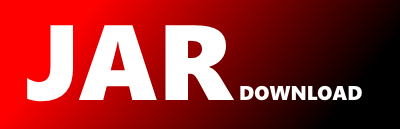
net.chestmc.common.extensions.Locations.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chest-server Show documentation
Show all versions of chest-server Show documentation
A spigot fork to kotlin structure and news.
The newest version!
package net.chestmc.common.extensions
import com.intellectualcrafters.plot.`object`.Plot
import net.chestmc.common.PlotLocation
import net.chestmc.common.extensions.constants.LOCATION_REPOSITORY_FOLDER
import org.bukkit.Location
import org.bukkit.block.BlockFace
import org.bukkit.entity.Entity
import kotlin.math.cos
import kotlin.math.sin
/**
* Gets the repository file of this location. Note that this not creates the repository if not exists.
*/
inline val Location.repository get() = file(LOCATION_REPOSITORY_FOLDER, "${asText()}.repository")
/**
* Spawns the specified entity in generic type with this location and returns the entity.
*/
inline fun Location.spawn(): T = world.spawn(this, T::class.java)
/**
* Converts this location to a plain string text.
*/
fun Location.asText(): String = "${world.name}|$blockX|$blockY|$blockZ"
/**
* Converts this location to a plot location.
*/
fun Location.toPlotLocation(): PlotLocation = PlotLocation(world.name, blockX, blockY, blockZ)
/**
* Gets the plot of this location or null.
*/
fun Location.plotOrNull(): Plot? = toPlotLocation().plot
/**
* Converts this plot location to a bukkit location.
*/
fun PlotLocation.toLocation(): Location = localization(world, x, y, z)
/**
* Returns all nearby entities with this location as central.
*/
fun Location.nearbyEntities(x: Number, y: Number, z: Number): List =
world.getNearbyEntities(this, x.toDouble(), y.toDouble(), z.toDouble()).toList()
/**
* Returns a list of locations demarcating a circle, with this location as central.
*/
fun Location.getCircle(radius: Double, amount: Int): List = buildList {
val increment = (2 * Math.PI) / amount
for (i in 0 until amount) {
val angle = i * increment
val x = x + radius * cos(angle)
val z = z + radius * sin(angle)
add(Location(world, x, y, z))
}
}
/**
* Returns the relative location of this location by the specified coordinates.
*/
fun Location.getRelative(x: Int, y: Int, z: Int) = Location(world, this.x + x, this.y + y, this.z + z)
/**
* Returns the relative location of this location by the specified face and distance.
*/
fun Location.getRelative(face: BlockFace, distance: Int = 1) =
getRelative(face.modX * distance, face.modY * distance, face.modZ * distance)
/**
* Gets the up relative location of this location.
*/
fun Location.up(distance: Int = 1) = getRelative(0, distance, 0)
/**
* Gets the down relative location of this location.
*/
fun Location.down(distance: Int = 1) = getRelative(0, -distance, 0)
/**
* Gets the south relative location of this location.
*/
fun Location.south(distance: Int = 1) = getRelative(BlockFace.SOUTH, distance)
/**
* Gets the north relative location of this location.
*/
fun Location.north(distance: Int = 1) = getRelative(BlockFace.NORTH, distance)
/**
* Gets the east relative location of this location.
*/
fun Location.east(distance: Int = 1) = getRelative(BlockFace.EAST, distance)
/**
* Gets the west relative location of this location.
*/
fun Location.west(distance: Int = 1) = getRelative(BlockFace.WEST, distance)
/**
* Gets the south west relative location of this location.
*/
fun Location.southwest(distance: Int = 1) = getRelative(BlockFace.SOUTH_WEST, distance)
/**
* Gets the north west relative location of this location.
*/
fun Location.northwest(distance: Int = 1) = getRelative(BlockFace.NORTH_WEST, distance)
/**
* Gets the south east relative location of this location.
*/
fun Location.southeast(distance: Int = 1) = getRelative(BlockFace.SOUTH_EAST, distance)
/**
* Gets the north east relative location of this location.
*/
fun Location.northeast(distance: Int = 1) = getRelative(BlockFace.NORTH_EAST, distance)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy