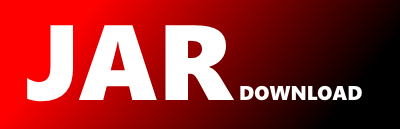
net.chestmc.common.extensions.Entities.kt Maven / Gradle / Ivy
package net.chestmc.common.extensions
import com.gmail.filoghost.holographicdisplays.api.HologramsAPI
import net.chestmc.BoundingBox
import net.chestmc.common.MinecraftEntity
import net.minecraft.server.NBTTagCompound
import org.bukkit.craftbukkit.entity.CraftEntity
import org.bukkit.entity.Ageable
import org.bukkit.entity.Entity
import org.bukkit.entity.Zombie
typealias TagTransformer = NBTTagCompound.() -> Unit
/**
* Get the craft handler of this entity.
*/
inline val Entity.craftHandler get() = this as CraftEntity
/**
* Get the minecraft handler of this entity.
*/
inline val Entity.handler: MinecraftEntity get() = craftHandler.handle
/**
* Gets the bounding box of this entity.
*/
inline val Entity.boundingBox get() = BoundingBox(handler.boundingBox)
/**
* Transforms this entity tag by the specified transform.
*/
inline fun Entity.applyTag(transform: TagTransformer) = transform {
tag = tag.apply(transform)
}
/**
* Returns if this entity is silent or not.
*/
inline var Entity.isSilent: Boolean
get() = tag.getBoolean("Silent")
set(value) {
applyTag {
setBoolean("Silent", value)
}
}
/**
* Returns if this entity is persistent or not.
*/
inline var Entity.isPersistent: Boolean
get() = tag.getBoolean("PersistenceRequired")
set(value) {
applyTag {
setBoolean("PersistenceRequired", value)
}
}
/**
* Returns if this entity is invulnerable or not.
*/
inline var Entity.isInvulnerable: Boolean
get() = tag.getBoolean("Invulnerable")
set(value) {
applyTag {
setBoolean("Invulnerable", value)
}
}
/**
* Returns if this entity has artificial intelligence or not.
*/
inline var Entity.hasAI: Boolean
get() = !tag.getBoolean("NoAI")
set(value) {
applyTag {
setBoolean("NoAI", !value)
}
}
/**
* Returns if this entity is baby or not.
*/
var Entity.isBaby: Boolean
get() = when (this) {
is Ageable -> !isAdult
is Zombie -> isBaby
else -> false
}
set(value) = when (this) {
is Ageable -> if (value) setBaby() else setAdult()
is Zombie -> isBaby = value
else -> Unit
}
/**
* Returns if this entity is adult or not.
*/
inline var Entity.isAdult: Boolean
get() = !isBaby
set(value) {
isBaby = !value
}
/**
* Returns if this entity is a hologram.
*/
inline val Entity.isHologram get() = HologramsAPI.isHologramEntity(this)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy