.tron.p2p.protos.Connect.CompressMessage.CompressType result = org.tron.p2p.protos.Connect.CompressMessage.CompressType.forNumber(type_);
return result == null ? org.tron.p2p.protos.Connect.CompressMessage.CompressType.UNRECOGNIZED : result;
}
public static final int DATA_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes data = 2;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (type_ != org.tron.p2p.protos.Connect.CompressMessage.CompressType.uncompress.getNumber()) {
output.writeEnum(1, type_);
}
if (!data_.isEmpty()) {
output.writeBytes(2, data_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (type_ != org.tron.p2p.protos.Connect.CompressMessage.CompressType.uncompress.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (!data_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, data_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tron.p2p.protos.Connect.CompressMessage)) {
return super.equals(obj);
}
org.tron.p2p.protos.Connect.CompressMessage other = (org.tron.p2p.protos.Connect.CompressMessage) obj;
if (type_ != other.type_) return false;
if (!getData()
.equals(other.getData())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tron.p2p.protos.Connect.CompressMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.CompressMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.CompressMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.CompressMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.CompressMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.CompressMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.CompressMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.CompressMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.CompressMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.CompressMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.CompressMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.CompressMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tron.p2p.protos.Connect.CompressMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code CompressMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:CompressMessage)
org.tron.p2p.protos.Connect.CompressMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tron.p2p.protos.Connect.internal_static_CompressMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tron.p2p.protos.Connect.internal_static_CompressMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tron.p2p.protos.Connect.CompressMessage.class, org.tron.p2p.protos.Connect.CompressMessage.Builder.class);
}
// Construct using org.tron.p2p.protos.Connect.CompressMessage.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
type_ = 0;
data_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tron.p2p.protos.Connect.internal_static_CompressMessage_descriptor;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.CompressMessage getDefaultInstanceForType() {
return org.tron.p2p.protos.Connect.CompressMessage.getDefaultInstance();
}
@java.lang.Override
public org.tron.p2p.protos.Connect.CompressMessage build() {
org.tron.p2p.protos.Connect.CompressMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.CompressMessage buildPartial() {
org.tron.p2p.protos.Connect.CompressMessage result = new org.tron.p2p.protos.Connect.CompressMessage(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.tron.p2p.protos.Connect.CompressMessage result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.type_ = type_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.data_ = data_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tron.p2p.protos.Connect.CompressMessage) {
return mergeFrom((org.tron.p2p.protos.Connect.CompressMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tron.p2p.protos.Connect.CompressMessage other) {
if (other == org.tron.p2p.protos.Connect.CompressMessage.getDefaultInstance()) return this;
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.getData() != com.google.protobuf.ByteString.EMPTY) {
setData(other.getData());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
type_ = input.readEnum();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
data_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int type_ = 0;
/**
* .CompressMessage.CompressType type = 1;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .CompressMessage.CompressType type = 1;
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .CompressMessage.CompressType type = 1;
* @return The type.
*/
@java.lang.Override
public org.tron.p2p.protos.Connect.CompressMessage.CompressType getType() {
org.tron.p2p.protos.Connect.CompressMessage.CompressType result = org.tron.p2p.protos.Connect.CompressMessage.CompressType.forNumber(type_);
return result == null ? org.tron.p2p.protos.Connect.CompressMessage.CompressType.UNRECOGNIZED : result;
}
/**
* .CompressMessage.CompressType type = 1;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(org.tron.p2p.protos.Connect.CompressMessage.CompressType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
* .CompressMessage.CompressType type = 1;
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes data = 2;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* bytes data = 2;
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
data_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* bytes data = 2;
* @return This builder for chaining.
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000002);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:CompressMessage)
}
// @@protoc_insertion_point(class_scope:CompressMessage)
private static final org.tron.p2p.protos.Connect.CompressMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tron.p2p.protos.Connect.CompressMessage();
}
public static org.tron.p2p.protos.Connect.CompressMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CompressMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.CompressMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface P2pDisconnectMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:P2pDisconnectMessage)
com.google.protobuf.MessageOrBuilder {
/**
* .DisconnectReason reason = 1;
* @return The enum numeric value on the wire for reason.
*/
int getReasonValue();
/**
* .DisconnectReason reason = 1;
* @return The reason.
*/
org.tron.p2p.protos.Connect.DisconnectReason getReason();
}
/**
* Protobuf type {@code P2pDisconnectMessage}
*/
public static final class P2pDisconnectMessage extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:P2pDisconnectMessage)
P2pDisconnectMessageOrBuilder {
private static final long serialVersionUID = 0L;
// Use P2pDisconnectMessage.newBuilder() to construct.
private P2pDisconnectMessage(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private P2pDisconnectMessage() {
reason_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new P2pDisconnectMessage();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tron.p2p.protos.Connect.internal_static_P2pDisconnectMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tron.p2p.protos.Connect.internal_static_P2pDisconnectMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tron.p2p.protos.Connect.P2pDisconnectMessage.class, org.tron.p2p.protos.Connect.P2pDisconnectMessage.Builder.class);
}
public static final int REASON_FIELD_NUMBER = 1;
private int reason_ = 0;
/**
* .DisconnectReason reason = 1;
* @return The enum numeric value on the wire for reason.
*/
@java.lang.Override public int getReasonValue() {
return reason_;
}
/**
* .DisconnectReason reason = 1;
* @return The reason.
*/
@java.lang.Override public org.tron.p2p.protos.Connect.DisconnectReason getReason() {
org.tron.p2p.protos.Connect.DisconnectReason result = org.tron.p2p.protos.Connect.DisconnectReason.forNumber(reason_);
return result == null ? org.tron.p2p.protos.Connect.DisconnectReason.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (reason_ != org.tron.p2p.protos.Connect.DisconnectReason.PEER_QUITING.getNumber()) {
output.writeEnum(1, reason_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (reason_ != org.tron.p2p.protos.Connect.DisconnectReason.PEER_QUITING.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, reason_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tron.p2p.protos.Connect.P2pDisconnectMessage)) {
return super.equals(obj);
}
org.tron.p2p.protos.Connect.P2pDisconnectMessage other = (org.tron.p2p.protos.Connect.P2pDisconnectMessage) obj;
if (reason_ != other.reason_) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + REASON_FIELD_NUMBER;
hash = (53 * hash) + reason_;
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tron.p2p.protos.Connect.P2pDisconnectMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.P2pDisconnectMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.P2pDisconnectMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.P2pDisconnectMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.P2pDisconnectMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tron.p2p.protos.Connect.P2pDisconnectMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.P2pDisconnectMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.P2pDisconnectMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.P2pDisconnectMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.P2pDisconnectMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tron.p2p.protos.Connect.P2pDisconnectMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tron.p2p.protos.Connect.P2pDisconnectMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tron.p2p.protos.Connect.P2pDisconnectMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code P2pDisconnectMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:P2pDisconnectMessage)
org.tron.p2p.protos.Connect.P2pDisconnectMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tron.p2p.protos.Connect.internal_static_P2pDisconnectMessage_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tron.p2p.protos.Connect.internal_static_P2pDisconnectMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tron.p2p.protos.Connect.P2pDisconnectMessage.class, org.tron.p2p.protos.Connect.P2pDisconnectMessage.Builder.class);
}
// Construct using org.tron.p2p.protos.Connect.P2pDisconnectMessage.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
reason_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tron.p2p.protos.Connect.internal_static_P2pDisconnectMessage_descriptor;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.P2pDisconnectMessage getDefaultInstanceForType() {
return org.tron.p2p.protos.Connect.P2pDisconnectMessage.getDefaultInstance();
}
@java.lang.Override
public org.tron.p2p.protos.Connect.P2pDisconnectMessage build() {
org.tron.p2p.protos.Connect.P2pDisconnectMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.P2pDisconnectMessage buildPartial() {
org.tron.p2p.protos.Connect.P2pDisconnectMessage result = new org.tron.p2p.protos.Connect.P2pDisconnectMessage(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.tron.p2p.protos.Connect.P2pDisconnectMessage result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.reason_ = reason_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tron.p2p.protos.Connect.P2pDisconnectMessage) {
return mergeFrom((org.tron.p2p.protos.Connect.P2pDisconnectMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tron.p2p.protos.Connect.P2pDisconnectMessage other) {
if (other == org.tron.p2p.protos.Connect.P2pDisconnectMessage.getDefaultInstance()) return this;
if (other.reason_ != 0) {
setReasonValue(other.getReasonValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
reason_ = input.readEnum();
bitField0_ |= 0x00000001;
break;
} // case 8
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int reason_ = 0;
/**
* .DisconnectReason reason = 1;
* @return The enum numeric value on the wire for reason.
*/
@java.lang.Override public int getReasonValue() {
return reason_;
}
/**
* .DisconnectReason reason = 1;
* @param value The enum numeric value on the wire for reason to set.
* @return This builder for chaining.
*/
public Builder setReasonValue(int value) {
reason_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .DisconnectReason reason = 1;
* @return The reason.
*/
@java.lang.Override
public org.tron.p2p.protos.Connect.DisconnectReason getReason() {
org.tron.p2p.protos.Connect.DisconnectReason result = org.tron.p2p.protos.Connect.DisconnectReason.forNumber(reason_);
return result == null ? org.tron.p2p.protos.Connect.DisconnectReason.UNRECOGNIZED : result;
}
/**
* .DisconnectReason reason = 1;
* @param value The reason to set.
* @return This builder for chaining.
*/
public Builder setReason(org.tron.p2p.protos.Connect.DisconnectReason value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
reason_ = value.getNumber();
onChanged();
return this;
}
/**
* .DisconnectReason reason = 1;
* @return This builder for chaining.
*/
public Builder clearReason() {
bitField0_ = (bitField0_ & ~0x00000001);
reason_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:P2pDisconnectMessage)
}
// @@protoc_insertion_point(class_scope:P2pDisconnectMessage)
private static final org.tron.p2p.protos.Connect.P2pDisconnectMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tron.p2p.protos.Connect.P2pDisconnectMessage();
}
public static org.tron.p2p.protos.Connect.P2pDisconnectMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public P2pDisconnectMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tron.p2p.protos.Connect.P2pDisconnectMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_KeepAliveMessage_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_KeepAliveMessage_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_HelloMessage_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_HelloMessage_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_StatusMessage_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_StatusMessage_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_CompressMessage_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_CompressMessage_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_P2pDisconnectMessage_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_P2pDisconnectMessage_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\rConnect.proto\032\016Discover.proto\"%\n\020KeepA" +
"liveMessage\022\021\n\ttimestamp\030\001 \001(\003\"m\n\014HelloM" +
"essage\022\027\n\004from\030\001 \001(\0132\t.Endpoint\022\022\n\nnetwo" +
"rk_id\030\002 \001(\005\022\014\n\004code\030\003 \001(\005\022\021\n\ttimestamp\030\004" +
" \001(\003\022\017\n\007version\030\005 \001(\005\"\224\001\n\rStatusMessage\022" +
"\027\n\004from\030\001 \001(\0132\t.Endpoint\022\017\n\007version\030\002 \001(" +
"\005\022\022\n\nnetwork_id\030\003 \001(\005\022\026\n\016maxConnections\030" +
"\004 \001(\005\022\032\n\022currentConnections\030\005 \001(\005\022\021\n\ttim" +
"estamp\030\006 \001(\003\"x\n\017CompressMessage\022+\n\004type\030" +
"\001 \001(\0162\035.CompressMessage.CompressType\022\014\n\004" +
"data\030\002 \001(\014\"*\n\014CompressType\022\016\n\nuncompress" +
"\020\000\022\n\n\006snappy\020\001\"9\n\024P2pDisconnectMessage\022!" +
"\n\006reason\030\001 \001(\0162\021.DisconnectReason*\304\002\n\020Di" +
"sconnectReason\022\020\n\014PEER_QUITING\020\000\022\020\n\014BAD_" +
"PROTOCOL\020\001\022\022\n\016TOO_MANY_PEERS\020\002\022\022\n\016DUPLIC" +
"ATE_PEER\020\003\022\025\n\021DIFFERENT_VERSION\020\004\022\026\n\022RAN" +
"DOM_ELIMINATION\020\005\022\021\n\rEMPTY_MESSAGE\020\006\022\020\n\014" +
"PING_TIMEOUT\020\007\022\021\n\rDISCOVER_MODE\020\010\022\023\n\017NO_" +
"SUCH_MESSAGE\020\n\022\017\n\013BAD_MESSAGE\020\013\022\037\n\033TOO_M" +
"ANY_PEERS_WITH_SAME_IP\020\014\022\025\n\021RECENT_DISCO" +
"NNECT\020\r\022\021\n\rDUP_HANDSHAKE\020\016\022\014\n\007UNKNOWN\020\377\001" +
"B\036\n\023org.tron.p2p.protosB\007Connectb\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
org.tron.p2p.protos.Discover.getDescriptor(),
});
internal_static_KeepAliveMessage_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_KeepAliveMessage_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_KeepAliveMessage_descriptor,
new java.lang.String[] { "Timestamp", });
internal_static_HelloMessage_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_HelloMessage_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_HelloMessage_descriptor,
new java.lang.String[] { "From", "NetworkId", "Code", "Timestamp", "Version", });
internal_static_StatusMessage_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_StatusMessage_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_StatusMessage_descriptor,
new java.lang.String[] { "From", "Version", "NetworkId", "MaxConnections", "CurrentConnections", "Timestamp", });
internal_static_CompressMessage_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_CompressMessage_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_CompressMessage_descriptor,
new java.lang.String[] { "Type", "Data", });
internal_static_P2pDisconnectMessage_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_P2pDisconnectMessage_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_P2pDisconnectMessage_descriptor,
new java.lang.String[] { "Reason", });
org.tron.p2p.protos.Discover.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy