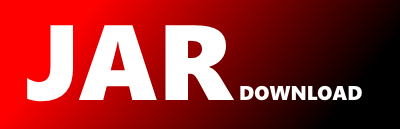
net.chestmc.common.extensions.Items.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chest-server Show documentation
Show all versions of chest-server Show documentation
A spigot fork to kotlin structure and news.
The newest version!
package net.chestmc.common.extensions
import net.chestmc.common.MinecraftItem
import net.chestmc.common.SkullCreator
import net.chestmc.common.extensions.collections.emptyMutableList
import net.chestmc.common.extensions.collections.process
import net.chestmc.common.extensions.strings.process
import net.chestmc.common.nbt.ItemTag
import net.chestmc.common.placeholder.Placeholder
import net.chestmc.common.placeholder.process
import org.bukkit.Material
import org.bukkit.craftbukkit.inventory.CraftItemStack
import org.bukkit.enchantments.Enchantment
import org.bukkit.inventory.ItemFlag
import org.bukkit.inventory.ItemStack
import org.bukkit.inventory.meta.ItemMeta
import org.bukkit.material.MaterialData
import java.util.*
typealias MetaTransformer = ItemMeta.() -> Unit
/**
* Transforms this item stack meta by the specified transform.
*/
inline fun ItemStack.applyMeta(transform: MetaTransformer) = transform {
val meta = itemMeta
meta.apply(transform)
itemMeta = meta
}
/**
* Returns the display name of this item stack.
*/
var ItemStack.name: String
get() = itemMeta.displayName ?: type.name
set(value) {
applyMeta {
displayName = value
}
}
/**
* Sets the specified name to this item stack.
*/
fun ItemStack.name(name: String) = applyMeta {
displayName = name
}
/**
* Returns the lore of this item stack.
*/
var ItemStack.lore: MutableList
get() = itemMeta.lore ?: emptyMutableList()
set(value) {
applyMeta {
lore = value
}
}
/**
* Inserts the specified lore to this item stack.
*/
fun ItemStack.lore(lines: Iterable) = applyMeta {
lore = lines.toList()
}
/**
* Inserts the specified lore to this item stack.
*/
fun ItemStack.lore(vararg lines: String) = applyMeta {
lore = lines.toList()
}
/**
* Inserts the specified multiline lore to this item stack. Like:
* ```
* lore("""
* §7First line
* §7Second line
* """)
* ```
*/
fun ItemStack.lore(lines: String) = applyMeta {
lore = lines.lines()
}
/**
* Returns the flags of this item stack.
*/
var ItemStack.flags: MutableSet
get() = itemMeta.itemFlags ?: mutableSetOf()
set(value) {
applyMeta {
addItemFlags(*value.toTypedArray())
}
}
/**
* Inserts the specified flags to this item stack.
*/
fun ItemStack.flags(vararg flags: ItemFlag) = applyMeta {
addItemFlags(*flags)
}
/**
* Inserts the specified enchantments to this item stack.
*/
fun ItemStack.enchantments(vararg enchantments: Pair) = transform {
addUnsafeEnchantments(enchantments.toMap())
}
/**
* Sets the specified amount of this item stack.
*/
fun ItemStack.amount(amount: Int) = transform {
this.amount = amount
}
/**
* Sets the specified durability of this item stack.
*/
fun ItemStack.durability(durability: Int) = transform {
this.durability = durability.toShort()
}
/**
* Sets the specified material data of this item stack.
*/
fun ItemStack.data(materialData: MaterialData) = transform {
this.data = materialData
}
/**
* Sets the specified material data of this item stack.
*/
fun ItemStack.data(type: Material, data: Number) = transform {
this.data = newData(type, data)
}
/**
* Sets the specified type of this item stack.
*/
fun ItemStack.type(type: Material) = transform {
this.type = type
}
/**
* Converts this item to a [MinecraftItem].
*/
fun ItemStack.asMinecraftItem(): MinecraftItem = CraftItemStack.asNMSCopy(this)
/**
* Converts this item to a [CraftItemStack].
*/
fun ItemStack.asCraftItem(): CraftItemStack = CraftItemStack.asCraftCopy(this)
/**
* Transforms this item stack tag by the specified transform.
*/
inline fun ItemStack.applyTag(transform: TagTransformer) = transform {
ItemTag(this).apply(transform).inject()
}
/**
* Returns if this item stack is unbreakable or not.
*/
inline var ItemStack.isUnbreakable: Boolean
get() = tag.getBoolean("Unbreakable")
set(value) {
applyTag {
setBoolean("Unbreakable", value)
}
}
/**
* Creates a copy of this item stack and returns them.
*/
fun ItemStack.copy(): ItemStack = clone()
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore.
*/
fun ItemStack.process(model: ItemStack, placeholder: Placeholder, value: T) = transform {
name(placeholder.process(model.name, value))
lore(placeholder.process(model.lore, value))
}
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore.
*/
fun ItemStack.process(model: ItemStack, value: Pair) = transform {
name(model.name.process(value))
lore(model.lore.process(value))
}
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore.
*/
fun ItemStack.process(model: ItemStack, vararg value: Pair) = transform {
name(model.name.process(*value))
lore(model.lore.process(*value))
}
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore.
*/
fun ItemStack.process(model: ItemStack, value: Map) = transform {
name(model.name.process(value))
lore(model.lore.process(value))
}
/**
* Process this item stack with the specified placeholder and value.
*/
fun ItemStack.process(placeholder: Placeholder, value: T) = transform {
name(placeholder.process(name, value))
lore(placeholder.process(lore, value))
}
/**
* Process only specified placeholder in this item stack.
*/
fun ItemStack.process(value: Pair) = transform {
name(name.process(value))
lore(lore.process(value))
}
/**
* Process all specifieds placeholders in this item stack.
*/
fun ItemStack.process(vararg values: Pair) = transform {
name(name.process(*values))
lore(lore.process(*values))
}
/**
* Process all specifieds placeholders in this item stack.
*/
fun ItemStack.process(values: Map) = transform {
name(name.process(values))
lore(lore.process(values))
}
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore and returns a copy of them.
*/
fun ItemStack.processCopy(model: ItemStack, placeholder: Placeholder, value: T): ItemStack =
copy().process(model, placeholder, value)
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore and returns a copy of them.
*/
fun ItemStack.processCopy(model: ItemStack, value: Pair): ItemStack =
copy().process(model, value)
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore and returns a copy of them.
*/
fun ItemStack.processCopy(model: ItemStack, vararg value: Pair): ItemStack =
copy().process(model, *value)
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore and returns a copy of them.
*/
fun ItemStack.processCopy(model: ItemStack, value: Map): ItemStack =
copy().process(model, value)
/**
* Process this item stack with the specified placeholder and value and returns a copy of them.
*/
fun ItemStack.processCopy(placeholder: Placeholder, value: T): ItemStack =
copy().process(placeholder, value)
/**
* Process only specified placeholder in this item stack and returns a copy of them.
*/
fun ItemStack.processCopy(value: Pair): ItemStack = copy().process(value)
/**
* Process all specifieds placeholders in this item stack and returns a copy of them.
*/
fun ItemStack.processCopy(vararg values: Pair): ItemStack = copy().process(*values)
/**
* Process all specifieds placeholders in this item stack and returns a copy of them.
*/
fun ItemStack.processCopy(values: Map): ItemStack = copy().process(values)
/**
* Creates a new empty item stack.
*/
fun emptyItem(): ItemStack = ItemStack(Material.AIR, 0)
/**
* Creates a new item stack by the specified material.
*/
fun newItem(material: Material, amount: Int = 1): ItemStack = ItemStack(material, amount)
/**
* Creates a new item stack by the specified material data.
*/
fun newItem(material: MaterialData, amount: Int = 1): ItemStack = material.toItemStack(amount)
/**
* Creates a new item stack by the specified material and name.
*/
fun newItem(material: Material, name: String, amount: Int = 1): ItemStack =
ItemStack(material, amount).name(name)
/**
* Creates a new item stack by the specified material and lore.
*/
fun newItem(material: Material, lore: List, amount: Int = 1): ItemStack =
ItemStack(material, amount).lore(lore)
/**
* Creates a new item stack by the specified material, name and lore.
*/
fun newItem(material: Material, name: String, lore: List, amount: Int = 1): ItemStack =
ItemStack(material, amount).name(name).lore(lore)
/**
* Creates a new item stack by the specified material data and name.
*/
fun newItem(material: MaterialData, name: String, amount: Int = 1): ItemStack =
material.toItemStack(amount).name(name)
/**
* Creates a new item stack by the specified material data and lore.
*/
fun newItem(material: MaterialData, lore: List, amount: Int = 1): ItemStack =
material.toItemStack(amount).lore(lore)
/**
* Creates a new item stack by the specified material data, name and lore.
*/
fun newItem(material: MaterialData, name: String, lore: List, amount: Int = 1): ItemStack =
material.toItemStack(amount).name(name).lore(lore)
/**
* Creates a new item stack head by the specified player id.
*/
fun newItem(owner: UUID, amount: Int = 1): ItemStack = SkullCreator.fromUUID(owner).amount(amount)
/**
* Creates a new item stack head by the specified player id and lore.
*/
fun newItem(owner: UUID, lore: List, amount: Int = 1): ItemStack =
SkullCreator.fromUUID(owner).amount(amount).lore(lore)
/**
* Creates a new item stack head by the specified player id and name.
*/
fun newItem(owner: UUID, name: String, amount: Int = 1): ItemStack =
SkullCreator.fromUUID(owner).amount(amount).name(name)
/**
* Creates a new item stack head by the specified player id, name and lore.
*/
fun newItem(owner: UUID, name: String, lore: List, amount: Int = 1): ItemStack =
SkullCreator.fromUUID(owner).amount(amount).name(name).lore(lore)
/**
* Creates a new item stack head by the specified url head.
*/
fun newItem(base: String, amount: Int = 1): ItemStack = SkullCreator.fromBase64(base).amount(amount)
/**
* Creates a new item stack head by the specified url head and lore.
*/
fun newItem(base: String, lore: List, amount: Int = 1): ItemStack =
SkullCreator.fromBase64(base).amount(amount).lore(lore)
/**
* Creates a new item stack head by the specified url head and name.
*/
fun newItem(base: String, name: String, amount: Int = 1): ItemStack =
SkullCreator.fromBase64(base).amount(amount).name(name)
/**
* Creates a new item stack head by the specified url head, name and lore.
*/
fun newItem(base: String, name: String, lore: List, amount: Int = 1): ItemStack =
SkullCreator.fromBase64(base).amount(amount).name(name).lore(lore)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy