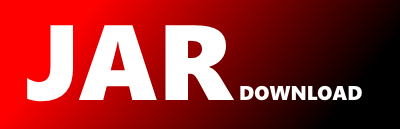
net.chestmc.common.Plugin.kt Maven / Gradle / Ivy
package net.chestmc.common
import io.github.uinnn.serializer.DefaultJsonStrategyFormat
import kotlinx.coroutines.CoroutineScope
import kotlinx.serialization.decodeFromString
import net.chestmc.common.extensions.cast
import net.chestmc.common.extensions.collections.emptyMutableList
import net.chestmc.common.metadata.Metadata
import net.chestmc.common.metadata.Metadatable
import net.chestmc.common.service.ServiceRegistry
import org.bukkit.event.HandlerList
import org.bukkit.plugin.Plugin
import org.bukkit.plugin.java.JavaPlugin
import kotlin.coroutines.CoroutineContext
import kotlin.coroutines.EmptyCoroutineContext
import kotlin.reflect.full.createInstance
/**
* A [Plugin] is a extended [JavaPlugin]. With [Metadatable] and [CoroutineScope] implemented.
*/
abstract class Plugin : JavaPlugin(), Metadatable, CoroutineScope, Comparable {
override val coroutineContext: CoroutineContext = EmptyCoroutineContext
override var metadata: Metadata = Metadata.builder().variableExpiration().build()
val services: MutableList = emptyMutableList()
/**
* Execution when this plugin is loading.
*/
open fun load() {}
/**
* Execution when this plugin starts.
*/
open fun startup() {}
/**
* Execution when this plugin disables.
*/
open fun shutdown() {}
/**
* Startups all services processed by the service processor.
*/
fun startupServices() {
val raw = getRawServices()
if (raw.isNullOrEmpty())
return
services.clear()
services.addAll(
raw.map {
val clazz = Class.forName(it, true, classLoader).kotlin
(clazz.objectInstance ?: clazz.createInstance()).cast()
}
)
services.forEach(ServiceRegistry::startup)
}
/**
* Shutdowns all services processed by the service processor.
*/
fun shutdownServices() = services.forEach(ServiceRegistry::shutdown)
/**
* Gets a raw list of all registered services processed by the service processor.
*/
fun getRawServices(): List {
val raw = getResource("services.json")?.reader()?.buffered().use { it?.readText() }
return if (raw == null) emptyList() else DefaultJsonStrategyFormat.decodeFromString(raw)
}
override fun compareTo(other: Plugin): Int = description.version.compareTo(other.description.version)
override fun onLoad() = load()
override fun onEnable() {
startupServices()
startup()
}
override fun onDisable() {
HandlerList.unregisterAll(this)
shutdownServices()
shutdown()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy