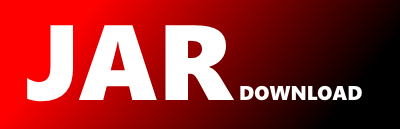
net.chestmc.block.IBlockController.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chest-server Show documentation
Show all versions of chest-server Show documentation
A spigot fork to kotlin structure and news.
The newest version!
package net.chestmc.block
import net.minecraft.server.NBTTagCompound
import org.bukkit.Location
import org.bukkit.World
import org.bukkit.block.Block
import org.bukkit.block.BlockFace
import org.bukkit.entity.Entity
import org.bukkit.entity.Player
import org.bukkit.inventory.ItemStack
import java.util.*
/**
* Represents a controller for custom blocks. This allows changing the interact actions,
* breaks events, places and much more.
*/
interface IBlockController {
/**
* Represents the block that this controller is controlling.
*/
val block: Block
/**
* Represents the location of the block that this controller is controlling.
*/
val location: Location
/**
* Represents the world of the block that this controller is controlling.
*/
val world: World
/**
* Initializes all necessary (or optional) action of this block controller.
* This is called when this controller starts controlling a block.
*/
fun startup()
/**
* Shutdown all necessary (or optional) action of this block controller.
* This is called when this controller is not more controlling a block.
*/
fun shutdown()
/**
* When a player attack with this block, this will be triggered.
* In other words, this will be triggered when a player left clicks this block.
*
* If you need when right click, uses [onInteract].
*
* If this function returns false, the block will not clicked.
*/
fun onAttack(player: Player, face: BlockFace): Boolean
/**
* When a player interact with this block, this will be triggered.
* In other words, this will be triggered when a player right clicks this block.
*
* If you need when left click, uses [onAttack].
*
* If this function returns false, the block will not clicked.
*/
fun onInteract(player: Player, face: BlockFace): Boolean
/**
* When a player breaks this block, this will be triggered.
* If this function returns false, the block will not breaked.
*/
fun onBreak(player: Player): Boolean
/**
* When a player place this block, this will be triggered.
* If this function returns false, the block will not placed.
*/
fun onPlace(player: Player, item: ItemStack, face: BlockFace): Boolean
/**
* Returns if this block can tick.
*/
fun canTick(): Boolean
/**
* When this block tick, this will be triggered.
* If the function [canTick] returns false, this block will **not** tick.
*/
fun onTick(random: Random)
/**
* When a entity collides with this block, this will be triggered.
*/
fun onCollide(entity: Entity)
/**
* When a entity jumps in this block, this will be triggered.
* If this function returns false, the entity cannot jump at this block.
*/
fun onJump(entity: Entity): Boolean
/**
* When a entity post jumps in this block, this will be triggered.
* This runs **after** [onJump]. And can be used to set the jump height.
*/
fun onPostJump(entity: Entity)
/**
* When a entity falls in this block, this will be triggered.
*/
fun onFall(entity: Entity, height: Float)
/**
* When a entity is walking above this block, this will be triggered.
*/
fun onStep(entity: Entity)
/**
* Executes a physics action of this block.
*/
fun doPhysics()
/**
* Returns if this block will persist your data on breaking.
*
* Note: the [beforeBreak] is a check mark used to know if this function is calling
* before the function [onBreak] or not. If you want for example, cancel the [onBreak], but
* persist the data, you can use:
*
* ```kt
* fun onBreak(player: Player): Boolean {
* block.type = Material.AIR
* return false
* }
*
* fun persistOnBreak(beforeBreak: Boolean): Boolean = true
* ```
* By default this is false for both parts.
*/
fun persistOnBreak(beforeBreak: Boolean): Boolean
/**
* Returns the item stack used to place this block.
* This is commonly used when you need to store data of this block in a item.
* By default this just returns a item of the block type
* with a persistent tag declaring the class of the controller.
*/
fun getItem(): ItemStack
/**
* Saves this block in the gived tag.
* This will persist across server restarts.
*/
fun save(tag: NBTTagCompound)
/**
* Loads this block from the specified tag.
*/
fun load(tag: NBTTagCompound)
/**
* Sets this controller to the specified block.
*/
fun setAt(block: Block) {
block.controller = this
}
/**
* Sets this controller to the block in the specified location.
*/
fun setAt(location: Location) {
location.block.controller = this
}
}
/**
* Returns the controller key used to get the class of a controller.
*/
const val CONTROLLER_KEY = "BlockControllerClass"
© 2015 - 2025 Weber Informatics LLC | Privacy Policy