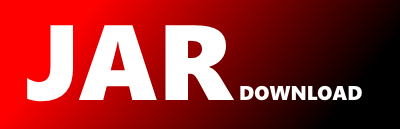
net.chestmc.common.extensions.Players.kt Maven / Gradle / Ivy
package net.chestmc.common.extensions
import com.intellectualcrafters.plot.`object`.Plot
import com.intellectualcrafters.plot.`object`.PlotPlayer
import net.chestmc.common.common.Notification
import net.chestmc.common.extensions.constants.ECONOMY
import net.chestmc.common.extensions.constants.PLAYER_REPOSITORY_FOLDER
import net.chestmc.common.extensions.constants.SUBTITLE
import net.chestmc.common.extensions.constants.TITLE
import net.md_5.bungee.api.chat.TextComponent
import net.minecraft.server.*
import org.bukkit.OfflinePlayer
import org.bukkit.block.Block
import org.bukkit.craftbukkit.entity.CraftPlayer
import org.bukkit.entity.Player
import org.bukkit.inventory.ItemStack
import org.bukkit.util.BlockIterator
import java.util.*
/**
* Gets the craft handler of this player.
*/
inline val Player.craftHandler get() = this as CraftPlayer
/**
* Gets the minecraft handler of this player.
*/
inline val Player.handler: EntityPlayer get() = craftHandler.handle
/**
* Shortcut for getting the UUID of this player.
*/
inline val OfflinePlayer.id: UUID get() = uniqueId
/**
* Returns the ip of this player.
*/
inline val Player.ip: String get() = address.hostName
/**
* Returns the head of this player.
*/
inline val OfflinePlayer.head: ItemStack get() = newItem(uniqueId)
/**
* Gets the repository file of this player. Note that this not creates the repository if not exists.
*/
inline val OfflinePlayer.repository get() = file(PLAYER_REPOSITORY_FOLDER, "${id}.repository")
/**
* Returns all plots of this player.
*/
inline val Player.plots: Set get() = toPlotPlayer().plots
/**
* Gets the vault balance of this player
*/
inline var Player.balance: Double
get() = ECONOMY.getBalance(this)
set(value) {
ECONOMY.withdrawPlayer(this, balance)
ECONOMY.depositPlayer(this, value)
}
/**
* Makes the player says a message in the chat.
*/
fun Player.say(message: String) = chat(message)
/**
* Gives a item to the player inventory.
*/
fun Player.giveItem(item: ItemStack): Map = inventory.addItem(item)
/**
* Converts this player to a plot player.
*/
fun Player.toPlotPlayer(): PlotPlayer = PlotPlayer.wrap(this)
/**
* Sends a packet to this player.
*/
fun Player.sendPacket(packet: Packet) = transform {
handler.playerConnection.sendPacket(packet)
}
/**
* Sends a action bar to this player.
*/
fun Player.action(message: Any) =
sendPacket(PacketPlayOutChat(IChatBaseComponent.ChatSerializer.a("{\"text\":\"$message\"}"), 2.toByte()))
/**
* Sends a title to this player.
*/
fun Player.title(
title: String = "",
subtitle: String = "",
fadeIn: Int = 20,
stay: Int = 40,
fadeOut: Int = 20
) = transform {
sendPacket(PacketPlayOutTitle(TITLE, IChatBaseComponent.ChatSerializer.a("{\"text\":\"$title\"}")))
sendPacket(PacketPlayOutTitle(SUBTITLE, IChatBaseComponent.ChatSerializer.a("{\"text\":\"$subtitle\"}")))
sendPacket(PacketPlayOutTitle(fadeIn, stay, fadeOut))
}
/**
* Logs a notification to this player.
*/
fun Player.log(notification: Notification) = transform {
notification.log(this)
}
/**
* Logs a text component to this player.
*/
fun Player.log(component: TextComponent) = transform {
spigot().sendMessage(component)
}
/**
* Gets the target block of this player. This not classifies AIR blocks as target.
* @see getTargetBlockAir
*/
fun Player.getTargetBlock(range: Int): Block? {
val iter = BlockIterator(this, range)
var lastBlock = iter.next()
while (iter.hasNext()) {
lastBlock = iter.next()
if (lastBlock.type === Material.AIR) continue
break
}
return lastBlock
}
/**
* Gets the target block of this player. This classifies AIR blocks as target.
*/
fun Player.getTargetBlockAir(range: Int): Block = BlockIterator(this, range).next()
© 2015 - 2025 Weber Informatics LLC | Privacy Policy