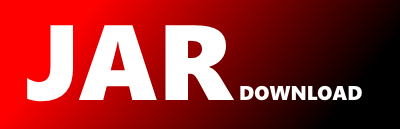
net.chestmc.BoundingBox.kt Maven / Gradle / Ivy
package net.chestmc
import kotlinx.serialization.Serializable
import net.minecraft.server.AxisAlignedBB
import org.bukkit.Location
import org.bukkit.block.Block
import org.bukkit.entity.Entity
/**
* A bounding box represents a hit box used for some things. This is similar to [AxisAlignedBB].
*/
@Serializable
data class BoundingBox(
var minX: Double,
var minY: Double,
var minZ: Double,
var maxX: Double,
var maxY: Double,
var maxZ: Double
) : Cloneable, Comparable {
companion object {
/**
* Converts a string to a bounding box.
*/
fun from(string: String): BoundingBox {
val split = string.split(':', limit = 6)
return BoundingBox(
split[0].toDouble(),
split[1].toDouble(),
split[2].toDouble(),
split[3].toDouble(),
split[4].toDouble(),
split[5].toDouble(),
)
}
}
constructor(axis: AxisAlignedBB) : this(axis.a, axis.b, axis.c, axis.d, axis.e, axis.f)
constructor(min: Location, max: Location) : this(min.x, min.y, min.z, max.x, max.y, max.z)
constructor(min: Block, max: Block) : this(min.location, max.location)
constructor(min: Entity, max: Entity) : this(min.location, max.location)
/**
* Grows this bounding box to the amount of radius.
*/
fun grow(radius: Double): BoundingBox = copy().apply {
minX -= radius
minY -= radius
minZ -= radius
maxX += radius
maxY += radius
maxZ += radius
}
/**
* Shrinks this bounding box to the amount of radius.
*/
fun shrink(radius: Double): BoundingBox = copy().apply {
minX += radius
minY += radius
minZ += radius
maxX -= radius
maxY -= radius
maxZ -= radius
}
/**
* Verifies if a another bounding box is collind with this bounding box.
*/
fun isColliding(other: BoundingBox): Boolean {
return isCollidingX(other) && isCollidingY(other) && isCollidingZ(other)
}
/**
* Verifies if the X of a another bounding box is collind with X of this bounding box.
*/
fun isCollidingX(other: BoundingBox): Boolean = other.maxX > minX && other.minX < maxX
/**
* Verifies if the Y of a another bounding box is collind with Y of this bounding box.
*/
fun isCollidingY(other: BoundingBox): Boolean = other.maxY > minY && other.minY < maxY
/**
* Verifies if the Z of a another bounding box is collind with Z of this bounding box.
*/
fun isCollidingZ(other: BoundingBox): Boolean = other.maxZ > minZ && other.minZ < maxZ
/**
* Verifies if a location is inside of this bounding box.
*/
fun isInside(loc: Location): Boolean {
return (minX..maxX).contains(loc.x) && (minY..maxY).contains(loc.y) && (minZ..maxZ).contains(loc.z)
}
/**
* Verifies if a block is inside of this bounding box.
*/
fun isInside(block: Block): Boolean = isInside(block.location)
/**
* Verifies if a entity is inside of this bounding box.
*/
fun isInside(entity: Entity): Boolean = isInside(entity.location)
/**
* Converts this bounding box to a [AxisAlignedBB].
*/
fun toAxis(): AxisAlignedBB = AxisAlignedBB(minX, minY, minZ, maxX, maxY, maxZ)
override fun compareTo(other: BoundingBox): Int =
minX.compareTo(other.minX) +
minY.compareTo(other.minY) +
minZ.compareTo(other.minZ) +
maxX.compareTo(other.maxX) +
maxY.compareTo(other.maxY) +
maxZ.compareTo(other.maxZ)
override fun toString(): String = "$minX:$minY:$minZ:$maxX:$maxY:$maxZ"
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy